Download this notebook from github.
Xsarsea example
The Normalized Radar Cross Section (sigma0) as computed from Level-1 SAR data can be detrended in the case of ocean scenes.
The goal is to remove the averaged trend (decreasing) of the NRCS with (increasing) incidence angle observed for acquisitions over ocean.
The detrend maximizes the contrasts in the image due to geophysical phenomena and improves the visualization experience of ocean scenes.
Sigma0_detrend is also termed image roughness or nice display.
[1]:
import xsar
import xsarsea
[2]:
# use holoviews for plots
import bokeh.io
bokeh.io.output_notebook()
import holoviews as hv
hv.extension('bokeh')
from holoviews.operation.datashader import datashade,rasterize
[3]:
# optional debug message
import logging
logging.basicConfig()
logging.getLogger('xsarsea').setLevel(logging.INFO) # .setLevel(logging.DEBUG) for more messages
read the dataset with xsar
[4]:
# get test file. You can replace with an path to other SAFE
filename = xsar.get_test_file('S1A_IW_GRDH_1SDV_20170907T103020_20170907T103045_018268_01EB76_Z010.SAFE')
filename
[4]:
'/tmp/S1A_IW_GRDH_1SDV_20170907T103020_20170907T103045_018268_01EB76_Z010.SAFE'
[5]:
# open the dataset with xsar
sar_ds = xsar.open_dataset(filename, resolution='1000m')
sar_ds[['longitude','latitude','sigma0','incidence']]
[5]:
<xarray.Dataset> Dimensions: (line: 167, sample: 251, pol: 2) Coordinates: * pol (pol) object 'VV' 'VH' * line (line) float64 49.5 149.5 249.5 ... 1.655e+04 1.665e+04 * sample (sample) float64 49.5 149.5 249.5 ... 2.495e+04 2.505e+04 spatial_ref int64 0 Data variables: longitude (line, sample) float64 dask.array<chunksize=(167, 251), meta=np.ndarray> latitude (line, sample) float64 dask.array<chunksize=(167, 251), meta=np.ndarray> sigma0 (pol, line, sample) float64 dask.array<chunksize=(1, 167, 251), meta=np.ndarray> incidence (line, sample) float64 dask.array<chunksize=(167, 251), meta=np.ndarray> Attributes: (12/15) name: SENTINEL1_DS:/tmp/S1A_IW_GRDH_1SDV_20170907T103020_201... short_name: SENTINEL1_DS:S1A_IW_GRDH_1SDV_20170907T103020_20170907... product: GRDH safe: S1A_IW_GRDH_1SDV_20170907T103020_20170907T103045_01826... swath: IW multidataset: False ... ... start_date: 2017-09-07 10:30:20.936409 stop_date: 2017-09-07 10:30:45.935264 footprint: POLYGON ((-67.84221143971432 20.72564283093837, -70.22... coverage: 170km * 251km (line * sample ) orbit_pass: Descending platform_heading: -167.7668824808032
- line: 167
- sample: 251
- pol: 2
- pol(pol)object'VV' 'VH'
- comment :
- ordered polarizations (copol, crosspol)
array(['VV', 'VH'], dtype=object)
- line(line)float6449.5 149.5 ... 1.655e+04 1.665e+04
- units :
- 1
- comment :
- azimuth direction, in pixels from full resolution tiff
- axis :
- Y
array([ 49.5, 149.5, 249.5, 349.5, 449.5, 549.5, 649.5, 749.5, 849.5, 949.5, 1049.5, 1149.5, 1249.5, 1349.5, 1449.5, 1549.5, 1649.5, 1749.5, 1849.5, 1949.5, 2049.5, 2149.5, 2249.5, 2349.5, 2449.5, 2549.5, 2649.5, 2749.5, 2849.5, 2949.5, 3049.5, 3149.5, 3249.5, 3349.5, 3449.5, 3549.5, 3649.5, 3749.5, 3849.5, 3949.5, 4049.5, 4149.5, 4249.5, 4349.5, 4449.5, 4549.5, 4649.5, 4749.5, 4849.5, 4949.5, 5049.5, 5149.5, 5249.5, 5349.5, 5449.5, 5549.5, 5649.5, 5749.5, 5849.5, 5949.5, 6049.5, 6149.5, 6249.5, 6349.5, 6449.5, 6549.5, 6649.5, 6749.5, 6849.5, 6949.5, 7049.5, 7149.5, 7249.5, 7349.5, 7449.5, 7549.5, 7649.5, 7749.5, 7849.5, 7949.5, 8049.5, 8149.5, 8249.5, 8349.5, 8449.5, 8549.5, 8649.5, 8749.5, 8849.5, 8949.5, 9049.5, 9149.5, 9249.5, 9349.5, 9449.5, 9549.5, 9649.5, 9749.5, 9849.5, 9949.5, 10049.5, 10149.5, 10249.5, 10349.5, 10449.5, 10549.5, 10649.5, 10749.5, 10849.5, 10949.5, 11049.5, 11149.5, 11249.5, 11349.5, 11449.5, 11549.5, 11649.5, 11749.5, 11849.5, 11949.5, 12049.5, 12149.5, 12249.5, 12349.5, 12449.5, 12549.5, 12649.5, 12749.5, 12849.5, 12949.5, 13049.5, 13149.5, 13249.5, 13349.5, 13449.5, 13549.5, 13649.5, 13749.5, 13849.5, 13949.5, 14049.5, 14149.5, 14249.5, 14349.5, 14449.5, 14549.5, 14649.5, 14749.5, 14849.5, 14949.5, 15049.5, 15149.5, 15249.5, 15349.5, 15449.5, 15549.5, 15649.5, 15749.5, 15849.5, 15949.5, 16049.5, 16149.5, 16249.5, 16349.5, 16449.5, 16549.5, 16649.5])
- sample(sample)float6449.5 149.5 ... 2.495e+04 2.505e+04
- units :
- 1
- comment :
- cross track direction, in pixels from full resolution tiff
- axis :
- X
array([ 49.5, 149.5, 249.5, ..., 24849.5, 24949.5, 25049.5])
- spatial_ref()int640
- crs_wkt :
- GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AXIS["Latitude",NORTH],AXIS["Longitude",EAST],AUTHORITY["EPSG","4326"]]
- semi_major_axis :
- 6378137.0
- semi_minor_axis :
- 6356752.314245179
- inverse_flattening :
- 298.257223563
- reference_ellipsoid_name :
- WGS 84
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- WGS 84
- horizontal_datum_name :
- World Geodetic System 1984
- grid_mapping_name :
- latitude_longitude
- spatial_ref :
- GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AXIS["Latitude",NORTH],AXIS["Longitude",EAST],AUTHORITY["EPSG","4326"]]
- gcps :
- {'type': 'FeatureCollection', 'features': [{'type': 'Feature', 'properties': {'id': 'f92abefc-acfd-42b0-9cc4-4ec52a1d914e', 'info': None, 'row': 0, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-67.84775397511133, 20.722083241325432, 0]}}, {'type': 'Feature', 'properties': {'id': '0be703ef-a4c8-4644-97b7-0f769c769db8', 'info': None, 'row': 0, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-67.97023959380435, 20.744666036125462, 0]}}, {'type': 'Feature', 'properties': {'id': '6d207fae-6271-4fdf-a599-470fb01979c7', 'info': None, 'row': 0, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.09276143156328, 20.767161696306058, 0]}}, {'type': 'Feature', 'properties': {'id': '9c35d6af-d9c9-4724-8f8e-eeef179c4b8d', 'info': None, 'row': 0, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.21531940308977, 20.789570095457513, 0]}}, {'type': 'Feature', 'properties': {'id': '06a0e99b-635b-49e9-aa09-b4c12989f784', 'info': None, 'row': 0, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.33791342173897, 20.811891107419683, 0]}}, {'type': 'Feature', 'properties': {'id': '104a2405-aa9b-4a2a-ba0d-2870ff798dc9', 'info': None, 'row': 0, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.46054339993769, 20.834124606360074, 0]}}, {'type': 'Feature', 'properties': {'id': '61cb08c0-3669-4f82-aca4-6b6024005fd4', 'info': None, 'row': 0, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.58320924930578, 20.856270466796982, 0]}}, {'type': 'Feature', 'properties': {'id': '364847ff-6e59-4bdb-8405-69a919ec4ac7', 'info': None, 'row': 0, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.70591088065385, 20.87832856359989, 0]}}, {'type': 'Feature', 'properties': {'id': '8c0d7b5e-7a4e-4a3a-b235-8e4321acc113', 'info': None, 'row': 0, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.82864820395255, 20.900298771984943, 0]}}, {'type': 'Feature', 'properties': {'id': '78b7c1d4-0bf3-4543-8ac0-1df3a89d0563', 'info': None, 'row': 0, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-68.95142112831574, 20.922180967513174, 0]}}, {'type': 'Feature', 'properties': {'id': 'd2b1e043-4a63-4dfb-b873-1a31f5508d4a', 'info': None, 'row': 0, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.07422956200863, 20.943975026093412, 0]}}, {'type': 'Feature', 'properties': {'id': 'b0d1083f-04e6-4d9e-b4b5-007043a28d3e', 'info': None, 'row': 0, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.19707341247357, 20.965680823988315, 0]}}, {'type': 'Feature', 'properties': {'id': 'e7571718-6a14-402c-845c-ff9655f9a67f', 'info': None, 'row': 0, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.31995258635963, 20.987298237821044, 0]}}, {'type': 'Feature', 'properties': {'id': '12140f88-373c-44e5-a216-be8a91700704', 'info': None, 'row': 0, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.44286698954143, 21.008827144580124, 0]}}, {'type': 'Feature', 'properties': {'id': '1bc6acf4-8614-43fc-a24f-31690a601558', 'info': None, 'row': 0, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.56581652711883, 21.030267421620866, 0]}}, {'type': 'Feature', 'properties': {'id': '9f2cde7f-0cf6-41fb-b948-19e041247565', 'info': None, 'row': 0, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.68880110339886, 21.05161894666391, 0]}}, {'type': 'Feature', 'properties': {'id': 'bee5a5c1-b300-4868-8637-f6a15af9bf85', 'info': None, 'row': 0, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.8118206218725, 21.072881597792932, 0]}}, {'type': 'Feature', 'properties': {'id': '11b1732d-baf3-4a9d-8dbb-ba107b1fb7bd', 'info': None, 'row': 0, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-69.93487498521344, 21.094055253456403, 0]}}, {'type': 'Feature', 'properties': {'id': '1b61b5f4-9d38-4061-a228-d25fce6d7f8c', 'info': None, 'row': 0, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.05796409534106, 21.11513979248025, 0]}}, {'type': 'Feature', 'properties': {'id': 'd16079d8-da0b-4f74-bff5-5520e064caf6', 'info': None, 'row': 0, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.18108773773331, 21.13613539382412, 0]}}, {'type': 'Feature', 'properties': {'id': 'e413ad96-0c96-48e0-a973-376796c91624', 'info': None, 'row': 9, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-67.86475708920521, 20.641064986177682, 0]}}, {'type': 'Feature', 'properties': {'id': '2adbc173-eb68-4d5e-8a9f-e50e5b256544', 'info': None, 'row': 9, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-67.98717673595714, 20.663653399193645, 0]}}, {'type': 'Feature', 'properties': {'id': '5924a1cd-26f0-4be1-89c7-bbcfa352cf95', 'info': None, 'row': 9, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.10963243740181, 20.68615504854947, 0]}}, {'type': 'Feature', 'properties': {'id': '24d8539e-84e1-434f-974e-b10edbb66a48', 'info': None, 'row': 9, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.23212410934363, 20.708569808120604, 0]}}, {'type': 'Feature', 'properties': {'id': '9d6a5e28-4506-4428-81ef-aead8ea1a0b5', 'info': None, 'row': 9, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.35465166624333, 20.73089755202884, 0]}}, {'type': 'Feature', 'properties': {'id': '94a8f733-68d1-4b79-88ba-64539dbc7f85', 'info': None, 'row': 9, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.47721502164272, 20.75313815472176, 0]}}, {'type': 'Feature', 'properties': {'id': 'da9a95cd-1914-4e42-99cf-6f418b31c5b6', 'info': None, 'row': 9, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.59981408828787, 20.77529149099606, 0]}}, {'type': 'Feature', 'properties': {'id': 'f24176f7-3be1-4b13-b973-130c07d91860', 'info': None, 'row': 9, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.72244877812581, 20.797357435997817, 0]}}, {'type': 'Feature', 'properties': {'id': 'd7888900-d9e7-4789-bcbb-f04690f6766b', 'info': None, 'row': 9, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.84511900227193, 20.819335865217553, 0]}}, {'type': 'Feature', 'properties': {'id': 'ffa20af6-e222-4bde-88bb-80044c795fbb', 'info': None, 'row': 9, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-68.96782467099112, 20.84122665448808, 0]}}, {'type': 'Feature', 'properties': {'id': '1c258704-fbd0-4973-acb9-cbc64ac80270', 'info': None, 'row': 9, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.09056569370442, 20.863029679987118, 0]}}, {'type': 'Feature', 'properties': {'id': '44d78927-5e78-4bc7-ab7e-99cc18ec457a', 'info': None, 'row': 9, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.21334197901446, 20.88474481824326, 0]}}, {'type': 'Feature', 'properties': {'id': 'b288c9b6-42c4-4484-bce4-e07e736fd597', 'info': None, 'row': 9, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.33615343473531, 20.90637194614272, 0]}}, {'type': 'Feature', 'properties': {'id': 'ed201d86-4f03-45ac-99fa-0fd4ec9a5c3c', 'info': None, 'row': 9, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.45899996791228, 20.9279109409343, 0]}}, {'type': 'Feature', 'properties': {'id': '80e6c4b0-2e5a-45a7-a603-39d47a9716e1', 'info': None, 'row': 9, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.58188148482299, 20.949361680231096, 0]}}, {'type': 'Feature', 'properties': {'id': '380e2e84-892d-437a-b444-12de1533a2d5', 'info': None, 'row': 9, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.70479789095984, 20.970724042009056, 0]}}, {'type': 'Feature', 'properties': {'id': 'd2d37fd6-c0a2-4d7a-9873-fb114908e1ea', 'info': None, 'row': 9, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.82774909100601, 20.991997904604588, 0]}}, {'type': 'Feature', 'properties': {'id': '5c951e3a-e80e-49d2-a676-c72e5b7c495d', 'info': None, 'row': 9, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-69.95073498883121, 21.01318314671569, 0]}}, {'type': 'Feature', 'properties': {'id': 'f6c9f3fd-9445-4378-b6ee-6e182f119baf', 'info': None, 'row': 9, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.0737554875473, 21.034279647413506, 0]}}, {'type': 'Feature', 'properties': {'id': '576167c4-56bb-4ec4-9cc3-201d7d8b602f', 'info': None, 'row': 9, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.19681037429092, 21.055287584636346, 0]}}, {'type': 'Feature', 'properties': {'id': 'c343f10d-c0bd-463c-90f2-aa6f225ddaab', 'info': None, 'row': 18, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-67.8817602032991, 20.560046731029917, 0]}}, {'type': 'Feature', 'properties': {'id': 'bdb92489-7c86-4741-96d9-2848308d73c8', 'info': None, 'row': 18, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.00411387810992, 20.582640762261832, 0]}}, {'type': 'Feature', 'properties': {'id': '77843cb6-9aed-4f3e-b573-c614de75868a', 'info': None, 'row': 18, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.12650344324037, 20.605148400792885, 0]}}, {'type': 'Feature', 'properties': {'id': 'a3f509bc-07b0-4a6f-8930-7495ac873339', 'info': None, 'row': 18, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.24892881559751, 20.62756952078369, 0]}}, {'type': 'Feature', 'properties': {'id': 'e8855e5a-9fe3-4081-a451-8d3b5dbaac35', 'info': None, 'row': 18, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.37138991074768, 20.649903996637995, 0]}}, {'type': 'Feature', 'properties': {'id': 'f30d4ca2-ff1a-41d1-b08a-d9711caf5881', 'info': None, 'row': 18, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.49388664334776, 20.67215170308344, 0]}}, {'type': 'Feature', 'properties': {'id': '2a4d47b1-32b1-4fb3-8e55-c06a752d229d', 'info': None, 'row': 18, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.61641892726996, 20.69431251519514, 0]}}, {'type': 'Feature', 'properties': {'id': 'e8e1ec2d-84d9-4b9c-8f0f-1df6c203f10a', 'info': None, 'row': 18, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.73898667559776, 20.716386308395744, 0]}}, {'type': 'Feature', 'properties': {'id': 'e0c96ff9-e686-4d9d-b48d-d9db1e7d2324', 'info': None, 'row': 18, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.8615898005913, 20.738372958450167, 0]}}, {'type': 'Feature', 'properties': {'id': '9c6fdbac-8a14-44fd-afbb-874a375ff5c6', 'info': None, 'row': 18, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-68.98422821366647, 20.76027234146298, 0]}}, {'type': 'Feature', 'properties': {'id': '7e83b894-178b-46eb-b0bc-e1379d01076f', 'info': None, 'row': 18, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.10690182540021, 20.782084333880825, 0]}}, {'type': 'Feature', 'properties': {'id': 'f536635e-1fc2-480d-b38a-53390a66a005', 'info': None, 'row': 18, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.22961054555536, 20.803808812498207, 0]}}, {'type': 'Feature', 'properties': {'id': '8cd3ebac-e761-4552-84e2-36236ccbe224', 'info': None, 'row': 18, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.35235428311097, 20.82544565446439, 0]}}, {'type': 'Feature', 'properties': {'id': '054385b6-3d3e-4ca0-a20d-07b96d1f65a9', 'info': None, 'row': 18, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.47513294628311, 20.846994737288473, 0]}}, {'type': 'Feature', 'properties': {'id': 'bbce6ae6-3679-4266-bbd4-381bcfc7607c', 'info': None, 'row': 18, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.59794644252713, 20.868455938841322, 0]}}, {'type': 'Feature', 'properties': {'id': 'e0f99589-5f7a-416c-b422-c1bd888422b9', 'info': None, 'row': 18, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.72079467852079, 20.889829137354198, 0]}}, {'type': 'Feature', 'properties': {'id': 'afbb117b-aadd-4201-99b1-1210a7e4cd8f', 'info': None, 'row': 18, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.84367756013953, 20.911114211416233, 0]}}, {'type': 'Feature', 'properties': {'id': '0fd27482-d89b-4e4b-b98f-6840f1376d61', 'info': None, 'row': 18, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-69.96659499244896, 20.93231103997498, 0]}}, {'type': 'Feature', 'properties': {'id': '0f50417a-e89b-4d21-8b14-7187a1443420', 'info': None, 'row': 18, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.08954687975356, 20.953419502346758, 0]}}, {'type': 'Feature', 'properties': {'id': 'a2005719-71b1-422d-a4d9-56c5c384621e', 'info': None, 'row': 18, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.21253301084852, 20.974439775448573, 0]}}, {'type': 'Feature', 'properties': {'id': '732514b8-0364-496d-b93d-1364feae3480', 'info': None, 'row': 27, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-67.898752692721, 20.479026455870112, 0]}}, {'type': 'Feature', 'properties': {'id': '11624b4d-d23c-4d2b-aee2-814e76613914', 'info': None, 'row': 27, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.02104095675365, 20.501626146323233, 0]}}, {'type': 'Feature', 'properties': {'id': '56aecd65-e5e4-4239-b2bc-83c59c9df34a', 'info': None, 'row': 27, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.14336494738866, 20.524139814326567, 0]}}, {'type': 'Feature', 'properties': {'id': '7210cc56-aa34-4fb9-9d5e-c803c9fcd588', 'info': None, 'row': 27, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.26572458261825, 20.546567334321946, 0]}}, {'type': 'Feature', 'properties': {'id': 'fa79cb71-0f05-4dad-957d-c0d3fe807bdc', 'info': None, 'row': 27, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.38811977911058, 20.568908580993746, 0]}}, {'type': 'Feature', 'properties': {'id': 'd39d35d7-d936-407c-ba88-14ef12109d71', 'info': None, 'row': 27, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.51055045263396, 20.59116342934833, 0]}}, {'type': 'Feature', 'properties': {'id': 'fb72322b-dc8d-49c1-9e5f-86724aec8c54', 'info': None, 'row': 27, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.63301651817791, 20.613331754737, 0]}}, {'type': 'Feature', 'properties': {'id': '019d5360-956b-4503-8a1a-20d6f061ea09', 'info': None, 'row': 27, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.755517889948, 20.635413432855795, 0]}}, {'type': 'Feature', 'properties': {'id': 'ef17a5a7-c0cc-4665-a2b3-144828e6b015', 'info': None, 'row': 27, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.87805448133128, 20.657408339740233, 0]}}, {'type': 'Feature', 'properties': {'id': 'a246796b-8fb3-436a-8a3d-7a53ebc79f99', 'info': None, 'row': 27, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.00062620487613, 20.679316351762825, 0]}}, {'type': 'Feature', 'properties': {'id': '9a21edc7-0b08-42b6-a0fb-612cfde7f6a2', 'info': None, 'row': 27, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.12323297229845, 20.701137345635626, 0]}}, {'type': 'Feature', 'properties': {'id': 'd0b4f6e9-7796-4035-8c14-0f02ee2d0a15', 'info': None, 'row': 27, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.24587469450732, 20.72287119841615, 0]}}, {'type': 'Feature', 'properties': {'id': '75f8e380-2456-4fd4-a744-08800ccf5e3e', 'info': None, 'row': 27, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.36855128163556, 20.744517787514326, 0]}}, {'type': 'Feature', 'properties': {'id': 'b40b612d-6db8-4593-8ea2-ff9b148c9781', 'info': None, 'row': 27, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.49126264306057, 20.766076990697503, 0]}}, {'type': 'Feature', 'properties': {'id': '2c412c7f-2b13-44f4-9419-56f4e1099bb0', 'info': None, 'row': 27, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.61400868740594, 20.787548686092318, 0]}}, {'type': 'Feature', 'properties': {'id': '32a9de7e-f726-42fd-93c8-1746a9d39fac', 'info': None, 'row': 27, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.73678932252366, 20.80893275218309, 0]}}, {'type': 'Feature', 'properties': {'id': 'd23f58d7-fdbb-4f35-81bc-309e1223cc3f', 'info': None, 'row': 27, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.85960445546814, 20.830229067809068, 0]}}, {'type': 'Feature', 'properties': {'id': '1d356505-e72a-4e01-b979-09188c1c7a77', 'info': None, 'row': 27, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-69.98245399248725, 20.851437512164747, 0]}}, {'type': 'Feature', 'properties': {'id': '952b7b04-518c-424e-9775-681aa011fa65', 'info': None, 'row': 27, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.10533783906979, 20.87255796480996, 0]}}, {'type': 'Feature', 'properties': {'id': '6c9c4073-465d-417e-9ef6-9cca3b7299cc', 'info': None, 'row': 27, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.22825578567607, 20.893590601644046, 0]}}, {'type': 'Feature', 'properties': {'id': '007e9956-4dba-450e-bc48-08b365583a4c', 'info': None, 'row': 36, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-67.91574279701247, 20.398005727238203, 0]}}, {'type': 'Feature', 'properties': {'id': '434eba9e-1492-497b-b75f-e8f40cd15751', 'info': None, 'row': 36, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.0379657762423, 20.420611086117802, 0]}}, {'type': 'Feature', 'properties': {'id': '5afbeedc-1921-4795-9dc3-abc931a15ef0', 'info': None, 'row': 36, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.16022431850443, 20.443130792639696, 0]}}, {'type': 'Feature', 'properties': {'id': '84c831bb-76e5-4b5f-a078-a051e20a40f2', 'info': None, 'row': 36, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.28251834287234, 20.465564721526057, 0]}}, {'type': 'Feature', 'properties': {'id': '90a85d80-d622-4b71-b643-0839696d818f', 'info': None, 'row': 36, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.4048477671152, 20.487912747741586, 0]}}, {'type': 'Feature', 'properties': {'id': '8cd945a2-8004-4385-b68a-e0272f58c4d3', 'info': None, 'row': 36, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.52721250811183, 20.51017474657108, 0]}}, {'type': 'Feature', 'properties': {'id': '9301a44a-97fd-43b7-ac70-0afd519284da', 'info': None, 'row': 36, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.64961248196714, 20.532350593641524, 0]}}, {'type': 'Feature', 'properties': {'id': '6901ec57-bad0-4c77-9f22-a7ea69a42d7e', 'info': None, 'row': 36, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.77204760400558, 20.554440164921626, 0]}}, {'type': 'Feature', 'properties': {'id': 'e42d0eda-7072-4408-9178-c8fb14a195fa', 'info': None, 'row': 36, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.89451778873709, 20.576443336716657, 0]}}, {'type': 'Feature', 'properties': {'id': 'cef16298-8609-40af-9eab-48709623cdab', 'info': None, 'row': 36, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.01702294983836, 20.598359985666228, 0]}}, {'type': 'Feature', 'properties': {'id': '8753dedc-4749-4c2a-911a-6f087ae024e2', 'info': None, 'row': 36, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.1395630001605, 20.620189988746993, 0]}}, {'type': 'Feature', 'properties': {'id': '33a70817-9b0d-4eef-8b55-49c6e47a1a9c', 'info': None, 'row': 36, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.26213785175563, 20.64193322327885, 0]}}, {'type': 'Feature', 'properties': {'id': '8666afd6-61a5-4103-90de-c0022504b4c0', 'info': None, 'row': 36, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.38474741590787, 20.663589566931822, 0]}}, {'type': 'Feature', 'properties': {'id': '19cfd89b-cac3-4ee8-940e-d8c1ce37ba04', 'info': None, 'row': 36, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.50739160315379, 20.68515889773109, 0]}}, {'type': 'Feature', 'properties': {'id': '1a7d7dbf-caa8-4ffe-b705-e80360a42fca', 'info': None, 'row': 36, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.63007032328314, 20.706641094058586, 0]}}, {'type': 'Feature', 'properties': {'id': '9ea9865c-d99e-4861-8f5d-5501cb986c81', 'info': None, 'row': 36, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.75278348531961, 20.728036034651186, 0]}}, {'type': 'Feature', 'properties': {'id': 'd32d5479-463a-43c4-aec7-5992390c8462', 'info': None, 'row': 36, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.8755309974936, 20.749343598597683, 0]}}, {'type': 'Feature', 'properties': {'id': 'ce1bd4d4-b648-4000-9f10-34fc994af67d', 'info': None, 'row': 36, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-69.99831276723219, 20.770563665338905, 0]}}, {'type': 'Feature', 'properties': {'id': '4d4b967a-b1ed-4347-82ee-c2cfb45b5932', 'info': None, 'row': 36, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.12112870120666, 20.79169611467784, 0]}}, {'type': 'Feature', 'properties': {'id': '961db61a-0c8d-4781-9790-0c35c3ed8dcb', 'info': None, 'row': 36, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.24397859154382, 20.812741121496984, 0]}}, {'type': 'Feature', 'properties': {'id': '3037b896-f0d7-4b16-96f4-58400957a830', 'info': None, 'row': 45, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-67.93272552336298, 20.31698358393159, 0]}}, {'type': 'Feature', 'properties': {'id': '14850c53-a352-46f0-9142-ef7a95c2a415', 'info': None, 'row': 45, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.05488361343701, 20.33959464010232, 0]}}, {'type': 'Feature', 'properties': {'id': 'bd65d8d8-8522-43e6-a801-e4f1e18e5d47', 'info': None, 'row': 45, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.17707710343109, 20.362120413511086, 0]}}, {'type': 'Feature', 'properties': {'id': '7282a256-79ba-4a5d-8ca1-00e072c9c674', 'info': None, 'row': 45, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.2993059135097, 20.384560779161685, 0]}}, {'type': 'Feature', 'properties': {'id': '5a1b73ad-213f-4461-b02c-e7ebcd97a2d0', 'info': None, 'row': 45, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.42156996253142, 20.406915612296512, 0]}}, {'type': 'Feature', 'properties': {'id': 'b54afb37-5392-4ff4-aa5b-98d478de9feb', 'info': None, 'row': 45, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.54386916846858, 20.429184788475194, 0]}}, {'type': 'Feature', 'properties': {'id': '0f0be530-83c9-4a72-8f43-dd8b318b58e4', 'info': None, 'row': 45, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.66620344852673, 20.451368183597275, 0]}}, {'type': 'Feature', 'properties': {'id': '11bdd03f-52d5-4301-b4d0-b6d73f0aa13f', 'info': None, 'row': 45, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.78857271913914, 20.473465673901906, 0]}}, {'type': 'Feature', 'properties': {'id': '3077eb01-c946-4103-bb71-09b036a64725', 'info': None, 'row': 45, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.91097689593259, 20.495477135962656, 0]}}, {'type': 'Feature', 'properties': {'id': '3071700f-231c-4e32-8b46-e2e9c50f947e', 'info': None, 'row': 45, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.03341589370807, 20.517402446685086, 0]}}, {'type': 'Feature', 'properties': {'id': '3dfbb59e-4a64-4a3f-8144-e986d10a90a5', 'info': None, 'row': 45, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.15588962644725, 20.539241483309315, 0]}}, {'type': 'Feature', 'properties': {'id': '3a868f2c-390c-4103-9fdd-2c302970f25f', 'info': None, 'row': 45, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.2783980073385, 20.56099412341603, 0]}}, {'type': 'Feature', 'properties': {'id': 'e1f18e97-c6b3-4d76-986b-99d6b990c34f', 'info': None, 'row': 45, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.4009409488071, 20.582660244933276, 0]}}, {'type': 'Feature', 'properties': {'id': '549e63cc-dbbd-4e93-8d6e-64004ff07d41', 'info': None, 'row': 45, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.52351836253573, 20.60423972614141, 0]}}, {'type': 'Feature', 'properties': {'id': '6601af51-0280-4805-96b6-04c061b2c295', 'info': None, 'row': 45, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.64613015946529, 20.625732445674807, 0]}}, {'type': 'Feature', 'properties': {'id': '2556ae78-45c8-4f56-9a64-6fa9755cf5a6', 'info': None, 'row': 45, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.76877624977631, 20.647138282520046, 0]}}, {'type': 'Feature', 'properties': {'id': '96a232f2-d9dd-456d-8d36-f897c74fbcf6', 'info': None, 'row': 45, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.89145654286278, 20.668457116013112, 0]}}, {'type': 'Feature', 'properties': {'id': '10799a5b-8a89-42a7-902d-6ea45bfe0bd1', 'info': None, 'row': 45, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.01417094732317, 20.689688825839653, 0]}}, {'type': 'Feature', 'properties': {'id': '892a18ef-4093-409a-90c3-1f090d80b8fb', 'info': None, 'row': 45, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.13691937100883, 20.71083329204525, 0]}}, {'type': 'Feature', 'properties': {'id': 'aa3ff6e8-62a1-4ac3-891f-fa52b20a6585', 'info': None, 'row': 45, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.25970160771438, 20.731890688497593, 0]}}, {'type': 'Feature', 'properties': {'id': 'aea2e7ac-b87a-4348-807f-2b7bcbf15be6', 'info': None, 'row': 54, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-67.94970288265088, 20.235960411523795, 0]}}, {'type': 'Feature', 'properties': {'id': 'b1338b03-6998-4c5a-9084-3e4663355513', 'info': None, 'row': 54, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.07179637138144, 20.258577185983167, 0]}}, {'type': 'Feature', 'properties': {'id': '0e044ae8-8004-411e-8fbc-35571e055e48', 'info': None, 'row': 54, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.19392509725277, 20.281109046915244, 0]}}, {'type': 'Feature', 'properties': {'id': '4157d695-dc41-49e2-9267-e63ca67a737f', 'info': None, 'row': 54, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.31608898152757, 20.30355586960642, 0]}}, {'type': 'Feature', 'properties': {'id': '1b91a9d5-a712-4e9d-8ee9-b4da5737e196', 'info': None, 'row': 54, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.43828794414532, 20.325917529574827, 0]}}, {'type': 'Feature', 'properties': {'id': '2ee967e4-20aa-4d85-b5eb-18452e92c505', 'info': None, 'row': 54, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.56052190415947, 20.348193902652312, 0]}}, {'type': 'Feature', 'properties': {'id': '8cc99803-bbee-4899-98a4-b129c7fb1efb', 'info': None, 'row': 54, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.6827907798655, 20.370384865008713, 0]}}, {'type': 'Feature', 'properties': {'id': 'ffa7b754-fd13-4792-90cd-b5e2e38fc799', 'info': None, 'row': 54, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.80509448879816, 20.392490293152015, 0]}}, {'type': 'Feature', 'properties': {'id': '137a160d-f862-41f2-aa8f-b89aa2febc66', 'info': None, 'row': 54, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.9274329476968, 20.41451006392298, 0]}}, {'type': 'Feature', 'properties': {'id': '9a2bdf47-2c31-4cba-b6f7-b357d88e70d4', 'info': None, 'row': 54, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.04980607248356, 20.436444054492338, 0]}}, {'type': 'Feature', 'properties': {'id': '33f66bb4-8e20-4fa1-882f-9c837b29d626', 'info': None, 'row': 54, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.17221377826749, 20.458292142362822, 0]}}, {'type': 'Feature', 'properties': {'id': '82770962-fa2f-4b68-a37a-7a5531e42105', 'info': None, 'row': 54, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.29465597936814, 20.480054205374767, 0]}}, {'type': 'Feature', 'properties': {'id': 'de209332-e6d9-4920-91e5-1b0431201504', 'info': None, 'row': 54, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.41713258934473, 20.501730121712683, 0]}}, {'type': 'Feature', 'properties': {'id': '74c481c7-ccee-4148-a711-45d955c53ed7', 'info': None, 'row': 54, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.53964352101639, 20.523319769910245, 0]}}, {'type': 'Feature', 'properties': {'id': '954574d9-bfbb-44fc-bc6d-c1902d313e1f', 'info': None, 'row': 54, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.66218868646428, 20.544823028852118, 0]}}, {'type': 'Feature', 'properties': {'id': 'a2bf77db-6576-4325-97e6-37c76fccedca', 'info': None, 'row': 54, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.78476799701502, 20.566239777772566, 0]}}, {'type': 'Feature', 'properties': {'id': '9e08f23a-4509-4b4a-b704-ae280e0c6634', 'info': None, 'row': 54, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.90738136321716, 20.587569896253036, 0]}}, {'type': 'Feature', 'properties': {'id': '4c6f129a-f86a-46ac-a194-2737004b61ca', 'info': None, 'row': 54, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.03002869483477, 20.608813264222896, 0]}}, {'type': 'Feature', 'properties': {'id': '5523e531-06dc-46aa-b66f-18384f8b4a91', 'info': None, 'row': 54, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.15270990089766, 20.62996976196991, 0]}}, {'type': 'Feature', 'properties': {'id': '0f658142-01db-4c9e-b65a-882d6803e6ea', 'info': None, 'row': 54, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.27542477686913, 20.651039562348416, 0]}}, {'type': 'Feature', 'properties': {'id': 'e339c985-d0a5-43a6-8789-2de5e62e79bb', 'info': None, 'row': 63, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-67.96667600418373, 20.1549362685391, 0]}}, {'type': 'Feature', 'properties': {'id': 'c9ba08ba-0abf-4d2b-a0b5-8c7e5de1aea6', 'info': None, 'row': 63, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.08870514272519, 20.17755877647519, 0]}}, {'type': 'Feature', 'properties': {'id': 'bfe3dc6b-11cc-455b-ac79-b08fa29e61e9', 'info': None, 'row': 63, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.21076932555312, 20.20009673420292, 0]}}, {'type': 'Feature', 'properties': {'id': '927eec22-35a4-4924-ab52-e5cb74822b80', 'info': None, 'row': 63, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.33286851449445, 20.222550024613998, 0]}}, {'type': 'Feature', 'properties': {'id': '9f6c5164-29da-4da6-a9dd-de6ffd58c211', 'info': None, 'row': 63, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.45500263051453, 20.24491852347264, 0]}}, {'type': 'Feature', 'properties': {'id': 'db94f31f-5260-4874-b6bd-2eb3ed9c5831', 'info': None, 'row': 63, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.57717158451639, 20.267202105179415, 0]}}, {'type': 'Feature', 'properties': {'id': 'fbc7e085-8d7b-40ec-a644-c058bd32afd0', 'info': None, 'row': 63, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.69937529027209, 20.289400645154718, 0]}}, {'type': 'Feature', 'properties': {'id': '637c6104-1c00-4287-9252-5f652bbad75a', 'info': None, 'row': 63, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.82161366701874, 20.311514020296034, 0]}}, {'type': 'Feature', 'properties': {'id': 'f063080b-15be-4259-8587-6cb9c1610cb9', 'info': None, 'row': 63, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.94388663704102, 20.333542108520696, 0]}}, {'type': 'Feature', 'properties': {'id': '65f383c3-d8bd-4fc6-a8cc-cd926dafbd62', 'info': None, 'row': 63, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.06619412184689, 20.355484788066963, 0]}}, {'type': 'Feature', 'properties': {'id': '02bab970-cd7e-455d-9e61-525f0659880e', 'info': None, 'row': 63, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.18853603919428, 20.377341936963912, 0]}}, {'type': 'Feature', 'properties': {'id': 'f52f5ecf-88f6-4908-9a75-6c72c80fc688', 'info': None, 'row': 63, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.31091230213873, 20.399113432874103, 0]}}, {'type': 'Feature', 'properties': {'id': '210f20b2-b28a-4377-adc0-0ff69b7743a7', 'info': None, 'row': 63, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.43332282037782, 20.420799153347332, 0]}}, {'type': 'Feature', 'properties': {'id': '3ff2a777-841a-485e-8403-3b1fc54a0a0b', 'info': None, 'row': 63, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.55576750341274, 20.442398976389075, 0]}}, {'type': 'Feature', 'properties': {'id': '4c8c012a-449b-478c-be8d-166f1440a693', 'info': None, 'row': 63, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.67824626439291, 20.46391278113515, 0]}}, {'type': 'Feature', 'properties': {'id': '6f4d0da0-4cbf-44ca-9eb0-a27df6eedda5', 'info': None, 'row': 63, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.80075902292967, 20.48534044832924, 0]}}, {'type': 'Feature', 'properties': {'id': '16e2a371-0ad7-4c4a-9f77-e654b04b36c5', 'info': None, 'row': 63, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.9233057046352, 20.506681860218116, 0]}}, {'type': 'Feature', 'properties': {'id': 'e6559843-db1a-4a3c-9194-bdbb501b4c1b', 'info': None, 'row': 63, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.04588623465179, 20.527936899407383, 0]}}, {'type': 'Feature', 'properties': {'id': '9de399e7-91d0-45b1-9556-bcff6eddc780', 'info': None, 'row': 63, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.16850052197, 20.549105446156346, 0]}}, {'type': 'Feature', 'properties': {'id': 'e80dd9c4-9d7e-490e-9e16-f4216fae3c56', 'info': None, 'row': 63, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.29114831881924, 20.570187664536032, 0]}}, {'type': 'Feature', 'properties': {'id': 'a8ca5ddb-1b18-43be-8f94-344dcc08d30d', 'info': None, 'row': 72, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-67.98364094008546, 20.073910250791858, 0]}}, {'type': 'Feature', 'properties': {'id': 'ab40e216-6aed-415f-b7ff-699e03c630d8', 'info': None, 'row': 72, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.10560621356666, 20.0965385215419, 0]}}, {'type': 'Feature', 'properties': {'id': '7503950a-be36-4737-a699-ec7848a25ba3', 'info': None, 'row': 72, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.2276062803871, 20.119082593975676, 0]}}, {'type': 'Feature', 'properties': {'id': 'b0ccda58-3fa6-490f-b1a4-deb328577e61', 'info': None, 'row': 72, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.34964121917066, 20.14154237273482, 0]}}, {'type': 'Feature', 'properties': {'id': '795fd8ec-3536-440f-9993-e179bc7bfc28', 'info': None, 'row': 72, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.47171095180187, 20.163917733772294, 0]}}, {'type': 'Feature', 'properties': {'id': '77e68121-7738-4e9f-a097-a78502de884c', 'info': None, 'row': 72, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.59381536320211, 20.186208546766906, 0]}}, {'type': 'Feature', 'properties': {'id': '8212de8d-4f98-4faf-86cb-cee052d7fe0f', 'info': None, 'row': 72, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.71595435177709, 20.20841468441992, 0]}}, {'type': 'Feature', 'properties': {'id': 'b0f4b6e0-5913-4ac0-8f9e-3357cf02bcaa', 'info': None, 'row': 72, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.8381278396269, 20.230536024251414, 0]}}, {'type': 'Feature', 'properties': {'id': '09d7bcfc-2d61-428d-8aba-acdc9955c958', 'info': None, 'row': 72, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.96033576314402, 20.252572446818654, 0]}}, {'type': 'Feature', 'properties': {'id': 'd0b12f8a-85fd-4189-a7ab-fd5d3e0217b9', 'info': None, 'row': 72, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.08257805804604, 20.274523832977255, 0]}}, {'type': 'Feature', 'properties': {'id': '3aa50b13-31bb-467c-b726-755bea58023e', 'info': None, 'row': 72, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.20485464767872, 20.29639006179208, 0]}}, {'type': 'Feature', 'properties': {'id': '98d52fbd-c565-43bd-a674-190b2fc2aa3c', 'info': None, 'row': 72, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.32716543920694, 20.318171009903004, 0]}}, {'type': 'Feature', 'properties': {'id': '7fe7fbbd-cc6d-4855-8992-0e3e3453a235', 'info': None, 'row': 72, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.44951032881747, 20.339866552503672, 0]}}, {'type': 'Feature', 'properties': {'id': 'bb618a54-5e80-4d78-bb1d-abee92e27e8c', 'info': None, 'row': 72, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.5718892140892, 20.3614765655618, 0]}}, {'type': 'Feature', 'properties': {'id': '40e36fa5-8174-409a-8319-5b6febb84143', 'info': None, 'row': 72, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.694302009101, 20.383000928466025, 0]}}, {'type': 'Feature', 'properties': {'id': 'b1d1d82e-9e9d-4ab7-afa1-319141935454', 'info': None, 'row': 72, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.81674865553764, 20.404439525906632, 0]}}, {'type': 'Feature', 'properties': {'id': '874bf38c-6c11-4160-a0b4-2954858ef29e', 'info': None, 'row': 72, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.93922912094166, 20.425792247469882, 0]}}, {'type': 'Feature', 'properties': {'id': 'ad0cd19a-2cae-402b-8457-2da63252dd9f', 'info': None, 'row': 72, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.06174337328672, 20.4470589831363, 0]}}, {'type': 'Feature', 'properties': {'id': 'c8f18166-1c06-44a1-b087-09f30d1b148d', 'info': None, 'row': 72, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.18429131917208, 20.468239612613885, 0]}}, {'type': 'Feature', 'properties': {'id': '7a85c6d1-39db-44e9-a2ad-bbed10b70d90', 'info': None, 'row': 72, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.30687258085943, 20.489334276182017, 0]}}, {'type': 'Feature', 'properties': {'id': '9cd29437-0a7a-4503-b064-f70588b8cedc', 'info': None, 'row': 81, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-68.00060463198908, 19.992883944644007, 0]}}, {'type': 'Feature', 'properties': {'id': '59c97b33-5811-44bb-88e1-4db4ff7b8e6c', 'info': None, 'row': 81, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.12250609288628, 20.015517978461965, 0]}}, {'type': 'Feature', 'properties': {'id': '99afdc25-a18a-49c1-b99e-75c64c6026b2', 'info': None, 'row': 81, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.24444212551239, 20.038068171200536, 0]}}, {'type': 'Feature', 'properties': {'id': '70e97be1-52d2-4f64-88cd-9849d447ddb5', 'info': None, 'row': 81, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.36641288751053, 20.060534442236584, 0]}}, {'type': 'Feature', 'properties': {'id': 'e8109b0a-8a35-4e3a-b600-2ab7e3601190', 'info': None, 'row': 81, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.48841830130945, 20.08291666766088, 0]}}, {'type': 'Feature', 'properties': {'id': '94192af6-77a7-4490-a2b6-2e0d273f83ba', 'info': None, 'row': 81, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.61045823458053, 20.105214714043147, 0]}}, {'type': 'Feature', 'properties': {'id': '49e9c20f-1f2e-4ffa-9f82-68fd7828ad2a', 'info': None, 'row': 81, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.73253257597224, 20.12742845238555, 0]}}, {'type': 'Feature', 'properties': {'id': '5dcbb36a-cb38-4d36-9a37-102fc33fba5f', 'info': None, 'row': 81, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.8546412501751, 20.14955776076992, 0]}}, {'type': 'Feature', 'properties': {'id': '9d33da76-1b9e-41e8-a99d-24f3059161e9', 'info': None, 'row': 81, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.97678420353712, 20.171602521633833, 0]}}, {'type': 'Feature', 'properties': {'id': '6d59a967-c1bf-47cd-a351-a4690e602d29', 'info': None, 'row': 81, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.09896138147388, 20.193562617637863, 0]}}, {'type': 'Feature', 'properties': {'id': '2c100914-dcbe-42cc-822f-1896cfbd394b', 'info': None, 'row': 81, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.22117271100315, 20.215437928547313, 0]}}, {'type': 'Feature', 'properties': {'id': 'faae1783-d072-407a-9b5f-06949d36e367', 'info': None, 'row': 81, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.3434180953271, 20.237228330333807, 0]}}, {'type': 'Feature', 'properties': {'id': '975c0a9f-5f49-4958-9b3b-0a0e62723585', 'info': None, 'row': 81, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.46569742206906, 20.25893369671921, 0]}}, {'type': 'Feature', 'properties': {'id': 'aca9b24b-167a-4546-a5c3-289515f8d1ea', 'info': None, 'row': 81, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.588010582251, 20.280553902577708, 0]}}, {'type': 'Feature', 'properties': {'id': 'c5e9902e-713b-4ae5-b79e-bbc74ee810bb', 'info': None, 'row': 81, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.71035749315705, 20.302088827936824, 0]}}, {'type': 'Feature', 'properties': {'id': '17d44f5f-b6c2-4308-bc79-021b7a77daeb', 'info': None, 'row': 81, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.8327381147005, 20.323538360742052, 0]}}, {'type': 'Feature', 'properties': {'id': '24fd946d-716b-4f38-bced-6c48436ac0fe', 'info': None, 'row': 81, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.95515244568611, 20.34490239604981, 0]}}, {'type': 'Feature', 'properties': {'id': '09b686d6-3f6a-4804-8a26-ba6a0c768481', 'info': None, 'row': 81, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.07760048334194, 20.366180828871382, 0]}}, {'type': 'Feature', 'properties': {'id': 'f02c0ffe-24b8-4404-a4a7-f923a2eb043b', 'info': None, 'row': 81, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.2000821266256, 20.387373537494526, 0]}}, {'type': 'Feature', 'properties': {'id': '53381e66-5f6a-4827-ab0c-99fdd0c40094', 'info': None, 'row': 81, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.32259689231039, 20.408480642661647, 0]}}, {'type': 'Feature', 'properties': {'id': '31219b96-b8ec-4fd7-a352-52760f0f9298', 'info': None, 'row': 90, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-68.01755752919904, 19.911855135923187, 0]}}, {'type': 'Feature', 'properties': {'id': 'e46c4aa9-0b95-4d90-a94d-24b032f65dad', 'info': None, 'row': 90, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.13939563287144, 19.934494935012854, 0]}}, {'type': 'Feature', 'properties': {'id': '77a24067-7932-4da3-bb26-6f855a07b35e', 'info': None, 'row': 90, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.26126834122967, 19.957051296638824, 0]}}, {'type': 'Feature', 'properties': {'id': 'b36c1add-4b8f-4a4f-9cb7-f64c59096181', 'info': None, 'row': 90, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.38317556312546, 19.97952409404396, 0]}}, {'type': 'Feature', 'properties': {'id': '5aa03328-3fbd-485a-b19c-6dabb939751a', 'info': None, 'row': 90, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.50511721827638, 20.001913203014695, 0]}}, {'type': 'Feature', 'properties': {'id': 'ef811067-d43d-4895-8b1a-061b4e6a4a6d', 'info': None, 'row': 90, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.62709323287297, 20.024218501005507, 0]}}, {'type': 'Feature', 'properties': {'id': 'a7b3d666-eb29-4d14-bfbb-2f8f408b22b5', 'info': None, 'row': 90, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.74910353447895, 20.046439866171, 0]}}, {'type': 'Feature', 'properties': {'id': 'e81e2376-6e64-4176-86b1-4cdf6c13b2bb', 'info': None, 'row': 90, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.87114804800932, 20.068577176626512, 0]}}, {'type': 'Feature', 'properties': {'id': '4b4ce874-9585-4596-8ee5-7e8294540810', 'info': None, 'row': 90, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-68.99322669373784, 20.09063031009838, 0]}}, {'type': 'Feature', 'properties': {'id': 'b462691d-b037-4e4f-9e1c-b95b4ac8671b', 'info': None, 'row': 90, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.11533938762821, 20.112599144002864, 0]}}, {'type': 'Feature', 'properties': {'id': '5cf4a6d6-149b-4060-a8e4-f58fca30d010', 'info': None, 'row': 90, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.23748604374572, 20.134483555895425, 0]}}, {'type': 'Feature', 'properties': {'id': 'b2eb4b48-125d-434c-9dde-51ea47001ec8', 'info': None, 'row': 90, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.35966657805962, 20.156283424155312, 0]}}, {'type': 'Feature', 'properties': {'id': 'ceeffcce-b5fc-41b7-9c0f-84f54586b9a9', 'info': None, 'row': 90, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.48188091255972, 20.177998628706522, 0]}}, {'type': 'Feature', 'properties': {'id': '521fcec8-80cd-42db-90b7-fbf950a76cce', 'info': None, 'row': 90, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.60412897826947, 20.199629051523132, 0]}}, {'type': 'Feature', 'properties': {'id': 'b57ae29d-b473-4f4e-82da-6c69e55ed3ae', 'info': None, 'row': 90, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.72641071542616, 20.221174576621756, 0]}}, {'type': 'Feature', 'properties': {'id': 'e8b45a7b-76c6-4724-8d20-c71bbf53da70', 'info': None, 'row': 90, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.84872606880768, 20.242635089203667, 0]}}, {'type': 'Feature', 'properties': {'id': '97d7efdd-10f1-4c7c-acae-7f6ea7aa8ca3', 'info': None, 'row': 90, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.97107497590858, 20.264010473574146, 0]}}, {'type': 'Feature', 'properties': {'id': 'f70936b4-14b8-497d-9982-360fe174c8d5', 'info': None, 'row': 90, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.09345734539902, 20.285300609434252, 0]}}, {'type': 'Feature', 'properties': {'id': '15e6e699-4829-4641-a40e-5923990ab5a0', 'info': None, 'row': 90, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.21587302303513, 20.306505366111082, 0]}}, {'type': 'Feature', 'properties': {'id': '73557987-da7f-4c1a-ab5f-0eb4f561cf26', 'info': None, 'row': 90, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.3383216325194, 20.32762488173005, 0]}}, {'type': 'Feature', 'properties': {'id': '4920470f-123a-47b8-8f8b-264befd5af30', 'info': None, 'row': 99, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-68.03451042640907, 19.830826327202367, 0]}}, {'type': 'Feature', 'properties': {'id': '7ecc931d-aec9-4624-851e-b5beab7a2911', 'info': None, 'row': 99, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.15628517285661, 19.85347189156374, 0]}}, {'type': 'Feature', 'properties': {'id': '01245b5a-92b9-4401-852d-c3a9f8f29d46', 'info': None, 'row': 99, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.27809455694697, 19.876034422077108, 0]}}, {'type': 'Feature', 'properties': {'id': 'a26386e3-6f20-4110-ba11-d628b5f2788f', 'info': None, 'row': 99, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.39993823874039, 19.89851374585134, 0]}}, {'type': 'Feature', 'properties': {'id': 'b9f55076-b0ea-4c18-b1be-5dbf1d52ee88', 'info': None, 'row': 99, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.52181613524334, 19.920909738368515, 0]}}, {'type': 'Feature', 'properties': {'id': 'f617735e-95ff-4264-91b5-bd258f6d134a', 'info': None, 'row': 99, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.64372823116541, 19.943222287967867, 0]}}, {'type': 'Feature', 'properties': {'id': '7c606de1-ae91-4dfa-9b3b-fae29733717f', 'info': None, 'row': 99, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.76567449298564, 19.965451279956454, 0]}}, {'type': 'Feature', 'properties': {'id': '4f68e784-7cec-4654-a1b9-e6a824f5c7a5', 'info': None, 'row': 99, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.88765484584351, 19.987596592483108, 0]}}, {'type': 'Feature', 'properties': {'id': '8b389bdb-6867-408a-a63c-fedfe670c01a', 'info': None, 'row': 99, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-69.00966918393856, 20.009658098562934, 0]}}, {'type': 'Feature', 'properties': {'id': 'e4214fb8-a8a4-4a14-9e01-0d5ee5132101', 'info': None, 'row': 99, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.13171739378252, 20.03163567036787, 0]}}, {'type': 'Feature', 'properties': {'id': 'c1c7625b-65db-49e2-aaa3-4597dadfb649', 'info': None, 'row': 99, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.2537993764883, 20.05352918324354, 0]}}, {'type': 'Feature', 'properties': {'id': '042d3e80-f33f-4206-96d9-bdcb3174b745', 'info': None, 'row': 99, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.37591506079212, 20.075338517976824, 0]}}, {'type': 'Feature', 'properties': {'id': '43720c72-4738-450d-b137-f95b84143a29', 'info': None, 'row': 99, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.49806440305039, 20.097063560693837, 0]}}, {'type': 'Feature', 'properties': {'id': '9c518934-440f-4733-96e9-d0610490cab9', 'info': None, 'row': 99, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.62024737428797, 20.118704200468557, 0]}}, {'type': 'Feature', 'properties': {'id': '0e633388-7ddf-428a-b30b-577b82d4fe17', 'info': None, 'row': 99, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.74246393769529, 20.140260325306688, 0]}}, {'type': 'Feature', 'properties': {'id': 'd46efd1d-4f4c-4f75-8bd7-bd67a242e6b6', 'info': None, 'row': 99, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.86471402291484, 20.16173181766528, 0]}}, {'type': 'Feature', 'properties': {'id': '4cef1246-906d-4eee-b746-bb96dbbaa347', 'info': None, 'row': 99, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-69.98699750613109, 20.18311855109848, 0]}}, {'type': 'Feature', 'properties': {'id': '5e19f248-d7f1-4426-928e-ea94e472bc9a', 'info': None, 'row': 99, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.1093142074561, 20.204420389997118, 0]}}, {'type': 'Feature', 'properties': {'id': '014ac1ef-d697-49ec-8adc-330832eafc26', 'info': None, 'row': 99, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.23166391944466, 20.225637194727636, 0]}}, {'type': 'Feature', 'properties': {'id': '3816b2bb-10d2-40a5-8b84-d170a66460be', 'info': None, 'row': 99, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.3540463727284, 20.24676912079845, 0]}}, {'type': 'Feature', 'properties': {'id': '9780faca-2acd-4f9d-9a5e-3739c7564298', 'info': None, 'row': 108, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-68.05145285923709, 19.749795459293594, 0]}}, {'type': 'Feature', 'properties': {'id': 'a5586d57-cd0e-407d-82a5-e216f0061355', 'info': None, 'row': 108, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.17316503358896, 19.77244686901027, 0]}}, {'type': 'Feature', 'properties': {'id': '57141b1a-9137-4e49-9eaa-2b606e520e73', 'info': None, 'row': 108, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.29491154878029, 19.79501558637744, 0]}}, {'type': 'Feature', 'properties': {'id': '8af063b8-7e73-48dc-844d-39641ce53f0b', 'info': None, 'row': 108, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.41669223135591, 19.817501469575664, 0]}}, {'type': 'Feature', 'properties': {'id': '07e0f9dd-bf31-4936-ae77-4e951acc00c4', 'info': None, 'row': 108, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.53850701470395, 19.83990439711138, 0]}}, {'type': 'Feature', 'properties': {'id': '76f79ef6-154f-4185-8938-4e1465967f60', 'info': None, 'row': 108, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.6603558507789, 19.86222425130994, 0]}}, {'type': 'Feature', 'properties': {'id': '0fd94312-9232-4b3d-ad60-a968076b7700', 'info': None, 'row': 108, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.78223867574981, 19.884460912001824, 0]}}, {'type': 'Feature', 'properties': {'id': '9ca2c60b-525b-4faf-a6a2-caf4984fb0fe', 'info': None, 'row': 108, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.9041554063264, 19.90661425591543, 0]}}, {'type': 'Feature', 'properties': {'id': '928aded2-2507-4ac0-95cf-5fbce665318b', 'info': None, 'row': 108, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-69.02610595027627, 19.928684158645037, 0]}}, {'type': 'Feature', 'properties': {'id': 'a5d67b6e-44db-418e-940f-e2c31b241d17', 'info': None, 'row': 108, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.14809021992126, 19.950670497106838, 0]}}, {'type': 'Feature', 'properties': {'id': '01daad42-7311-4216-8e61-a4ba6e4b7851', 'info': None, 'row': 108, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.27010814160037, 19.972573151213016, 0]}}, {'type': 'Feature', 'properties': {'id': '0c5dab13-9e05-490f-93ed-368380babc30', 'info': None, 'row': 108, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.39215965750424, 19.994392004144107, 0]}}, {'type': 'Feature', 'properties': {'id': 'ea9fb6ae-e53d-4333-a98b-88a34ab9ae58', 'info': None, 'row': 108, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.51424471913464, 20.016126941126803, 0]}}, {'type': 'Feature', 'properties': {'id': '1d856c72-36d8-45fe-a07c-4c28405f8e93', 'info': None, 'row': 108, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.63636327407876, 20.037777847059278, 0]}}, {'type': 'Feature', 'properties': {'id': 'e74d9332-eebd-4945-ab17-ee26cf8a78dc', 'info': None, 'row': 108, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.75851524992427, 20.059344603692388, 0]}}, {'type': 'Feature', 'properties': {'id': '96e3936c-d0dc-4d66-b1fb-57276b38fd3b', 'info': None, 'row': 108, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.88070054106032, 20.080827087389075, 0]}}, {'type': 'Feature', 'properties': {'id': '6d562e76-446e-4e62-9813-1b47dc06ab2e', 'info': None, 'row': 108, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-70.00291900587558, 20.102225168758558, 0]}}, {'type': 'Feature', 'properties': {'id': '916d6cd2-fdcc-4836-9d17-f3fb9fd50d99', 'info': None, 'row': 108, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.1251704835197, 20.123538715705347, 0]}}, {'type': 'Feature', 'properties': {'id': '65746665-c59e-42f4-90a3-7a50df251c9e', 'info': None, 'row': 108, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.2474548409754, 20.14476760165254, 0]}}, {'type': 'Feature', 'properties': {'id': 'f8f833d4-d77a-457b-8874-580a571407ab', 'info': None, 'row': 108, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.3697719518644, 20.165912005458267, 0]}}, {'type': 'Feature', 'properties': {'id': 'c17cc089-32ed-47b5-8aba-c806a6d3887b', 'info': None, 'row': 117, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-68.06839366666046, 19.66876427153662, 0]}}, {'type': 'Feature', 'properties': {'id': '13cd74f3-1150-479a-81f7-2d4187152ffe', 'info': None, 'row': 117, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.19004339086868, 19.691421539047788, 0]}}, {'type': 'Feature', 'properties': {'id': '18e41f5c-9ead-468f-a142-97240eac6d95', 'info': None, 'row': 117, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.31172710789225, 19.713996446059426, 0]}}, {'type': 'Feature', 'properties': {'id': '0ab075d6-ab7b-47b2-ab68-dabf18493993', 'info': None, 'row': 117, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.4334448752642, 19.736488893815977, 0]}}, {'type': 'Feature', 'properties': {'id': '6160ce24-a7d2-42db-935a-de6d2bc29d01', 'info': None, 'row': 117, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.55519664572007, 19.75889876436525, 0]}}, {'type': 'Feature', 'properties': {'id': '73f666c1-0278-4bb5-b9d5-1ca3c946f0a7', 'info': None, 'row': 117, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.6769823242818, 19.781225931393926, 0]}}, {'type': 'Feature', 'properties': {'id': 'bf2aa35f-0c99-47d7-9e84-dc3012c40e27', 'info': None, 'row': 117, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.79880180605588, 19.803470267294244, 0]}}, {'type': 'Feature', 'properties': {'id': '6ba0f4dd-94fc-4ee5-8d5f-37382336769a', 'info': None, 'row': 117, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.92065499797806, 19.82563164714835, 0]}}, {'type': 'Feature', 'properties': {'id': 'd34b59b7-33b4-4b49-abf2-f83c826609e2', 'info': None, 'row': 117, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-69.04254182754157, 19.847709950262086, 0]}}, {'type': 'Feature', 'properties': {'id': '39fe439e-5f49-443c-8cc8-79d23461f6d7', 'info': None, 'row': 117, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.16446224146192, 19.869705059847412, 0]}}, {'type': 'Feature', 'properties': {'id': 'd1fe4e06-fab8-4307-b61e-166c9092116f', 'info': None, 'row': 117, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.28641619723453, 19.89161686143562, 0]}}, {'type': 'Feature', 'properties': {'id': '6033084d-c4c8-4406-8ed0-d9b6925fb8f0', 'info': None, 'row': 117, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.40840365061116, 19.913445240598737, 0]}}, {'type': 'Feature', 'properties': {'id': '8ec46c8b-fd89-4241-9f71-d0dfc3a5da74', 'info': None, 'row': 117, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.53042454214678, 19.93519008056096, 0]}}, {'type': 'Feature', 'properties': {'id': '514f841e-e826-409e-b9a1-7d8399d04f4a', 'info': None, 'row': 117, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.65247878613712, 19.95685126029323, 0]}}, {'type': 'Feature', 'properties': {'id': '314ff777-eabb-496c-b7ac-e8d517dcf82a', 'info': None, 'row': 117, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.7745662654718, 19.978428653700412, 0]}}, {'type': 'Feature', 'properties': {'id': 'f105ab59-ae1c-4ddf-afd5-71edebfafdb1', 'info': None, 'row': 117, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.89668683616173, 19.999922130531004, 0]}}, {'type': 'Feature', 'properties': {'id': 'c301d55b-8728-45d9-9c79-03609849fff1', 'info': None, 'row': 117, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-70.01884034555869, 20.021331559661803, 0]}}, {'type': 'Feature', 'properties': {'id': 'acb59a1f-4b87-4a22-be78-77b85747dfe4', 'info': None, 'row': 117, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.1410266685625, 20.04265681543487, 0]}}, {'type': 'Feature', 'properties': {'id': 'c51ca337-9148-4ba6-a274-84b8c3cce767', 'info': None, 'row': 117, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.26324576640815, 20.06389778774986, 0]}}, {'type': 'Feature', 'properties': {'id': 'c378fa29-1f44-4324-8681-ec9466008c63', 'info': None, 'row': 117, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.3854976613087, 20.085054679741404, 0]}}, {'type': 'Feature', 'properties': {'id': 'a06adca5-6b64-49e7-8444-dbe3a3dca6fd', 'info': None, 'row': 126, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-68.08532719228799, 19.58773163659993, 0]}}, {'type': 'Feature', 'properties': {'id': '0e267a3d-d44e-43f2-bb49-271c6fbab6a7', 'info': None, 'row': 126, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.20691476416941, 19.610394769603598, 0]}}, {'type': 'Feature', 'properties': {'id': 'f3e38ca8-ac16-4f3c-8bff-5c88569ba327', 'info': None, 'row': 126, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.3285361711987, 19.632975908785617, 0]}}, {'type': 'Feature', 'properties': {'id': 'c6e72163-5edd-4a74-9fd3-1139cf140771', 'info': None, 'row': 126, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.45019148547875, 19.65547495813271, 0]}}, {'type': 'Feature', 'properties': {'id': '5552e7fb-3dab-447b-b4c2-a5840e49457a', 'info': None, 'row': 126, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.57188064070368, 19.677891796227, 0]}}, {'type': 'Feature', 'properties': {'id': '30b4537b-79b2-47cf-be6f-41e5c00d4822', 'info': None, 'row': 126, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.69360353065527, 19.70022629477904, 0]}}, {'type': 'Feature', 'properties': {'id': 'eb6e272a-e559-4545-a4ff-bdf647137bb6', 'info': None, 'row': 126, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.81536005735857, 19.722478327551183, 0]}}, {'type': 'Feature', 'properties': {'id': '5e1200a1-8cda-45fd-949a-91eaf63221b4', 'info': None, 'row': 126, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.9371501469935, 19.744647773214233, 0]}}, {'type': 'Feature', 'properties': {'id': '2576fc9c-0f5b-4750-a1eb-53ee8fb21eb3', 'info': None, 'row': 126, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-69.05897374694116, 19.766734514721847, 0]}}, {'type': 'Feature', 'properties': {'id': 'cad6bb21-4ea1-464c-a6b2-de22b4cecf03', 'info': None, 'row': 126, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.18083081372524, 19.788738437057813, 0]}}, {'type': 'Feature', 'properties': {'id': '8cf2cf5e-1b1a-407e-99a9-4b38a9548d0d', 'info': None, 'row': 126, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.30272129878794, 19.81065942464837, 0]}}, {'type': 'Feature', 'properties': {'id': '1a5f9e2d-3d7a-42ee-b973-8022f3c48836', 'info': None, 'row': 126, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.42464513680491, 19.832497359291384, 0]}}, {'type': 'Feature', 'properties': {'id': '205a099b-e932-43e7-8219-2fd85dfb28cf', 'info': None, 'row': 126, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.54660223943641, 19.854252119105443, 0]}}, {'type': 'Feature', 'properties': {'id': '7d3acfcd-0ac0-4bc6-a9ba-aaff23ccc843', 'info': None, 'row': 126, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.6685924959205, 19.875923578719732, 0]}}, {'type': 'Feature', 'properties': {'id': '21c09ddb-76a8-4cc0-9670-a24773f1ba51', 'info': None, 'row': 126, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.79061578065358, 19.897511610694842, 0]}}, {'type': 'Feature', 'properties': {'id': '80f29937-476c-4cc2-b590-38b647454448', 'info': None, 'row': 126, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.91267196681193, 19.919016087971308, 0]}}, {'type': 'Feature', 'properties': {'id': 'c7ece9b8-3920-41b0-957d-f7aeb62c3168', 'info': None, 'row': 126, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-70.0347609441014, 19.940436886978517, 0]}}, {'type': 'Feature', 'properties': {'id': '4f27ca1a-5270-41f5-904d-2453ab77db0f', 'info': None, 'row': 126, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.15688263784337, 19.961773890894133, 0]}}, {'type': 'Feature', 'properties': {'id': 'e2510d3b-f647-42be-bcef-28e54a17eabc', 'info': None, 'row': 126, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.27903702579206, 19.983026992418964, 0]}}, {'type': 'Feature', 'properties': {'id': 'eb8fa0e0-1912-472e-a6ec-16100ecab139', 'info': None, 'row': 126, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.4012240381884, 20.004196377355772, 0]}}, {'type': 'Feature', 'properties': {'id': '42524517-2da2-4bab-bef9-a5373d22b8f7', 'info': None, 'row': 135, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-68.10225640039053, 19.50669814360092, 0]}}, {'type': 'Feature', 'properties': {'id': 'de7fe0bf-c78f-455e-93cb-f5af0c47b675', 'info': None, 'row': 135, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.22378199652682, 19.529367146661393, 0]}}, {'type': 'Feature', 'properties': {'id': '54c304ad-111d-4101-9d2d-3fe1039777d3', 'info': None, 'row': 135, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.34534138300984, 19.55195454322828, 0]}}, {'type': 'Feature', 'properties': {'id': '41344d2b-d1c3-42ad-aea4-1981328d0a6d', 'info': None, 'row': 135, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.46693451819347, 19.57446021612308, 0]}}, {'type': 'Feature', 'properties': {'id': '2be0eb1b-c9c4-4656-8433-08ff7756fb87', 'info': None, 'row': 135, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.58856129396891, 19.596884036307586, 0]}}, {'type': 'Feature', 'properties': {'id': 'c501f15c-82a5-4ada-af7a-aa4de5be4a70', 'info': None, 'row': 135, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.71022161404049, 19.61922587746659, 0]}}, {'type': 'Feature', 'properties': {'id': '4931a795-ec4c-4f16-8f30-6a2ee0f69f21', 'info': None, 'row': 135, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.83191541580086, 19.641485619955226, 0]}}, {'type': 'Feature', 'properties': {'id': '6b0c1886-d193-43e8-b5a4-06bb99834e86', 'info': None, 'row': 135, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.9536426618795, 19.663663149136834, 0]}}, {'type': 'Feature', 'properties': {'id': '72148f71-9aec-41e9-8031-62b0fd74f47d', 'info': None, 'row': 135, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-69.07540331964162, 19.685758351575064, 0]}}, {'type': 'Feature', 'properties': {'id': '5c04cec6-93b6-4a63-bf8b-c7cfb8d7514f', 'info': None, 'row': 135, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.19719734084178, 19.70777111134324, 0]}}, {'type': 'Feature', 'properties': {'id': '68ef5a68-94c2-492d-8b94-e4e67ce0f1d2', 'info': None, 'row': 135, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.31902464880673, 19.729701307775624, 0]}}, {'type': 'Feature', 'properties': {'id': '259e9967-0d48-497e-af96-1cd017e9d205', 'info': None, 'row': 135, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.44088513659884, 19.751548815240206, 0]}}, {'type': 'Feature', 'properties': {'id': 'c9605ffb-9965-4edb-a328-efeca0c4decf', 'info': None, 'row': 135, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.56277867634189, 19.77331350491002, 0]}}, {'type': 'Feature', 'properties': {'id': 'c6a5b65b-a98c-469e-922a-7264d8a45545', 'info': None, 'row': 135, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.68470513709835, 19.794995248012615, 0]}}, {'type': 'Feature', 'properties': {'id': '37cf2561-c527-4ae1-a038-1741521e95d6', 'info': None, 'row': 135, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.806664406238, 19.816593919619258, 0]}}, {'type': 'Feature', 'properties': {'id': '08ddba24-cf81-4ee3-97ab-44c639b405e3', 'info': None, 'row': 135, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.92865640703532, 19.83810940167702, 0]}}, {'type': 'Feature', 'properties': {'id': 'cb96ceca-f521-4560-b32c-37a6ba50e16e', 'info': None, 'row': 135, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-70.05068110320686, 19.85954158367313, 0]}}, {'type': 'Feature', 'properties': {'id': '28dda739-2608-4dd8-831b-26c4f1b72f1d', 'info': None, 'row': 135, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.17273847919458, 19.880890359042713, 0]}}, {'type': 'Feature', 'properties': {'id': '004e6feb-cfe7-42b5-a886-773d1bfe5eeb', 'info': None, 'row': 135, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.29482848318246, 19.902155615179296, 0]}}, {'type': 'Feature', 'properties': {'id': '43809bd7-ff59-4141-8c7a-8a1abdc0c8b5', 'info': None, 'row': 135, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.41695081080414, 19.923337495883352, 0]}}, {'type': 'Feature', 'properties': {'id': 'a12fc9fd-6a41-4a5e-87b0-c7a628de4592', 'info': None, 'row': 144, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-68.11918116030074, 19.425663587124642, 0]}}, {'type': 'Feature', 'properties': {'id': 'f4480013-8080-408c-b152-3f61c58f6f17', 'info': None, 'row': 144, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.24064507834863, 19.44833848014618, 0]}}, {'type': 'Feature', 'properties': {'id': '66ec4275-4f0f-4fa0-ac42-057b0f489e3d', 'info': None, 'row': 144, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.36214269916091, 19.47093214566042, 0]}}, {'type': 'Feature', 'properties': {'id': '48825c45-acce-42b5-b7af-5b445229dcf1', 'info': None, 'row': 144, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.48367388390415, 19.49344444845072, 0]}}, {'type': 'Feature', 'properties': {'id': '3979c245-5e49-4c5e-95bc-c8b1a8155981', 'info': None, 'row': 144, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.60523852763585, 19.515875260197834, 0]}}, {'type': 'Feature', 'properties': {'id': 'cc1deacc-f939-465b-91c1-894af566bdf3', 'info': None, 'row': 144, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.72683656656174, 19.538224460673245, 0]}}, {'type': 'Feature', 'properties': {'id': '867903d0-114e-44c5-aabe-64d638cffd58', 'info': None, 'row': 144, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.8484679639933, 19.560491935019005, 0]}}, {'type': 'Feature', 'properties': {'id': 'b7904510-1ab9-489f-97b5-626bd84a4a2c', 'info': None, 'row': 144, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.97013268885739, 19.58267756973788, 0]}}, {'type': 'Feature', 'properties': {'id': '11437d44-693e-4201-8285-a6706672f8d0', 'info': None, 'row': 144, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-69.09183069593865, 19.604781249092735, 0]}}, {'type': 'Feature', 'properties': {'id': 'f28c7016-ed31-479e-a23d-3fea9dea1541', 'info': None, 'row': 144, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.21356191336196, 19.62680285289886, 0]}}, {'type': 'Feature', 'properties': {'id': '182d9a49-34c0-4563-9ea2-c47fe21a6f0f', 'info': None, 'row': 144, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.33532623986687, 19.648742256130237, 0]}}, {'type': 'Feature', 'properties': {'id': 'e62b0178-cc3e-4170-9947-f1b7b31d904a', 'info': None, 'row': 144, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.45712355198701, 19.670599330310054, 0]}}, {'type': 'Feature', 'properties': {'id': 'c8ba285f-9b73-4b3f-aacf-1ce989949129', 'info': None, 'row': 144, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.57895371918035, 19.69237394628655, 0]}}, {'type': 'Feature', 'properties': {'id': '4677e449-6074-4daa-8b84-b0112e43622e', 'info': None, 'row': 144, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.70081662315565, 19.71406597768767, 0]}}, {'type': 'Feature', 'properties': {'id': '95fdec17-cd98-4743-b5c8-404da7051aa7', 'info': None, 'row': 144, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.82271217603095, 19.73567530408487, 0]}}, {'type': 'Feature', 'properties': {'id': 'd0ed6908-23ec-4aba-889d-aa094cbde4e8', 'info': None, 'row': 144, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.94464033048204, 19.75720181266818, 0]}}, {'type': 'Feature', 'properties': {'id': '1ff8bba7-ab17-495c-909a-6660babda933', 'info': None, 'row': 144, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-70.0666010736564, 19.778645397031976, 0]}}, {'type': 'Feature', 'properties': {'id': '9cc8f90b-0cf2-4b63-896e-476997704183', 'info': None, 'row': 144, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.18859439530554, 19.800005951488476, 0]}}, {'type': 'Feature', 'properties': {'id': '962b12fb-5ed7-440c-a6f9-7e21ef2d4b17', 'info': None, 'row': 144, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.31062021930886, 19.821283359159114, 0]}}, {'type': 'Feature', 'properties': {'id': 'ca818ca9-c3fd-43a4-8da5-c516644d10fc', 'info': None, 'row': 144, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.43267818020384, 19.8424777519894, 0]}}, {'type': 'Feature', 'properties': {'id': '149eb996-7bfa-42a2-8175-6013f24c2049', 'info': None, 'row': 153, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-68.13609896278187, 19.34462736726083, 0]}}, {'type': 'Feature', 'properties': {'id': '56982216-1d43-412b-a5a3-d618cf450cf9', 'info': None, 'row': 153, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.2575016683071, 19.367308181375734, 0]}}, {'type': 'Feature', 'properties': {'id': 'cef54201-b148-4bf5-b4ad-309da6ed08af', 'info': None, 'row': 153, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.37893792210015, 19.389908133922248, 0]}}, {'type': 'Feature', 'properties': {'id': '3ac23319-e5d0-4af3-98bd-de0e203a8d6f', 'info': None, 'row': 153, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.50040751404437, 19.412427076536662, 0]}}, {'type': 'Feature', 'properties': {'id': 'b9e4cd78-5cd3-4d4d-afef-f2111fbdb8e1', 'info': None, 'row': 153, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.6219104127003, 19.43486489466217, 0]}}, {'type': 'Feature', 'properties': {'id': '10c315bc-c781-4bc2-b402-1590ea129225', 'info': None, 'row': 153, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.7434466220906, 19.457221480589265, 0]}}, {'type': 'Feature', 'properties': {'id': '3c993239-9fa4-4fd5-8f47-d050c9c2775d', 'info': None, 'row': 153, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.86501611666665, 19.47949672142235, 0]}}, {'type': 'Feature', 'properties': {'id': 'dce7fbd0-87c0-429b-a70d-0ca7b63aeb4f', 'info': None, 'row': 153, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-68.98661882449186, 19.501690496118023, 0]}}, {'type': 'Feature', 'properties': {'id': '7e181175-fb1a-4844-96dc-6e69d4d13845', 'info': None, 'row': 153, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-69.10825463683545, 19.523802677393263, 0]}}, {'type': 'Feature', 'properties': {'id': 'a4b1d860-3992-4b74-9247-e8c4147f8653', 'info': None, 'row': 153, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.2299234286929, 19.545833135569364, 0]}}, {'type': 'Feature', 'properties': {'id': 'cd903a10-a9f6-46a3-8e57-eeaf63403c62', 'info': None, 'row': 153, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.35162507973524, 19.56778174240457, 0]}}, {'type': 'Feature', 'properties': {'id': '5ffcd6f8-1603-4291-b620-4f72eea9fee5', 'info': None, 'row': 153, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.4733594892021, 19.58964837374869, 0]}}, {'type': 'Feature', 'properties': {'id': 'f747de6d-4007-4406-9fc5-ef2e0a086a4a', 'info': None, 'row': 153, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.59512658155495, 19.611432910480737, 0]}}, {'type': 'Feature', 'properties': {'id': '76c65cfb-868b-4e80-9e73-1ad52e4ddb4a', 'info': None, 'row': 153, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.7169263024859, 19.63313523770408, 0]}}, {'type': 'Feature', 'properties': {'id': '6a03794b-bfc6-4f9c-80a1-238a9bb1e6b5', 'info': None, 'row': 153, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.8387586072783, 19.654755242602086, 0]}}, {'type': 'Feature', 'properties': {'id': '25387665-d8c6-4b6a-afc4-c7a2a5123c39', 'info': None, 'row': 153, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.96062344563707, 19.676292811721176, 0]}}, {'type': 'Feature', 'properties': {'id': '530820e6-ee99-4083-9e15-8eaedd7e3686', 'info': None, 'row': 153, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-70.08252074902873, 19.697747828763077, 0]}}, {'type': 'Feature', 'properties': {'id': 'd6ae9c16-4fc2-4735-ba1f-d68d84de9691', 'info': None, 'row': 153, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.20445042834844, 19.719120174245212, 0]}}, {'type': 'Feature', 'properties': {'id': 'a3937dc7-f2b2-4a04-bf11-583701afb604', 'info': None, 'row': 153, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.32641239140695, 19.74040972863606, 0]}}, {'type': 'Feature', 'properties': {'id': '249afcb3-76b6-4094-9c7a-b24881ccb710', 'info': None, 'row': 153, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.4484064830348, 19.76161665917973, 0]}}, {'type': 'Feature', 'properties': {'id': 'cabac2d0-f9a3-49d9-94bd-fd794c45142c', 'info': None, 'row': 162, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [-68.15301560536376, 19.26359089282891, 0]}}, {'type': 'Feature', 'properties': {'id': 'd3eb12f0-ba36-4379-99fa-131b654b5a5b', 'info': None, 'row': 162, 'col': 13}, 'geometry': {'type': 'Point', 'coordinates': [-68.27435721598496, 19.28627764161197, 0]}}, {'type': 'Feature', 'properties': {'id': '48be7f14-f76a-469a-abc3-54f40d52e293', 'info': None, 'row': 162, 'col': 26}, 'geometry': {'type': 'Point', 'coordinates': [-68.39573213167108, 19.30888387809661, 0]}}, {'type': 'Feature', 'properties': {'id': '19b4c337-6bfd-4fe0-ac2b-724781f164f0', 'info': None, 'row': 162, 'col': 39}, 'geometry': {'type': 'Point', 'coordinates': [-68.51714019839093, 19.331409464558536, 0]}}, {'type': 'Feature', 'properties': {'id': 'a85a54fd-1ae0-46a5-8ac8-d03ee0e292dd', 'info': None, 'row': 162, 'col': 52}, 'geometry': {'type': 'Point', 'coordinates': [-68.63858145719851, 19.353854299942657, 0]}}, {'type': 'Feature', 'properties': {'id': 'dcdda188-387c-454a-b5e8-5d1eb62a1ba6', 'info': None, 'row': 162, 'col': 65}, 'geometry': {'type': 'Point', 'coordinates': [-68.76005594414586, 19.37621828240422, 0]}}, {'type': 'Feature', 'properties': {'id': 'f9ac4edb-0a0d-4d65-92fe-a9f796d43af3', 'info': None, 'row': 162, 'col': 78}, 'geometry': {'type': 'Point', 'coordinates': [-68.88156361777911, 19.39850129605297, 0]}}, {'type': 'Feature', 'properties': {'id': 'f8145d23-fc5f-44b3-b9e8-5558de82e7c4', 'info': None, 'row': 162, 'col': 91}, 'geometry': {'type': 'Point', 'coordinates': [-69.00310435984242, 19.420703211353324, 0]}}, {'type': 'Feature', 'properties': {'id': 'bf066ba1-26b2-49c1-aea4-8a4ae0a92ce7', 'info': None, 'row': 162, 'col': 104}, 'geometry': {'type': 'Point', 'coordinates': [-69.12467801043027, 19.44282389177818, 0]}}, {'type': 'Feature', 'properties': {'id': '012226b5-d70a-4a79-acb3-51576fa53523', 'info': None, 'row': 162, 'col': 117}, 'geometry': {'type': 'Point', 'coordinates': [-69.2462844109842, 19.464863201732403, 0]}}, {'type': 'Feature', 'properties': {'id': 'cc98c4a4-9e93-4e34-a935-09ded9d4159f', 'info': None, 'row': 162, 'col': 130}, 'geometry': {'type': 'Point', 'coordinates': [-69.36792343817507, 19.486821012651415, 0]}}, {'type': 'Feature', 'properties': {'id': '17990339-b275-4f7d-90a7-743957ecaf26', 'info': None, 'row': 162, 'col': 143}, 'geometry': {'type': 'Point', 'coordinates': [-69.48959501953459, 19.508697205680427, 0]}}, {'type': 'Feature', 'properties': {'id': '5eb9a832-3faf-40d0-a338-749328d0ab72', 'info': None, 'row': 162, 'col': 156}, 'geometry': {'type': 'Point', 'coordinates': [-69.6112991281951, 19.530491670553143, 0]}}, {'type': 'Feature', 'properties': {'id': '3c42996f-7b60-42c0-b1fd-ac03ef588468', 'info': None, 'row': 162, 'col': 169}, 'geometry': {'type': 'Point', 'coordinates': [-69.73303575962427, 19.55220430128794, 0]}}, {'type': 'Feature', 'properties': {'id': 'bc4fb94d-cf99-44ae-8866-ffa41a381b21', 'info': None, 'row': 162, 'col': 182}, 'geometry': {'type': 'Point', 'coordinates': [-69.85480489807003, 19.573834990158893, 0]}}, {'type': 'Feature', 'properties': {'id': 'c46bbcef-9e01-41de-86eb-0e5f1ee90e29', 'info': None, 'row': 162, 'col': 195}, 'geometry': {'type': 'Point', 'coordinates': [-69.97660648475095, 19.59538362211795, 0]}}, {'type': 'Feature', 'properties': {'id': 'fdd36f6f-2248-49c9-b1cd-99eb366f96f5', 'info': None, 'row': 162, 'col': 208}, 'geometry': {'type': 'Point', 'coordinates': [-70.09844040378555, 19.616850072470932, 0]}}, {'type': 'Feature', 'properties': {'id': '7098fef1-7471-4a49-b62b-1d4ff64642df', 'info': None, 'row': 162, 'col': 221}, 'geometry': {'type': 'Point', 'coordinates': [-70.22030650555465, 19.638234211166385, 0]}}, {'type': 'Feature', 'properties': {'id': 'b9c54d92-9287-4b9f-84e6-e25d6259f2fb', 'info': None, 'row': 162, 'col': 234}, 'geometry': {'type': 'Point', 'coordinates': [-70.3422046907136, 19.65953591755677, 0]}}, {'type': 'Feature', 'properties': {'id': '6473e0c2-097d-44f2-a888-b7baaaedb626', 'info': None, 'row': 162, 'col': 247}, 'geometry': {'type': 'Point', 'coordinates': [-70.46413497172658, 19.680755386761145, 0]}}]}
array(0)
- longitude(line, sample)float64dask.array<chunksize=(167, 251), meta=np.ndarray>
- history :
- longitude: annotation/s1a.xml: - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/line - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/pixel - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/longitude
Array Chunk Bytes 327.48 kiB 327.48 kiB Shape (167, 251) (167, 251) Dask graph 1 chunks in 4 graph layers Data type float64 numpy.ndarray - latitude(line, sample)float64dask.array<chunksize=(167, 251), meta=np.ndarray>
- history :
- latitude: annotation/s1a.xml: - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/line - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/pixel - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/latitude
Array Chunk Bytes 327.48 kiB 327.48 kiB Shape (167, 251) (167, 251) Dask graph 1 chunks in 4 graph layers Data type float64 numpy.ndarray - sigma0(pol, line, sample)float64dask.array<chunksize=(1, 167, 251), meta=np.ndarray>
- history :
- sigma0: nesz: noise_lut: noise_lut_azi_raw_grd: annotation/calibration/noise.xml: - /noise/noiseAzimuthVectorList/noiseAzimuthVector/line - /noise/noiseAzimuthVectorList/noiseAzimuthVector/firstAzimuthLine - /noise/noiseAzimuthVectorList/noiseAzimuthVector/lastAzimuthLine - /noise/noiseAzimuthVectorList/noiseAzimuthVector/firstRangeSample - /noise/noiseAzimuthVectorList/noiseAzimuthVector/lastRangeSample - /noise/noiseAzimuthVectorList/noiseAzimuthVector/noiseAzimuthLut - /noise/noiseAzimuthVectorList/noiseAzimuthVector/swath noise_lut_range_raw: annotation/calibration/noise.xml: - /noise/noiseRangeVectorList/noiseRangeVector/line | /noise/noiseVectorList/noiseVector/line - /noise/noiseRangeVectorList/noiseRangeVector/pixel | /noise/noiseVectorList/noiseVector/pixel - /noise/noiseRangeVectorList/noiseRangeVector/noiseRangeLut | /noise/noiseVectorList/noiseVector/noiseLut - /noise/noiseRangeVectorList/noiseRangeVector/azimuthTime sigma0_lut: annotation/calibration/calibration.xml: //calibration/calibrationVectorList/calibrationVector/sigmaNought sigma0_raw: sigma0_lut: annotation/calibration/calibration.xml: //calibration/calibrationVectorList/calibrationVector/sigmaNought
- comment_recalibration :
- kersten recalibration not applied
- comment :
- not clipped, some values can be <0
Array Chunk Bytes 654.95 kiB 327.48 kiB Shape (2, 167, 251) (1, 167, 251) Dask graph 2 chunks in 44 graph layers Data type float64 numpy.ndarray - incidence(line, sample)float64dask.array<chunksize=(167, 251), meta=np.ndarray>
- history :
- incidenceAngle: annotation/s1a.xml: - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/line - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/pixel - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/incidenceAngle
Array Chunk Bytes 327.48 kiB 327.48 kiB Shape (167, 251) (167, 251) Dask graph 1 chunks in 4 graph layers Data type float64 numpy.ndarray
- polPandasIndex
PandasIndex(Index(['VV', 'VH'], dtype='object', name='pol'))
- linePandasIndex
PandasIndex(Float64Index([ 49.5, 149.5, 249.5, 349.5, 449.5, 549.5, 649.5, 749.5, 849.5, 949.5, ... 15749.5, 15849.5, 15949.5, 16049.5, 16149.5, 16249.5, 16349.5, 16449.5, 16549.5, 16649.5], dtype='float64', name='line', length=167))
- samplePandasIndex
PandasIndex(Float64Index([ 49.5, 149.5, 249.5, 349.5, 449.5, 549.5, 649.5, 749.5, 849.5, 949.5, ... 24149.5, 24249.5, 24349.5, 24449.5, 24549.5, 24649.5, 24749.5, 24849.5, 24949.5, 25049.5], dtype='float64', name='sample', length=251))
- name :
- SENTINEL1_DS:/tmp/S1A_IW_GRDH_1SDV_20170907T103020_20170907T103045_018268_01EB76_Z010.SAFE:IW
- short_name :
- SENTINEL1_DS:S1A_IW_GRDH_1SDV_20170907T103020_20170907T103045_018268_01EB76_Z010.SAFE:IW
- product :
- GRDH
- safe :
- S1A_IW_GRDH_1SDV_20170907T103020_20170907T103045_018268_01EB76_Z010.SAFE
- swath :
- IW
- multidataset :
- False
- ipf :
- 2.84
- platform :
- SENTINEL-1A
- pols :
- VV VH
- start_date :
- 2017-09-07 10:30:20.936409
- stop_date :
- 2017-09-07 10:30:45.935264
- footprint :
- POLYGON ((-67.84221143971432 20.72564283093837, -70.22162571215458 21.14758369556577, -70.51434171237564 19.64044158236074, -68.15836401927538 19.21519321274318, -67.84221143971432 20.72564283093837))
- coverage :
- 170km * 251km (line * sample )
- orbit_pass :
- Descending
- platform_heading :
- -167.7668824808032
Sigma0 detrending with xsarsea
Sigma0 detrending is done by xsarsea.sigma0_detrend function
As the resulting xarray dataset have the same coordinates as the original sigma0, we can add a sigma0_detrend
variable to the dataset.
By default, analytical models are available. We load all luts : replace paths by your own path containing all luts
[6]:
nc_luts_path = xsarsea.get_test_file('nc_luts_reduce')
path_cmod7 = xsarsea.get_test_file("cmod7_and_python_script")
xsarsea.windspeed.register_luts(nc_luts_path, path_cmod7)
xsarsea.windspeed.available_models()
DEBUG:xsarsea.windspeed:register model nc_lut_gmf_cmod5n_Rlow_hh_mouche1 pol=HH units=linear
inc_range=[17.0, 50.0] wspd_range=[0.2, 50.0] phi_range=[0.0, 180.0]
inc_step=0.1 wspd_step=0.1 phi_step=1.0
inc_step_lr=1.0 wspd_step_lr=0.2 phi_step_lr=2.5
DEBUG:xsarsea.windspeed:register model nc_lut_cmodms1ahw pol=VH units=dB
inc_range=[17.0, 50.0] wspd_range=[3.0, 80.0] phi_range=None
inc_step=0.1 wspd_step=0.1 phi_step=1.0
inc_step_lr=1.0 wspd_step_lr=0.2 phi_step_lr=2.5
DEBUG:xsarsea.windspeed:register model nc_lut_gmf_cmod5n_Rlow_hh_zhangB pol=HH units=linear
inc_range=[17.0, 50.0] wspd_range=[0.2, 50.0] phi_range=[0.0, 180.0]
inc_step=0.1 wspd_step=0.1 phi_step=1.0
inc_step_lr=1.0 wspd_step_lr=0.2 phi_step_lr=2.5
DEBUG:xsarsea.windspeed:register model nc_lut_gmf_cmod5n_Rlow_hh_zhangA pol=HH units=linear
inc_range=[17.0, 50.0] wspd_range=[0.2, 50.0] phi_range=[0.0, 180.0]
inc_step=0.1 wspd_step=0.1 phi_step=1.0
inc_step_lr=1.0 wspd_step_lr=0.2 phi_step_lr=2.5
DEBUG:xsarsea.windspeed:register model nc_lut_gmf_cmod7_Rlow_hh_zhangB pol=HH units=linear
inc_range=[16.0, 66.0] wspd_range=[0.2, 50.0] phi_range=[0.0, 180.0]
inc_step=0.1 wspd_step=0.1 phi_step=1.0
inc_step_lr=1 wspd_step_lr=0.2 phi_step_lr=2.5
DEBUG:xsarsea.windspeed:register model nc_lut_gmf_cmod7_Rlow_hh_zhangA pol=HH units=linear
inc_range=[16.0, 66.0] wspd_range=[0.2, 50.0] phi_range=[0.0, 180.0]
inc_step=0.1 wspd_step=0.1 phi_step=1.0
inc_step_lr=1 wspd_step_lr=0.2 phi_step_lr=2.5
DEBUG:xsarsea.windspeed:register model nc_lut_gmf_cmod7_Rlow_hh_mouche1 pol=HH units=linear
inc_range=[16.0, 66.0] wspd_range=[0.2, 50.0] phi_range=[0.0, 180.0]
inc_step=0.1 wspd_step=0.1 phi_step=1.0
inc_step_lr=1 wspd_step_lr=0.2 phi_step_lr=2.5
DEBUG:xsarsea.windspeed:register model gmf_cmod7 pol=VV units=None
inc_range=[16.0, 66.0] wspd_range=None phi_range=None
inc_step=0.1 wspd_step=0.1 phi_step=1.0
inc_step_lr=1.0 wspd_step_lr=0.2 phi_step_lr=2.5
[6]:
alias | pol | model | |
---|---|---|---|
gmf_cmod7 | cmod7 | VV | <Cmod7Model('gmf_cmod7') pol=VV> |
gmf_cmod5 | cmod5 | VV | <GmfModel('gmf_cmod5') pol=VV> |
gmf_cmod5n | cmod5n | VV | <GmfModel('gmf_cmod5n') pol=VV> |
gmf_cmodifr2 | cmodifr2 | VV | <GmfModel('gmf_cmodifr2') pol=VV> |
gmf_rs2_v2 | rs2_v2 | VH | <GmfModel('gmf_rs2_v2') pol=VH> |
gmf_s1_v2 | s1_v2 | VH | <GmfModel('gmf_s1_v2') pol=VH> |
gmf_rcm_noaa | rcm_noaa | VH | <GmfModel('gmf_rcm_noaa') pol=VH> |
nc_lut_gmf_cmod5n_Rlow_hh_mouche1 | cmod5n_Rlow_hh_mouche1 | HH | <NcLutModel('nc_lut_gmf_cmod5n_Rlow_hh_mouche1... |
nc_lut_cmodms1ahw | cmodms1ahw | VH | <NcLutModel('nc_lut_cmodms1ahw') pol=VH> |
nc_lut_gmf_cmod5n_Rlow_hh_zhangB | cmod5n_Rlow_hh_zhangB | HH | <NcLutModel('nc_lut_gmf_cmod5n_Rlow_hh_zhangB'... |
nc_lut_gmf_cmod5n_Rlow_hh_zhangA | cmod5n_Rlow_hh_zhangA | HH | <NcLutModel('nc_lut_gmf_cmod5n_Rlow_hh_zhangA'... |
nc_lut_gmf_cmod7_Rlow_hh_zhangB | cmod7_Rlow_hh_zhangB | HH | <NcLutModel('nc_lut_gmf_cmod7_Rlow_hh_zhangB')... |
nc_lut_gmf_cmod7_Rlow_hh_zhangA | cmod7_Rlow_hh_zhangA | HH | <NcLutModel('nc_lut_gmf_cmod7_Rlow_hh_zhangA')... |
nc_lut_gmf_cmod7_Rlow_hh_mouche1 | cmod7_Rlow_hh_mouche1 | HH | <NcLutModel('nc_lut_gmf_cmod7_Rlow_hh_mouche1'... |
default detrend with gmf_cmod5n
[7]:
sar_ds['sigma0'] = sar_ds.sigma0
sar_ds['sigma0_detrend'] = xsarsea.sigma0_detrend(sar_ds.sigma0, sar_ds.incidence)
DEBUG:xsarsea.windspeed:timing _gmf_function : 0.92s. mem: +13.9Mb
INFO:xsarsea:timing sigma0_detrend : 0.95s. mem: +15.2Mb
[8]:
original_sigma0_plot = hv.Image(sar_ds.sigma0.sel(pol='VV')).opts(
title="Original Sigma0",
cmap='gray',
clim=(0, 0.4),
colorbar=True,
width=400,
height=400,
tools=['hover']
)
detrended_sigma0_plot = hv.Image(sar_ds.sigma0_detrend.isel(pol=0)).opts(
title="Detrended Sigma0",
cmap='gray',
clim=(0, 0.4),
colorbar=True,
width=400,
height=400,
tools=['hover']
)
(original_sigma0_plot + detrended_sigma0_plot).cols(2)
/home/vincelhx/miniconda3/envs/xsar_N3_local/lib/python3.10/site-packages/holoviews/plotting/bokeh/plot.py:987: UserWarning: found multiple competing values for 'toolbar.active_drag' property; using the latest value
layout_plot = gridplot(
/home/vincelhx/miniconda3/envs/xsar_N3_local/lib/python3.10/site-packages/holoviews/plotting/bokeh/plot.py:987: UserWarning: found multiple competing values for 'toolbar.active_scroll' property; using the latest value
layout_plot = gridplot(
[8]:
custom detrend
very small differences
[9]:
detrend_vv_gmf_cmod7 = xsarsea.sigma0_detrend(sar_ds.sigma0.sel(pol='VV'), sar_ds.incidence, model='gmf_cmod7')
detrend_vv_gmf_cmod5 = xsarsea.sigma0_detrend(sar_ds.sigma0.sel(pol='VV'), sar_ds.incidence, model='gmf_cmod5')
detrend_vv_gmf_cmod5n = xsarsea.sigma0_detrend(sar_ds.sigma0.sel(pol='VV'), sar_ds.incidence, model='gmf_cmod5n')
## HH GMF
detrend_vv_gmf_cmod5n_zhangA = xsarsea.sigma0_detrend(sar_ds.sigma0.sel(pol='VV'), sar_ds.incidence, model='cmod5n_Rlow_hh_zhangA')
INFO:xsarsea.windspeed:load gmf lut from /tmp/cmod7_and_python_script
DEBUG:xsarsea.windspeed:lut_resolution gmf_cmod7 from _raw_lut : low
DEBUG:xsarsea.windspeed:desired gmf_cmod7 resolution : high
DEBUG:xsarsea.windspeed:interp lut gmf_cmod7 to high res
INFO:xsarsea:timing sigma0_detrend : 1.04s. mem: +6.5Mb
DEBUG:xsarsea.windspeed:timing _gmf_function : 0.66s. mem: +4.5Mb
INFO:xsarsea:timing sigma0_detrend : 0.69s. mem: +4.6Mb
DEBUG:xsarsea.windspeed:timing _gmf_function : 0.00s. mem: +0.0Mb
INFO:xsarsea:timing sigma0_detrend : 0.02s. mem: +0.2Mb
DEBUG:xsarsea.windspeed:lut_resolution nc_lut_gmf_cmod5n_Rlow_hh_zhangA from _raw_lut : low
DEBUG:xsarsea.windspeed:desired nc_lut_gmf_cmod5n_Rlow_hh_zhangA resolution : high
DEBUG:xsarsea.windspeed:interp lut nc_lut_gmf_cmod5n_Rlow_hh_zhangA to high res
INFO:xsarsea:timing sigma0_detrend : 0.68s. mem: +2.7Mb
[10]:
import matplotlib.pyplot as plt
from mpl_toolkits.axes_grid1 import make_axes_locatable
import numpy as np
fig, axs = plt.subplots(1, 4, figsize=(20, 5))
datasets = [detrend_vv_gmf_cmod7, detrend_vv_gmf_cmod5, detrend_vv_gmf_cmod5n, detrend_vv_gmf_cmod5n_zhangA]
titles = ['GMF CMOD7', 'GMF CMOD5', 'GMF CMOD5N', 'GMF CMOD5N ZhangA']
for ax, data, title in zip(axs, datasets, titles):
img = ax.pcolormesh(sar_ds.longitude, sar_ds.latitude, data, cmap='gray', vmin=0, vmax=0.4)
ax.set_title(title)
ax.set_xlabel("longitude")
ax.set_ylabel("latitude")
divider = make_axes_locatable(ax)
cax = divider.append_axes("right", size="5%", pad=0.05)
plt.colorbar(img, cax=cax)
# Improve spacing between plots
plt.tight_layout()
plt.show()
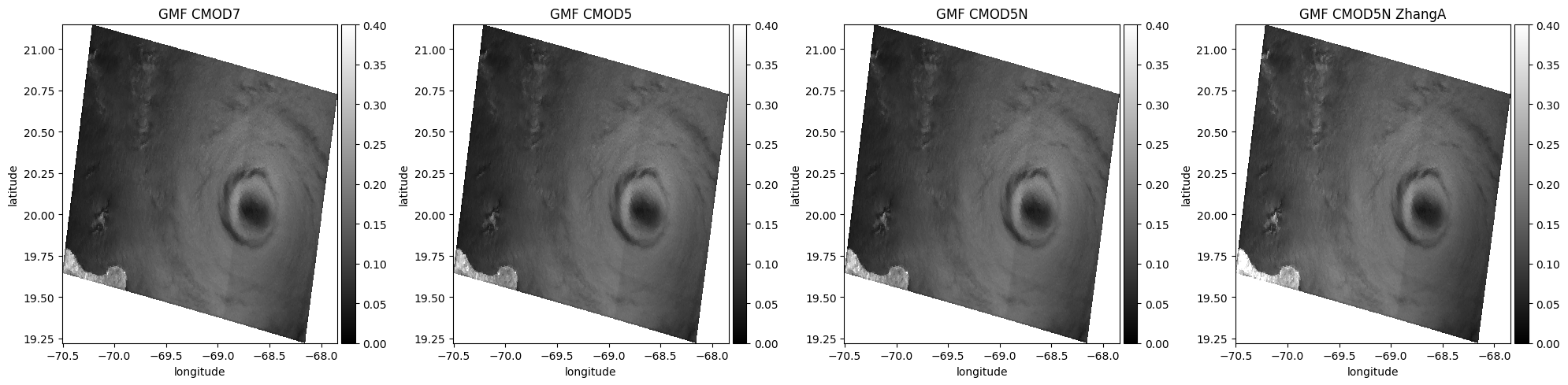
[ ]: