Download this notebook from github.
Wind speed inversion from level-1 product
Warning
Use of ancillary wind
On this notebook, we changed from
ancillary_wind = -np.conj(sarwing_ds.owi_ancillary_wind)
tosarwing_ds.owi_ancillary_wind
; then it won’t match sarwing results anymore
[1]:
import xsarsea
from xsarsea import windspeed
import xarray as xr
import numpy as np
import holoviews as hv
hv.extension('bokeh')
import os,sys,re, cv2
[2]:
# optional debug messages
#import logging
#logging.basicConfig()
#logging.getLogger('xsarsea.windspeed').setLevel(logging.DEBUG) # or .setLevel(logging.INFO)
Requirements for inversion
[3]:
import xsar
Getting metadata
[4]:
safe_path = xsarsea.get_test_file("S1A_EW_GRDM_1SDV_20230217T002336_20230217T002412_047268_05AC30_Z005.SAFE")
s1meta = xsar.Sentinel1Meta(safe_path)
[5]:
safe_path
[5]:
'/tmp/S1A_EW_GRDM_1SDV_20230217T002336_20230217T002412_047268_05AC30_Z005.SAFE'
[6]:
from xsarsea.utils import _load_config
config = _load_config()
land mask: not applied in the doc
getting ancillary data
[7]:
s1meta.set_raster('ecmwf_0100_1h',config["path_ecmwf_0100_1h"])
import datetime
for ecmwf_name in ['ecmwf_0100_1h' ]:
ecmwf_infos = s1meta.rasters.loc[ecmwf_name]
ecmwf_file = ecmwf_infos['get_function'](ecmwf_infos['resource'], date=datetime.datetime.strptime(s1meta.start_date, '%Y-%m-%d %H:%M:%S.%f'))
ecmwf_file = xsarsea.get_test_file(ecmwf_file[1].split('/')[-1],iszip=False)
map_model = { '%s_%s' % (ecmwf_name, uv) : 'model_%s' % uv for uv in ['U10', 'V10'] }
[8]:
map_model
[8]:
{'ecmwf_0100_1h_U10': 'model_U10', 'ecmwf_0100_1h_V10': 'model_V10'}
[9]:
s1meta.rasters.at["ecmwf_0100_1h","resource"] = ecmwf_file
Mapping model & adding ancillary wind
[10]:
### Loading dataset & merging ancillary
xsar_obj = xsar.Sentinel1Dataset(s1meta, resolution='1000m')
[11]:
dataset = xsar_obj.datatree['measurement'].to_dataset()
dataset = dataset.rename(map_model)
## variables of interest
creation of variables of interest for inversion here we could add a land/ice mask.
[12]:
### Variables of interest
#xsar_obj.dataset['land_mask'].values = cv2.dilate(xsar_obj.dataset['land_mask'].values.astype('uint8'),np.ones((3,3),np.uint8),iterations = 3)
#xsar_obj.dataset['sigma0_ocean'] = xr.where(xsar_obj.dataset['land_mask'], np.nan, xsar_obj.dataset['sigma0'].compute()).transpose(*xsar_obj.dataset['sigma0'].dims)
#xsar_obj.dataset['sigma0_ocean'] = xr.where(xsar_obj.dataset['sigma0_ocean'] <= 0, 1e-15, xsar_obj.dataset['sigma0_ocean'])
[13]:
dataset['sigma0_ocean'] = xr.where(dataset['sigma0'] <= 0, 1e-15, xsar_obj.dataset['sigma0'])
dataset['ancillary_wind_direction'] = (90. - np.rad2deg(np.arctan2(dataset.model_V10, dataset.model_U10)) + 180) % 360
dataset['ancillary_wind_speed'] = np.sqrt(dataset['model_U10']**2+dataset['model_V10']**2)
dataset['ancillary_wind'] = dataset.ancillary_wind_speed * np.exp(1j * xsarsea.dir_meteo_to_sample(dataset.ancillary_wind_direction, dataset.ground_heading)) # ref antenna
[14]:
hv.Image(dataset['sigma0_ocean'].sel(pol='VH')).opts(colorbar=True,cmap='binary',width=425, height=400, tools = ['hover'], title = "sigma0 VH")
[14]:
Inversion
inversion parameters
[15]:
apply_flattening = True
GMF_VV_NAME = "gmf_cmod5n"
GMF_VH_NAME = "gmf_s1_v2"
apply flattening or not
[16]:
nesz_cr = dataset.nesz.isel(pol=1) #(no_flattening)
if apply_flattening :
dataset=dataset.assign(nesz_VH_final=(['line','sample'],windspeed.nesz_flattening(nesz_cr, dataset.incidence)))
dataset['nesz_VH_final'].attrs["comment"] = 'nesz has been flattened using windspeed.nesz_flattening'
else :
dataset=dataset.assign(nesz_VH_final=(['line','sample'],nesz_cr.values))
dataset['nesz_VH_final'].attrs["comment"] = 'nesz has not been flattened'
compute dsig_cr (mix between polarisations) using the last version : “gmf_s1_v2”
[17]:
dsig_cr = windspeed.get_dsig("gmf_s1_v2", dataset.incidence,dataset.sigma0_ocean.sel(pol='VH'),dataset.nesz_VH_final)
retrieve windspeed & direction in dfferent polarizations
define kwargs to parameter LUT resolution
[18]:
kwargs = {"wspd_step" : 0.1, "inc_step" : 0.1, "phi_step" : 1.0, "resolution": "high"}
copol and dual polarization
[19]:
wind_co, wind_dual = windspeed.invert_from_model(
dataset.incidence,
dataset.sigma0_ocean.isel(pol=0),
dataset.sigma0_ocean.isel(pol=1),
ancillary_wind=dataset['ancillary_wind'],
dsig_cr = dsig_cr,
model=(GMF_VV_NAME,GMF_VH_NAME),
** kwargs)
dataset["windspeed_co"] = np.abs(wind_co)
dataset["windspeed_dual"] = np.abs(wind_dual)
dataset['winddir_co'] = (90 - np.angle(wind_co, deg=True) + dataset.ground_heading) % 360
dataset['winddir_dual'] = (90 - np.angle(wind_dual, deg=True) + dataset.ground_heading) % 360
cross polarization
[20]:
windspeed_cr = windspeed.invert_from_model(
dataset.incidence.values,
dataset.sigma0_ocean.isel(pol=1).values,
dsig_cr = dsig_cr.values,
model=GMF_VH_NAME,
** kwargs)
dataset = dataset.assign(
windspeed_cross=(['line', 'sample'], windspeed_cr))
/home/vincelhx/Documents/autoentreprise/IFREMER/libs/fork_xsarsea_vinc/xsarsea/src/xsarsea/windspeed/windspeed.py:80: UserWarning: Unable to check sigma0 pol. Assuming VH
warnings.warn(
[21]:
windspeed_co = dataset.windspeed_co.compute() # compute data if needed
windspeed_cross = dataset.windspeed_cross
windspeed_dual = dataset.windspeed_dual.compute()
windspeeed illustration
[22]:
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
import cartopy.feature as cfeature
projection = ccrs.PlateCarree()
fig, axs = plt.subplots(1, 3, figsize=(18, 6), subplot_kw={'projection': projection})
# Wind Speed Co-Pol
img0 = axs[0].pcolormesh(dataset.longitude, dataset.latitude, windspeed_co, cmap='jet', vmin=0, vmax=80, transform=ccrs.PlateCarree())
axs[0].add_feature(cfeature.COASTLINE)
axs[0].add_feature(cfeature.BORDERS, linestyle=':')
axs[0].set_title('Wind Speed Co-Pol')
gl0 = axs[0].gridlines(draw_labels=True, color='gray', alpha=0.5, linestyle='--')
gl0.top_labels = gl0.right_labels = False
# Wind Speed Cr-Pol
img1 = axs[1].pcolormesh(dataset.longitude, dataset.latitude, windspeed_cross, cmap='jet', vmin=0, vmax=80, transform=ccrs.PlateCarree())
axs[1].add_feature(cfeature.COASTLINE)
axs[1].add_feature(cfeature.BORDERS, linestyle=':')
axs[1].set_title('Wind Speed Cr-Pol')
gl1 = axs[1].gridlines(draw_labels=True, color='gray', alpha=0.5, linestyle='--')
gl1.top_labels = gl1.right_labels = False
# Wind Speed Dual-Pol
img2 = axs[2].pcolormesh(dataset.longitude, dataset.latitude, windspeed_dual, cmap='jet', vmin=0, vmax=80, transform=ccrs.PlateCarree())
axs[2].add_feature(cfeature.COASTLINE)
axs[2].add_feature(cfeature.BORDERS, linestyle=':')
axs[2].set_title('Wind Speed Dual-Pol')
gl2 = axs[2].gridlines(draw_labels=True, color='gray', alpha=0.5, linestyle='--')
gl2.top_labels = gl2.right_labels = False
fig.colorbar(img0, ax=axs, orientation='horizontal', fraction=0.02, pad=0.1, aspect=40)
plt.show()
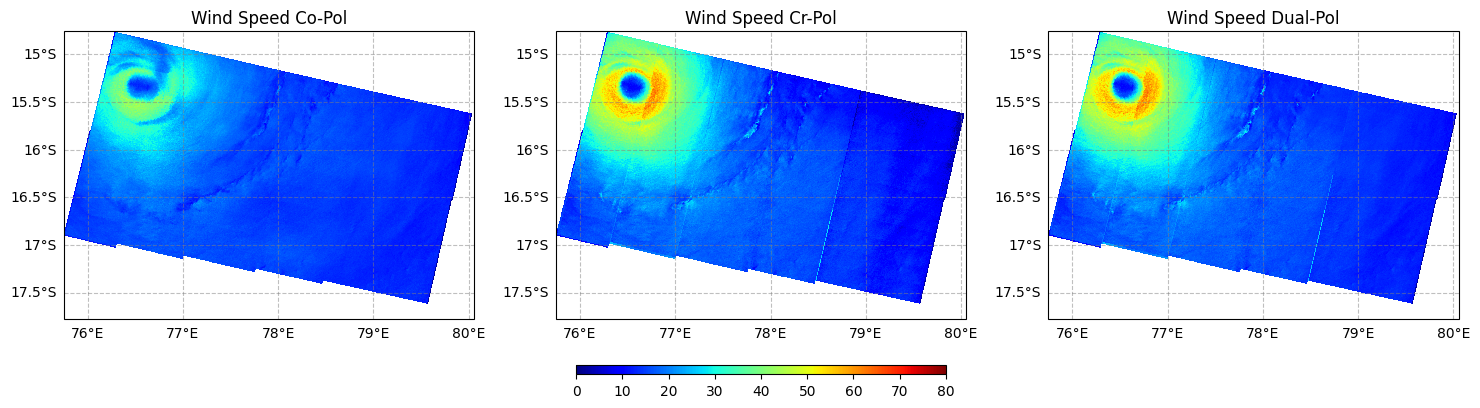
winddir illustration
[23]:
sub_ds = dataset.sel(pol='VV').isel(sample=slice(None, None, 10), line=slice(None, None, 10))
vectorfield = hv.VectorField(
(
sub_ds.sample, sub_ds.line,
xsarsea.dir_meteo_to_sample(sub_ds.winddir_dual,sub_ds.ground_heading),
sub_ds.windspeed_dual
)
)
hv.Image(dataset.windspeed_dual, kdims=['sample','line']).opts(title='speed and dir', clim=(0,50), cmap='jet') * vectorfield
[23]:
[24]:
sub_ds = dataset.sel(pol='VV').isel(sample=slice(None, None, 10), line=slice(None, None, 10))
vectorfield = hv.VectorField(
(
sub_ds.sample, sub_ds.line,
xsarsea.dir_meteo_to_sample(sub_ds.ancillary_wind_direction,sub_ds.ground_heading),
sub_ds.ancillary_wind_speed
)
)
hv.Image(dataset.ancillary_wind_speed, kdims=['sample','line']).opts(title='ECMWF speed and dir', clim=(0,50), cmap='jet') * vectorfield
[24]:
[ ]:
saving
delete useless variables
[25]:
# prepare dataset for netcdf export
dataset['sigma0_ocean_VV'] = dataset['sigma0_ocean'].sel(pol='VV')
dataset['sigma0_ocean_VH'] = dataset['sigma0_ocean'].sel(pol='VH')
black_list = ['model_U10', 'model_V10', 'digital_number', 'gamma0_raw', 'negz',
'azimuth_time', 'slant_range_time', 'velocity', 'range_ground_spacing',
'gamma0', 'time', 'sigma0', 'nesz', 'sigma0_raw', 'sigma0_ocean', 'altitude', 'elevation',
'nd_co', 'nd_cr']
variables = list(set(dataset) - set(black_list))
dataset = dataset[variables]
remove complex
[26]:
del dataset['ancillary_wind']
[27]:
ds_1000 = dataset.compute()
ds_1000
[27]:
<xarray.Dataset> Dimensions: (line: 240, sample: 417, pol: 2) Coordinates: * pol (pol) object 'VV' 'VH' * line (line) float64 12.0 37.0 ... 5.962e+03 5.987e+03 * sample (sample) float64 12.0 37.0 ... 1.039e+04 1.041e+04 spatial_ref int64 0 Data variables: (12/23) windspeed_dual (line, sample) float64 nan nan 1.5 ... 9.0 10.0 ancillary_wind_speed (line, sample) float64 11.03 11.07 ... 13.71 13.73 incidence (line, sample) float64 19.47 19.55 ... 46.52 46.57 windspeed_cross (line, sample) float64 nan nan 3.0 ... 6.8 7.0 3.0 sigma0_ocean_VH (line, sample) float64 nan nan ... 0.0002512 1e-15 winddir_dual (line, sample) float64 nan nan ... 136.8 140.8 ... ... latitude (line, sample) float64 -15.63 -15.63 ... -16.89 winddir_co (line, sample) float64 nan nan ... 136.8 140.8 gamma0_lut (pol, line, sample) float64 1.818e+03 ... 1.056... sampleSpacing float64 1e+03 ground_heading (line, sample) float32 -167.1 -167.1 ... -167.2 ancillary_wind_direction (line, sample) float64 55.97 56.17 ... 131.1 131.2 Attributes: (12/15) name: SENTINEL1_DS:/tmp/S1A_EW_GRDM_1SDV_20230217T002336_202... short_name: SENTINEL1_DS:S1A_EW_GRDM_1SDV_20230217T002336_20230217... product: GRDM safe: S1A_EW_GRDM_1SDV_20230217T002336_20230217T002412_04726... swath: EW multidataset: False ... ... start_date: 2023-02-17 00:23:36.783295 stop_date: 2023-02-17 00:24:12.397167 footprint: POLYGON ((80.05316482421334 -15.62417982506369, 76.271... coverage: 244km * 417km (line * sample ) orbit_pass: Descending platform_heading: -167.8308256427968
xarray.Dataset
- line: 240
- sample: 417
- pol: 2
- pol(pol)object'VV' 'VH'
array(['VV', 'VH'], dtype=object)
- line(line)float6412.0 37.0 ... 5.962e+03 5.987e+03
array([ 12., 37., 62., ..., 5937., 5962., 5987.])
- sample(sample)float6412.0 37.0 ... 1.039e+04 1.041e+04
array([ 12., 37., 62., ..., 10362., 10387., 10412.])
- spatial_ref()int640
- crs_wkt :
- GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AXIS["Latitude",NORTH],AXIS["Longitude",EAST],AUTHORITY["EPSG","4326"]]
- semi_major_axis :
- 6378137.0
- semi_minor_axis :
- 6356752.314245179
- inverse_flattening :
- 298.257223563
- reference_ellipsoid_name :
- WGS 84
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- WGS 84
- horizontal_datum_name :
- World Geodetic System 1984
- grid_mapping_name :
- latitude_longitude
- spatial_ref :
- GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AXIS["Latitude",NORTH],AXIS["Longitude",EAST],AUTHORITY["EPSG","4326"]]
- gcps :
- {'type': 'FeatureCollection', 'features': [{'type': 'Feature', 'properties': {'id': 'ba2f9888-aa84-467e-a5b8-b51574f092e3', 'info': None, 'row': 0, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [80.04782947416675, -15.627522836227252, 0]}}, {'type': 'Feature', 'properties': {'id': 'd45d2066-1640-4e73-896f-563f19591f4e', 'info': None, 'row': 0, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.85686777318767, -15.585523700616232, 0]}}, {'type': 'Feature', 'properties': {'id': 'a81645c4-37dd-4fc5-bf11-0ebed39f1f2c', 'info': None, 'row': 0, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.66598302214089, -15.543360414179306, 0]}}, {'type': 'Feature', 'properties': {'id': '916d6988-dac4-462a-af95-f126f0282241', 'info': None, 'row': 0, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.47517527508023, -15.50103352558102, 0]}}, {'type': 'Feature', 'properties': {'id': '5b07d30f-7d9c-459f-91af-9f34c51e02c0', 'info': None, 'row': 0, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.28444467673147, -15.458543510510118, 0]}}, {'type': 'Feature', 'properties': {'id': '05a36bdf-66f9-42c5-a8e0-864694b9e49b', 'info': None, 'row': 0, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [79.09379130979363, -15.415891049175503, 0]}}, {'type': 'Feature', 'properties': {'id': 'cc81b75c-21bb-4087-98e8-a98203962ca4', 'info': None, 'row': 0, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.90321528461942, -15.373076563918017, 0]}}, {'type': 'Feature', 'properties': {'id': '3a562299-6c5a-4015-b215-87c2008f5b78', 'info': None, 'row': 0, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.7127166481353, -15.330100647856336, 0]}}, {'type': 'Feature', 'properties': {'id': '2bf2fbbe-5300-41bd-8d34-fda5b9342b09', 'info': None, 'row': 0, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.52229545708146, -15.286963801581576, 0]}}, {'type': 'Feature', 'properties': {'id': 'd2402ad7-85b9-4a12-8b33-5e440bdc958c', 'info': None, 'row': 0, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.3319517544089, -15.243666568537671, 0]}}, {'type': 'Feature', 'properties': {'id': 'fdbfc19f-50f4-4713-a82e-711e18690483', 'info': None, 'row': 0, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [78.14168556997221, -15.200209540850494, 0]}}, {'type': 'Feature', 'properties': {'id': 'eae4f06d-b3a9-4722-a8a1-2706557886c3', 'info': None, 'row': 0, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.9514969405715, -15.156593267138204, 0]}}, {'type': 'Feature', 'properties': {'id': '9d278096-a532-4b6a-adba-cc765f8d50e6', 'info': None, 'row': 0, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.76138591144387, -15.11281823352861, 0]}}, {'type': 'Feature', 'properties': {'id': '60e067c7-0ef8-4dbc-b233-c7cd386d8d42', 'info': None, 'row': 0, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.57135250202873, -15.068884997007824, 0]}}, {'type': 'Feature', 'properties': {'id': 'f97df3ab-6f0f-4289-be0f-a2f8d5040489', 'info': None, 'row': 0, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.38139672764503, -15.024794095608083, 0]}}, {'type': 'Feature', 'properties': {'id': 'cab95056-e706-409e-96fd-fa91663f0eeb', 'info': None, 'row': 0, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [77.19151859460197, -14.980546081820966, 0]}}, {'type': 'Feature', 'properties': {'id': '8e7633b6-5030-453e-b593-dc57886159fd', 'info': None, 'row': 0, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [77.0017180981444, -14.936141550420118, 0]}}, {'type': 'Feature', 'properties': {'id': '11f0422f-7898-4978-bf17-2e0f1aa6ae65', 'info': None, 'row': 0, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.81199527073566, -14.891580954829292, 0]}}, {'type': 'Feature', 'properties': {'id': 'fc2f4302-165e-44f2-a292-dfa54160d800', 'info': None, 'row': 0, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.62235010538306, -14.846864909138356, 0]}}, {'type': 'Feature', 'properties': {'id': 'e6255f68-2e69-41b1-ab78-3858b3b262ad', 'info': None, 'row': 0, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.43278255754416, -14.801994052958182, 0]}}, {'type': 'Feature', 'properties': {'id': 'aaf3e979-0ffd-40bb-997a-3c33f559cc70', 'info': None, 'row': 13, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [80.02154379000997, -15.74405879183486, 0]}}, {'type': 'Feature', 'properties': {'id': '4b6bad26-61a1-49da-b6b8-e306f8b1b8a6', 'info': None, 'row': 13, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.83047886035003, -15.702037403761045, 0]}}, {'type': 'Feature', 'properties': {'id': 'fdf23600-d8ae-41df-99a0-8ce06530ce05', 'info': None, 'row': 13, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.63949158636065, -15.659850580050504, 0]}}, {'type': 'Feature', 'properties': {'id': 'd328d450-ebc8-47b8-aa62-77341b9c3a55', 'info': None, 'row': 13, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.44858202464096, -15.617498865461162, 0]}}, {'type': 'Feature', 'properties': {'id': '62b88ae2-ac4c-44be-af34-825c6c54ccdd', 'info': None, 'row': 13, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.25775031820537, -15.574982753813766, 0]}}, {'type': 'Feature', 'properties': {'id': '854974d3-44d0-4f1f-9394-b75bac5ac083', 'info': None, 'row': 13, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [79.066996557428, -15.532302904333973, 0]}}, {'type': 'Feature', 'properties': {'id': '453dafeb-63fc-408a-a1b4-0038e27ad3c5', 'info': None, 'row': 13, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.87632085183682, -15.48945975647899, 0]}}, {'type': 'Feature', 'properties': {'id': '77c52c9b-117e-48c0-9593-defb874ef73a', 'info': None, 'row': 13, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.68572325793525, -15.446453876408912, 0]}}, {'type': 'Feature', 'properties': {'id': '83ee4d20-b6c3-410c-8146-d0b61bba8db3', 'info': None, 'row': 13, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.49520381546589, -15.403285851253731, 0]}}, {'type': 'Feature', 'properties': {'id': 'f6bbbace-c890-4566-83e7-5462aa5cbadd', 'info': None, 'row': 13, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.30476259378298, -15.359956127126656, 0]}}, {'type': 'Feature', 'properties': {'id': '1841dfa0-f664-4da9-892d-86ceb7614dca', 'info': None, 'row': 13, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [78.11439961416535, -15.316465347327282, 0]}}, {'type': 'Feature', 'properties': {'id': 'f5e5d013-4ce1-49c2-9c3e-2ea82fce84b0', 'info': None, 'row': 13, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.92411492231693, -15.272814038131768, 0]}}, {'type': 'Feature', 'properties': {'id': 'f252f60a-30c4-495e-9319-90e62bf8a4ac', 'info': None, 'row': 13, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.73390856925202, -15.229002676860118, 0]}}, {'type': 'Feature', 'properties': {'id': '3c28441c-2dc8-4019-b1eb-0c1fb99e9977', 'info': None, 'row': 13, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.54378056273217, -15.185031883861235, 0]}}, {'type': 'Feature', 'properties': {'id': 'c65636e3-f258-48f3-9210-a54468ce4565', 'info': None, 'row': 13, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.35373092276959, -15.140902192153433, 0]}}, {'type': 'Feature', 'properties': {'id': '9919592d-cf75-4d08-958d-226fa920e4e8', 'info': None, 'row': 13, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [77.16375967396554, -15.096614093543948, 0]}}, {'type': 'Feature', 'properties': {'id': 'e90489ea-2237-434c-9e10-15b89a42ec41', 'info': None, 'row': 13, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.9738668149499, -15.052168183715878, 0]}}, {'type': 'Feature', 'properties': {'id': '0492350d-5eb8-451f-a3d4-7121144ba43d', 'info': None, 'row': 13, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.78405235587792, -15.007565021613729, 0]}}, {'type': 'Feature', 'properties': {'id': '91aa9669-a403-459a-958d-c37df71cfa1a', 'info': None, 'row': 13, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.59431632138948, -14.96280510676387, 0]}}, {'type': 'Feature', 'properties': {'id': 'ec7942b6-0c6d-4c8f-b401-8497196b6d2f', 'info': None, 'row': 13, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.4046586636428, -14.91788910621785, 0]}}, {'type': 'Feature', 'properties': {'id': '5a1e455c-6bf5-42f7-8dd8-c33ef87c8965', 'info': None, 'row': 26, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.9952388014265, -15.860591942130634, 0]}}, {'type': 'Feature', 'properties': {'id': 'e13d9716-7197-46ce-8dc9-b4ff44a6e682', 'info': None, 'row': 26, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.80406998521077, -15.818548242997513, 0]}}, {'type': 'Feature', 'properties': {'id': '81773a2e-8714-4b30-b162-9be1801700f7', 'info': None, 'row': 26, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.61297953237758, -15.776337822715224, 0]}}, {'type': 'Feature', 'properties': {'id': 'c1d13b04-beca-469f-955a-10c5c798dac5', 'info': None, 'row': 26, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.42196750091746, -15.733961227150965, 0]}}, {'type': 'Feature', 'properties': {'id': '203765c6-ba2e-42ca-81e1-b7e29b9ccb33', 'info': None, 'row': 26, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.23103403582486, -15.691418952299706, 0]}}, {'type': 'Feature', 'properties': {'id': '501f69da-6344-4e05-aa53-807bf55d6283', 'info': None, 'row': 26, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [79.04017923016028, -15.648711658093388, 0]}}, {'type': 'Feature', 'properties': {'id': '8d27aec1-8572-4f3a-9900-2b70cb3fc3d7', 'info': None, 'row': 26, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.84940319631531, -15.605839785288985, 0]}}, {'type': 'Feature', 'properties': {'id': 'f4599b0c-bea8-4e7a-8bc8-653f358ac6d5', 'info': None, 'row': 26, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.65870599704586, -15.562803887424609, 0]}}, {'type': 'Feature', 'properties': {'id': '2b16a8e1-bb60-45df-8f8a-78815e61cf44', 'info': None, 'row': 26, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.46808766286796, -15.519604605193942, 0]}}, {'type': 'Feature', 'properties': {'id': '3fbc0642-e0b6-4e3f-92a7-51f42509f7d1', 'info': None, 'row': 26, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.27754828031546, -15.476242326722563, 0]}}, {'type': 'Feature', 'properties': {'id': '084dfb71-2386-4c49-9d3c-592794676f59', 'info': None, 'row': 26, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [78.08708786753533, -15.43271772352058, 0]}}, {'type': 'Feature', 'properties': {'id': '1da9bda2-4fdc-4856-b279-c41b40c3760c', 'info': None, 'row': 26, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.89670647461632, -15.38903131855152, 0]}}, {'type': 'Feature', 'properties': {'id': '03fb858e-66bc-411d-9070-c414bc8760ca', 'info': None, 'row': 26, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.70640415905689, -15.345183577379984, 0]}}, {'type': 'Feature', 'properties': {'id': '813c6b82-96b5-40d8-86fd-95af0540c35b', 'info': None, 'row': 26, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.51618092434192, -15.301175153097384, 0]}}, {'type': 'Feature', 'properties': {'id': '291a2492-acc9-4ef5-b7bc-ce0d23fd58e0', 'info': None, 'row': 26, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.32603679579837, -15.257006571532193, 0]}}, {'type': 'Feature', 'properties': {'id': 'c8a1dcef-6b0f-489a-b125-3f15b9e37599', 'info': None, 'row': 26, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [77.13597179940689, -15.212678333449114, 0]}}, {'type': 'Feature', 'properties': {'id': 'b45f95e2-40b9-49f6-b8be-a1eda28bb838', 'info': None, 'row': 26, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.94598594769317, -15.16819099227186, 0]}}, {'type': 'Feature', 'properties': {'id': 'd34f0c87-d07d-4da6-b899-d65cd2a4ca38', 'info': None, 'row': 26, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.7560792384461, -15.123545172025544, 0]}}, {'type': 'Feature', 'properties': {'id': '58b77f8a-a968-4c7b-b9e7-3ad149dc487d', 'info': None, 'row': 26, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.56625171206937, -15.07874132254029, 0]}}, {'type': 'Feature', 'properties': {'id': 'a0cc606e-bfb0-4469-b62c-714339603be6', 'info': None, 'row': 26, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.37650332251398, -15.033780117586018, 0]}}, {'type': 'Feature', 'properties': {'id': '8002de1a-1ae8-4612-af1a-dcd51b999a28', 'info': None, 'row': 39, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.96891289971403, -15.977122053338592, 0]}}, {'type': 'Feature', 'properties': {'id': 'e2b75aff-7d49-4bbc-9faa-3d698cf1783a', 'info': None, 'row': 39, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.77763948424479, -15.9350559796666, 0]}}, {'type': 'Feature', 'properties': {'id': 'c5fd754b-7f1e-4b09-92a4-ee0ff94c46d5', 'info': None, 'row': 39, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.58644514200805, -15.892821898572922, 0]}}, {'type': 'Feature', 'properties': {'id': '8c827139-7129-450c-bde8-eaf6804df2ff', 'info': None, 'row': 39, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.395329931136, -15.8504203624679, 0]}}, {'type': 'Feature', 'properties': {'id': 'f86c1d09-5533-48cd-a8b7-de227b59859a', 'info': None, 'row': 39, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.20429400260204, -15.807851852233126, 0]}}, {'type': 'Feature', 'properties': {'id': '71c271cf-bd4f-4b57-84cc-f46d4011cbf1', 'info': None, 'row': 39, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [79.01333744674862, -15.765117052003827, 0]}}, {'type': 'Feature', 'properties': {'id': '75c58883-a215-43fd-9180-37cd48fa5897', 'info': None, 'row': 39, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.82246038282659, -15.722216386702085, 0]}}, {'type': 'Feature', 'properties': {'id': '0bfe4d68-22a0-4871-9738-39d40355c492', 'info': None, 'row': 39, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.63166287624297, -15.679150412775346, 0]}}, {'type': 'Feature', 'properties': {'id': 'e35578a8-be50-48a4-95fb-d00f03b2bb6e', 'info': None, 'row': 39, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.44094495670582, -15.635919788757874, 0]}}, {'type': 'Feature', 'properties': {'id': 'f59cc90f-11f4-4818-a753-a3f3e0f6204e', 'info': None, 'row': 39, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.25030671793624, -15.592524887409297, 0]}}, {'type': 'Feature', 'properties': {'id': 'c2055320-6542-4148-b82c-e9e4ba32ae4e', 'info': None, 'row': 39, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [78.05974818084684, -15.548966383573427, 0]}}, {'type': 'Feature', 'properties': {'id': 'c9862165-d498-48a5-acb8-70c46e8fb864', 'info': None, 'row': 39, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.8692693950158, -15.505244817516308, 0]}}, {'type': 'Feature', 'properties': {'id': '2072d279-9197-4279-9479-2eb5eb8758e8', 'info': None, 'row': 39, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.6788704251915, -15.461360639853902, 0]}}, {'type': 'Feature', 'properties': {'id': '6f0a7730-a180-4ce2-be5e-bbb0607981c6', 'info': None, 'row': 39, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.48855127860014, -15.417314503248162, 0]}}, {'type': 'Feature', 'properties': {'id': 'b2bff5bf-199d-4c52-9bb3-74c0762502b2', 'info': None, 'row': 39, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.29831198655671, -15.373106923980473, 0]}}, {'type': 'Feature', 'properties': {'id': '4ec6a558-fe7e-414d-87ea-01428361a190', 'info': None, 'row': 39, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [77.10815255809918, -15.328738487218304, 0]}}, {'type': 'Feature', 'properties': {'id': '922d8e04-a95b-4c13-8307-a5afad555788', 'info': None, 'row': 39, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.91807303103562, -15.284209657359746, 0]}}, {'type': 'Feature', 'properties': {'id': '7084890d-fffc-4c50-a542-e043f22f1623', 'info': None, 'row': 39, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.72807340155904, -15.239521079700348, 0]}}, {'type': 'Feature', 'properties': {'id': '212cac80-92a2-47c9-85b2-68c50f775423', 'info': None, 'row': 39, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.53815370864558, -15.19467322464686, 0]}}, {'type': 'Feature', 'properties': {'id': '04de4374-351e-4fd6-99e1-6f21f27c73c1', 'info': None, 'row': 39, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.34831391355536, -15.149666750238378, 0]}}, {'type': 'Feature', 'properties': {'id': '895c328d-9b7d-4c1a-bfb9-6e95dd2c91dd', 'info': None, 'row': 52, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.94254943869474, -16.093646708962577, 0]}}, {'type': 'Feature', 'properties': {'id': 'fb8a1be1-a014-4e61-86cd-a097f10656ff', 'info': None, 'row': 52, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.7511701539668, -16.05155814707808, 0]}}, {'type': 'Feature', 'properties': {'id': '750d96df-8f1d-4886-946b-eeb5ab31550b', 'info': None, 'row': 52, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.55987065642178, -16.009300289364102, 0]}}, {'type': 'Feature', 'properties': {'id': '22bf9324-6f9d-497c-96ee-8de5b6bca83f', 'info': None, 'row': 52, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.36865100648603, -15.966873685142936, 0]}}, {'type': 'Feature', 'properties': {'id': '6fa9d569-2478-41ae-828c-659221ad0acf', 'info': None, 'row': 52, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.17751135404725, -15.924278830904044, 0]}}, {'type': 'Feature', 'properties': {'id': '49631e17-f763-475e-abb0-db91d4eb5987', 'info': None, 'row': 52, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.98645179642325, -15.881516393322055, 0]}}, {'type': 'Feature', 'properties': {'id': '3b8f9d09-d4c4-4a8c-8dd6-425745b96eff', 'info': None, 'row': 52, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.79547245225261, -15.838586813881983, 0]}}, {'type': 'Feature', 'properties': {'id': 'a44a1577-d6bf-4902-b35a-073d76ffe0a8', 'info': None, 'row': 52, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.60457339060486, -15.795490647692745, 0]}}, {'type': 'Feature', 'properties': {'id': '30a651e0-6ac1-4ee6-8cfc-690e946ff33b', 'info': None, 'row': 52, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.41375465334492, -15.752228516544951, 0]}}, {'type': 'Feature', 'properties': {'id': '7369534e-53b6-4953-a6b4-b20426c59445', 'info': None, 'row': 52, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.22301631307441, -15.708800897314603, 0]}}, {'type': 'Feature', 'properties': {'id': 'f5748488-72e4-4a70-a81a-0b9440b3b078', 'info': None, 'row': 52, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [78.03235841663368, -15.66520837081663, 0]}}, {'type': 'Feature', 'properties': {'id': '7c0da942-c69b-497b-b975-37d1c3208a8f', 'info': None, 'row': 52, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.84178101794755, -15.621451474937437, 0]}}, {'type': 'Feature', 'properties': {'id': 'a7573c0f-1c9a-44cd-bc04-02a2ed81662d', 'info': None, 'row': 52, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.65128416131466, -15.577530760646406, 0]}}, {'type': 'Feature', 'properties': {'id': '5bec0b27-4419-4a0c-bb03-d01c34368043', 'info': None, 'row': 52, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.46086787904663, -15.533446791742378, 0]}}, {'type': 'Feature', 'properties': {'id': 'd70ecd29-24d5-4419-9805-81c6767501fd', 'info': None, 'row': 52, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.27053220182725, -15.48920010263801, 0]}}, {'type': 'Feature', 'properties': {'id': '31878aff-890f-4c7a-bb15-650cd338ed83', 'info': None, 'row': 52, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [77.08027714482414, -15.44479126856391, 0]}}, {'type': 'Feature', 'properties': {'id': '23719eeb-4444-4997-bdc4-f3efa7c60e9f', 'info': None, 'row': 52, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.89010272989196, -15.400220833909565, 0]}}, {'type': 'Feature', 'properties': {'id': '353e36e5-8541-4dc3-820d-f08204881b5e', 'info': None, 'row': 52, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.70000898281785, -15.355489338189606, 0]}}, {'type': 'Feature', 'properties': {'id': 'f9636a2a-6631-4654-9aad-58ea97e71a03', 'info': None, 'row': 52, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.50999591880208, -15.310597362922904, 0]}}, {'type': 'Feature', 'properties': {'id': '2d528bc6-bc20-411e-a9f5-518295823862', 'info': None, 'row': 52, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.32006352257244, -15.265545485306331, 0]}}, {'type': 'Feature', 'properties': {'id': '74a826b1-c9ce-4653-8feb-31e059f2125c', 'info': None, 'row': 65, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.91616777456161, -16.210168721272243, 0]}}, {'type': 'Feature', 'properties': {'id': '1be13ec5-5a3a-4388-b07b-1c2c0be1d7a8', 'info': None, 'row': 65, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.72468200919309, -16.168057616316936, 0]}}, {'type': 'Feature', 'properties': {'id': '1aed4c72-6f45-4bb4-944d-6e2ee780c27c', 'info': None, 'row': 65, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.53327670380419, -16.125775918995902, 0]}}, {'type': 'Feature', 'properties': {'id': '24ff9fb8-98a0-47be-87bb-f6806322cda7', 'info': None, 'row': 65, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.34195206442936, -16.08332420387183, 0]}}, {'type': 'Feature', 'properties': {'id': '8164ec78-fb86-408b-a262-48cbb705ded5', 'info': None, 'row': 65, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.15070820964986, -16.04070297244796, 0]}}, {'type': 'Feature', 'properties': {'id': '99690bd2-f0ff-494e-8cc5-25e9eae7d117', 'info': None, 'row': 65, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.9595451789406, -15.99791285692123, 0]}}, {'type': 'Feature', 'properties': {'id': '5aecf24a-9f72-4ecc-953d-4e5324e11f78', 'info': None, 'row': 65, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.76846304008266, -15.954954324891908, 0]}}, {'type': 'Feature', 'properties': {'id': '953aac6e-248f-4506-a13e-dbc64b0ade98', 'info': None, 'row': 65, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.57746185210803, -15.911827926090965, 0]}}, {'type': 'Feature', 'properties': {'id': 'd1be9ff6-28f8-4395-91b3-38bccaa3f062', 'info': None, 'row': 65, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.38654168309472, -15.8685342348591, 0]}}, {'type': 'Feature', 'properties': {'id': 'b6ce9058-7b84-4997-b41d-7ace54616f6b', 'info': None, 'row': 65, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.1957026113124, -15.82507382032651, 0]}}, {'type': 'Feature', 'properties': {'id': '0876279f-884f-413a-8e53-62ed8b978848', 'info': None, 'row': 65, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [78.00494472601972, -15.78144719876348, 0]}}, {'type': 'Feature', 'properties': {'id': '84a910fc-22c8-4941-93c9-08bf9cb48d9f', 'info': None, 'row': 65, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.81426809709393, -15.737654917064214, 0]}}, {'type': 'Feature', 'properties': {'id': '8e9cc722-352c-43f0-a504-12251ddc4eca', 'info': None, 'row': 65, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.6236727591549, -15.693697590958088, 0]}}, {'type': 'Feature', 'properties': {'id': 'ae8f49cf-8c1a-4c8c-8433-ec505d05ba82', 'info': None, 'row': 65, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.43315875532203, -15.64957572275883, 0]}}, {'type': 'Feature', 'properties': {'id': 'c0391fc1-6f91-4378-bf4b-8bcc4ab85575', 'info': None, 'row': 65, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.24272610479203, -15.605289862106329, 0]}}, {'type': 'Feature', 'properties': {'id': '5268f61e-446c-49b7-9015-acdeae1c6316', 'info': None, 'row': 65, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [77.05237482177448, -15.560840571474769, 0]}}, {'type': 'Feature', 'properties': {'id': '5f3a0f7e-fd4f-41c8-9fcf-28350a9c0c66', 'info': None, 'row': 65, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.8621049197991, -15.516228452289912, 0]}}, {'type': 'Feature', 'properties': {'id': '795967b4-2e2b-48dd-9a9a-df07edb892e9', 'info': None, 'row': 65, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.67191645775394, -15.47145397388131, 0]}}, {'type': 'Feature', 'properties': {'id': '0ef0eaed-7ee7-4081-832a-e6a799053f6f', 'info': None, 'row': 65, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.48180944984635, -15.426517788380313, 0]}}, {'type': 'Feature', 'properties': {'id': 'ecb20a44-9af9-4ce7-9c86-f45c3ab04158', 'info': None, 'row': 65, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.29178387803867, -15.381420450640169, 0]}}, {'type': 'Feature', 'properties': {'id': 'b81d088a-dde2-489f-a958-00e3d35bfb4d', 'info': None, 'row': 78, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.8897600173751, -16.3266869447705, 0]}}, {'type': 'Feature', 'properties': {'id': '338de712-1267-415a-8a22-c595985331cc', 'info': None, 'row': 78, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.69816689621551, -16.284553218177884, 0]}}, {'type': 'Feature', 'properties': {'id': '191d7151-7afd-4ce4-af26-20dfaf907b96', 'info': None, 'row': 78, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.50665483599974, -16.242247588920232, 0]}}, {'type': 'Feature', 'properties': {'id': '20007587-57db-423a-84bd-7e2caa4cf796', 'info': None, 'row': 78, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.31522443614959, -16.19977070722914, 0]}}, {'type': 'Feature', 'properties': {'id': '7638cf26-c7b3-44e1-bb78-fb0f5b76f77f', 'info': None, 'row': 78, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.1238757326443, -16.15712305685052, 0]}}, {'type': 'Feature', 'properties': {'id': '4787a92b-346d-4002-9774-03ccbd11dc9f', 'info': None, 'row': 78, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.9326085935108, -16.114305212055772, 0]}}, {'type': 'Feature', 'properties': {'id': 'fde5dc6c-0194-4982-aac4-f9fc132a41c5', 'info': None, 'row': 78, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.7414229497715, -16.071317678422755, 0]}}, {'type': 'Feature', 'properties': {'id': '70d01137-018e-46f9-accc-46aa413a04a6', 'info': None, 'row': 78, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.5503188265589, -16.028160993924644, 0]}}, {'type': 'Feature', 'properties': {'id': 'bfec4355-da17-42ce-8ed0-1990c6c78cef', 'info': None, 'row': 78, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.35929634062643, -15.984835674792961, 0]}}, {'type': 'Feature', 'properties': {'id': '0fa6a456-3669-4666-b6ae-914dbedb08f7', 'info': None, 'row': 78, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.16835562636705, -15.941342344641276, 0]}}, {'type': 'Feature', 'properties': {'id': 'd49a4c0a-0fa1-4714-8df4-976a4fdb6bdf', 'info': None, 'row': 78, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.97749684066295, -15.8976815202805, 0]}}, {'type': 'Feature', 'properties': {'id': 'd254d5da-5936-4d68-bdba-4ca5eb527411', 'info': None, 'row': 78, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.78672008873393, -15.853853780164638, 0]}}, {'type': 'Feature', 'properties': {'id': 'e4eb7efd-fefa-421b-bd33-ddc6ccbb4613', 'info': None, 'row': 78, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.59602541816248, -15.809859726534313, 0]}}, {'type': 'Feature', 'properties': {'id': '050621e2-c8c3-4529-af2b-3e8009a9715f', 'info': None, 'row': 78, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.40541285508078, -15.765699860369272, 0]}}, {'type': 'Feature', 'properties': {'id': '40bb08cd-f736-4271-a5e2-fa4725ca613c', 'info': None, 'row': 78, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.21488238936095, -15.721374737516628, 0]}}, {'type': 'Feature', 'properties': {'id': '01f1bd4e-b60a-4cc0-99c3-ae81e8352b6a', 'info': None, 'row': 78, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [77.02443401865648, -15.676884916422438, 0]}}, {'type': 'Feature', 'properties': {'id': 'e8160b88-bab5-4d4f-a5f1-641f0ba9c5db', 'info': None, 'row': 78, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.83406776732642, -15.632230991429296, 0]}}, {'type': 'Feature', 'properties': {'id': '99e0efae-4546-4d06-b0dc-57f8c1c07a51', 'info': None, 'row': 78, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.64378372893106, -15.587413441629488, 0]}}, {'type': 'Feature', 'properties': {'id': '04aabe26-5e8d-45e8-a6e1-c318f1b04825', 'info': None, 'row': 78, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.45358195934794, -15.54243290659782, 0]}}, {'type': 'Feature', 'properties': {'id': '893d5a18-f6ce-49f3-b7df-88d3ad6c2e5e', 'info': None, 'row': 78, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.263462390031, -15.497290029503171, 0]}}, {'type': 'Feature', 'properties': {'id': '208f9cb5-3a0f-4040-af41-33e078408885', 'info': None, 'row': 91, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.86331856102629, -16.443200282031302, 0]}}, {'type': 'Feature', 'properties': {'id': 'bcaaa84c-cf15-4a1c-a226-1004184a49da', 'info': None, 'row': 91, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.67161684764505, -16.40104380687674, 0]}}, {'type': 'Feature', 'properties': {'id': '0df80c4d-b3b3-4944-9b7f-71dcb25e6eb7', 'info': None, 'row': 91, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.47999700817556, -16.358714152231645, 0]}}, {'type': 'Feature', 'properties': {'id': '009f8f8e-5267-4da8-857f-30166599ee91', 'info': None, 'row': 91, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.28845940292801, -16.316211897600308, 0]}}, {'type': 'Feature', 'properties': {'id': 'a09c2ac4-616f-4a92-92be-a40a4ee1d201', 'info': None, 'row': 91, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.09700410094061, -16.273537620390474, 0]}}, {'type': 'Feature', 'properties': {'id': '4d26271e-abd5-490e-998c-b76e9dea3296', 'info': None, 'row': 91, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.90563110383371, -16.230691823347712, 0]}}, {'type': 'Feature', 'properties': {'id': '8f7b4917-8f9e-49f5-b3f9-18815fc1677c', 'info': None, 'row': 91, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.71434041036768, -16.187675076197603, 0]}}, {'type': 'Feature', 'properties': {'id': '3ac827ea-4077-42a8-a4b5-5e8d4858afd2', 'info': None, 'row': 91, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.52313207103205, -16.144487923088846, 0]}}, {'type': 'Feature', 'properties': {'id': '51bf8f31-e6c0-4dac-a294-25f111c27511', 'info': None, 'row': 91, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.33200618930108, -16.10113086499536, 0]}}, {'type': 'Feature', 'properties': {'id': 'dee70da2-8f4e-4cb6-80ee-1569b609cd9e', 'info': None, 'row': 91, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.14096286267284, -16.05760452258809, 0]}}, {'type': 'Feature', 'properties': {'id': '149adebe-7a44-400c-9518-61abe4385d40', 'info': None, 'row': 91, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.9500022108623, -16.013909415339576, 0]}}, {'type': 'Feature', 'properties': {'id': '378a60ac-9baf-4f69-bd71-e8d2e2381b48', 'info': None, 'row': 91, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.75912432205183, -15.970046105253692, 0]}}, {'type': 'Feature', 'properties': {'id': 'eee230e5-1938-45d2-9b42-cd5a7eceff2b', 'info': None, 'row': 91, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.5683292406945, -15.92601520603543, 0]}}, {'type': 'Feature', 'properties': {'id': 'b3fc1d06-0051-4416-a8b3-f358c1ccf855', 'info': None, 'row': 91, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.37761700844045, -15.88181722266885, 0]}}, {'type': 'Feature', 'properties': {'id': '9bd5ba3f-9738-4a57-a3bb-521f3c33d5fd', 'info': None, 'row': 91, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.18698763831262, -15.837452710378287, 0]}}, {'type': 'Feature', 'properties': {'id': 'd4b85a43-0197-49b4-ae10-58b3ce091c75', 'info': None, 'row': 91, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.9964411440088, -15.792922230136273, 0]}}, {'type': 'Feature', 'properties': {'id': 'cdb834e4-977f-436a-9801-3a9d8fa958ac', 'info': None, 'row': 91, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.80597755223874, -15.74822636753494, 0]}}, {'type': 'Feature', 'properties': {'id': 'a029119a-0550-4b77-892f-d2af8fcc2452', 'info': None, 'row': 91, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.61559692410692, -15.703365663935621, 0]}}, {'type': 'Feature', 'properties': {'id': '17cb78c2-17ea-4476-a5db-11ce12c953f0', 'info': None, 'row': 91, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.42529931796315, -15.65834067631504, 0]}}, {'type': 'Feature', 'properties': {'id': '0e6b9f3e-b100-47cd-ba06-c6dee1d29736', 'info': None, 'row': 91, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.2350846827197, -15.613152071654897, 0]}}, {'type': 'Feature', 'properties': {'id': 'ddf2eb72-e97b-4b4f-a763-dfd0123c6439', 'info': None, 'row': 104, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.83685853299947, -16.559710922309186, 0]}}, {'type': 'Feature', 'properties': {'id': '76785d2a-7db1-49e7-a66f-306f9935b6a1', 'info': None, 'row': 104, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.64504761959066, -16.517531644551422, 0]}}, {'type': 'Feature', 'properties': {'id': '9a5bde4e-531e-4a20-93e3-561009901258', 'info': None, 'row': 104, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.45331940863828, -16.47517791339829, 0]}}, {'type': 'Feature', 'properties': {'id': 'a7ae8b1e-ccc3-47b8-a15e-6d688c3554fa', 'info': None, 'row': 104, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.2616739715036, -16.432650225691813, 0]}}, {'type': 'Feature', 'properties': {'id': 'e601a29f-a6c8-41bb-b61d-5435d1f974bb', 'info': None, 'row': 104, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.07011142184768, -16.38994925331621, 0]}}, {'type': 'Feature', 'properties': {'id': 'e20c500a-939f-4d2c-9138-7aa7746ae2df', 'info': None, 'row': 104, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.87863191595537, -16.347075442086805, 0]}}, {'type': 'Feature', 'properties': {'id': '1a0aa6f1-fad1-467d-8cf1-54d35bb8804e', 'info': None, 'row': 104, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.68723554000024, -16.30402941475372, 0]}}, {'type': 'Feature', 'properties': {'id': '329ba4e1-b969-4976-9af5-de33da34dae6', 'info': None, 'row': 104, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.49592237464307, -16.260811723708166, 0]}}, {'type': 'Feature', 'properties': {'id': 'dc8b56a0-3ea0-4a2e-bc52-39238fb95689', 'info': None, 'row': 104, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.30469250328419, -16.217422866651752, 0]}}, {'type': 'Feature', 'properties': {'id': '7b09a296-12f0-4c65-a4e6-194fd7653c74', 'info': None, 'row': 104, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.11354597998962, -16.173863453521214, 0]}}, {'type': 'Feature', 'properties': {'id': 'ad209d54-0651-4dc9-b266-712299cb594f', 'info': None, 'row': 104, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.92248287996851, -16.13013400283265, 0]}}, {'type': 'Feature', 'properties': {'id': '6e7d8dcc-0b39-41a4-931a-3515842c54ae', 'info': None, 'row': 104, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.73150326899798, -16.086235061646725, 0]}}, {'type': 'Feature', 'properties': {'id': '0a211f60-b266-493b-99d6-1d3a037d933a', 'info': None, 'row': 104, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.5406071884346, -16.04216725204798, 0]}}, {'type': 'Feature', 'properties': {'id': '25fb5f9f-176f-4db7-8263-e2d9813a742e', 'info': None, 'row': 104, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.34979469945637, -15.997931078017047, 0]}}, {'type': 'Feature', 'properties': {'id': '5a67507c-0e31-4c56-8278-99e30ca3acb4', 'info': None, 'row': 104, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.15906583633335, -15.953527118768953, 0]}}, {'type': 'Feature', 'properties': {'id': '6efb89e0-d7cc-4d41-a18c-064b8dfd6a51', 'info': None, 'row': 104, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.9684206373103, -15.908955914480808, 0]}}, {'type': 'Feature', 'properties': {'id': '0dd15426-be24-4bee-a013-2d47c668027e', 'info': None, 'row': 104, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.77785912879767, -15.864218050142822, 0]}}, {'type': 'Feature', 'properties': {'id': '4d25fadf-709b-43d4-ab54-4ccb610f391d', 'info': None, 'row': 104, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.58738133633356, -15.819314124651266, 0]}}, {'type': 'Feature', 'properties': {'id': '9407d631-19d8-48de-adf8-df64dbc756f9', 'info': None, 'row': 104, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.39698731428568, -15.774244620445087, 0]}}, {'type': 'Feature', 'properties': {'id': '25fb3f0b-dbc9-4747-858c-ad033ccdc290', 'info': None, 'row': 104, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.2066770367245, -15.729010214109834, 0]}}, {'type': 'Feature', 'properties': {'id': '9567fa92-447b-43d2-a1fb-c61c67fe3519', 'info': None, 'row': 117, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.81036701161318, -16.676216983832187, 0]}}, {'type': 'Feature', 'properties': {'id': '2df4b823-ab87-4029-a4be-c2c206577396', 'info': None, 'row': 117, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.61844596076592, -16.634014831994076, 0]}}, {'type': 'Feature', 'properties': {'id': 'e98f564e-53d3-4d14-8280-37d8defb75ca', 'info': None, 'row': 117, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.42660833897412, -16.591636938126126, 0]}}, {'type': 'Feature', 'properties': {'id': '94da8364-19ef-46e4-935c-e8e0af21adf8', 'info': None, 'row': 117, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.23485422282562, -16.549083783312113, 0]}}, {'type': 'Feature', 'properties': {'id': '2b52b93b-667d-42fe-8a7b-8dcaf4ae012d', 'info': None, 'row': 117, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.04318372407182, -16.506356058489562, 0]}}, {'type': 'Feature', 'properties': {'id': '33a239b2-c9c1-4690-94ab-f22c70da0770', 'info': None, 'row': 117, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.85159700300251, -16.463454205297435, 0]}}, {'type': 'Feature', 'properties': {'id': 'af1665a0-884d-4edc-88a7-34d91e918deb', 'info': None, 'row': 117, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.6600941486497, -16.4203788482653, 0]}}, {'type': 'Feature', 'properties': {'id': '3be61bc5-8b5f-4b06-98b7-11ab00e1df2d', 'info': None, 'row': 117, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.46867524471446, -16.377130541624126, 0]}}, {'type': 'Feature', 'properties': {'id': 'a97b3d6c-d9aa-4151-838b-2ed42bac5ff2', 'info': None, 'row': 117, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.27734037766976, -16.333709785384467, 0]}}, {'type': 'Feature', 'properties': {'id': 'ea92bd74-e4f7-4b34-82ef-7a3b2e42b4e2', 'info': None, 'row': 117, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.08608960375936, -16.290117195776872, 0]}}, {'type': 'Feature', 'properties': {'id': '139f250f-6ccf-4d18-a1f4-8a670d90ae05', 'info': None, 'row': 117, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.89492300514588, -16.246353278157496, 0]}}, {'type': 'Feature', 'properties': {'id': '1a884899-d7a3-411d-be5e-211e37a2a651', 'info': None, 'row': 117, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.70384064588706, -16.20241860264911, 0]}}, {'type': 'Feature', 'properties': {'id': '3dd1f81c-1cd0-4da5-877e-0bffced49aec', 'info': None, 'row': 117, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.51284257558244, -16.158313773141998, 0]}}, {'type': 'Feature', 'properties': {'id': '92a81954-7245-4a9a-84fa-4bd42aabdeae', 'info': None, 'row': 117, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.32192886411238, -16.114039274043975, 0]}}, {'type': 'Feature', 'properties': {'id': '02590575-deca-4e67-bd36-2e08d50c8544', 'info': None, 'row': 117, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.13109952469374, -16.069595786272167, 0]}}, {'type': 'Feature', 'properties': {'id': '39a0a167-e708-4015-8f6f-101cd84e49df', 'info': None, 'row': 117, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.94035462254038, -16.024983755516736, 0]}}, {'type': 'Feature', 'properties': {'id': '0706e3b8-c1ff-4e75-b0a0-e61d0e2fa2d3', 'info': None, 'row': 117, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.74969418183348, -15.980203791478242, 0]}}, {'type': 'Feature', 'properties': {'id': '58f8b8ed-5ec5-40d5-b2ac-03c2424909e3', 'info': None, 'row': 117, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.55911823022265, -15.935256499877863, 0]}}, {'type': 'Feature', 'properties': {'id': '7cffcf9b-ea42-47db-b02d-bb23f028bb25', 'info': None, 'row': 117, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.36862682635014, -15.890142361433837, 0]}}, {'type': 'Feature', 'properties': {'id': '603575c2-4677-453f-8466-779a68c20ec6', 'info': None, 'row': 117, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.17821994478098, -15.844862065290062, 0]}}, {'type': 'Feature', 'properties': {'id': 'f429ca21-12a3-4727-9781-0098244c72f2', 'info': None, 'row': 130, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.78384585768828, -16.792718756625753, 0]}}, {'type': 'Feature', 'properties': {'id': '1b00a8ff-1f3c-4a2c-adf7-bae2e08f4ced', 'info': None, 'row': 130, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.59181369776505, -16.750493641788612, 0]}}, {'type': 'Feature', 'properties': {'id': '44be76c9-43c6-4ba1-bb37-60a845382499', 'info': None, 'row': 130, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.39986569841203, -16.708091487188312, 0]}}, {'type': 'Feature', 'properties': {'id': 'efb708a0-4aa6-4d92-b88e-437d2df76622', 'info': None, 'row': 130, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.20800193596878, -16.665512781983004, 0]}}, {'type': 'Feature', 'properties': {'id': 'b01627b4-1e2f-45c5-9d64-9df4c1d024cc', 'info': None, 'row': 130, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [79.01622252590434, -16.62275821187316, 0]}}, {'type': 'Feature', 'properties': {'id': '9997dbf8-4342-4bb6-aa91-9d902d174bd0', 'info': None, 'row': 130, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.824527630642, -16.579828222518078, 0]}}, {'type': 'Feature', 'properties': {'id': '44392635-bee2-43a4-b9f7-c092425e9499', 'info': None, 'row': 130, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.63291734209511, -16.53672344024224, 0]}}, {'type': 'Feature', 'properties': {'id': '47d3a04f-3d1e-45d4-945a-402152b341d6', 'info': None, 'row': 130, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.44139174703422, -16.49344442113331, 0]}}, {'type': 'Feature', 'properties': {'id': 'd63c7468-991c-41e1-bf93-d6a5a9d0f5ae', 'info': None, 'row': 130, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.24995093560003, -16.449991665196826, 0]}}, {'type': 'Feature', 'properties': {'id': '1f2f0b7a-afd0-499a-9ada-f46d91161b46', 'info': None, 'row': 130, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.05859497263361, -16.40636576847348, 0]}}, {'type': 'Feature', 'properties': {'id': '88f5025b-5b74-4aa2-9a78-c75610da7fff', 'info': None, 'row': 130, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.86732393151664, -16.362567288800967, 0]}}, {'type': 'Feature', 'properties': {'id': 'fa5c2af3-0ef9-4e10-b2ba-0af561e400c0', 'info': None, 'row': 130, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.67613788060292, -16.318596794219694, 0]}}, {'type': 'Feature', 'properties': {'id': '4f436d48-8ee8-409b-b43a-dd3ecbb6c52f', 'info': None, 'row': 130, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.48503688911781, -16.274454823862932, 0]}}, {'type': 'Feature', 'properties': {'id': '05e5495b-4c71-420a-8b92-0f517529d1cf', 'info': None, 'row': 130, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.2940210109346, -16.230141943978577, 0]}}, {'type': 'Feature', 'properties': {'id': '5b072a4a-c52c-4467-922a-2d164c8641a1', 'info': None, 'row': 130, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.10309027717872, -16.185658778094112, 0]}}, {'type': 'Feature', 'properties': {'id': '3610b600-ed5e-4cde-83c1-0c2492e58660', 'info': None, 'row': 130, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.91224474285414, -16.141005829188423, 0]}}, {'type': 'Feature', 'properties': {'id': '76340119-9e2b-4e45-afe8-6be9ac403551', 'info': None, 'row': 130, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.7214844489279, -16.096183654572464, 0]}}, {'type': 'Feature', 'properties': {'id': '177f8874-8283-4418-8851-3f26314619bc', 'info': None, 'row': 130, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.53080942458166, -16.05119286944493, 0]}}, {'type': 'Feature', 'properties': {'id': '54ef31e4-9da4-4ecc-ba80-70e3934e7e57', 'info': None, 'row': 130, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.34021971692799, -16.006034016179168, 0]}}, {'type': 'Feature', 'properties': {'id': '43f71700-07dd-4660-993c-9b7691dad64a', 'info': None, 'row': 130, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.14971532045796, -15.96070771890222, 0]}}, {'type': 'Feature', 'properties': {'id': '2239ae33-f5a8-4fd4-b5ac-1914288b18fb', 'info': None, 'row': 143, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.75730575587254, -16.909217784035466, 0]}}, {'type': 'Feature', 'properties': {'id': '6d02fd6c-e186-41c2-935f-f7bddbdd1701', 'info': None, 'row': 143, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.56516186698143, -16.86696964948371, 0]}}, {'type': 'Feature', 'properties': {'id': 'ac14c73b-e9c7-41eb-b191-fa060ceb24b1', 'info': None, 'row': 143, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.37310287261923, -16.824543174701, 0]}}, {'type': 'Feature', 'properties': {'id': '24d599d7-0f9d-40b7-9e11-821eaf8607cb', 'info': None, 'row': 143, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.18112884756478, -16.781938862617935, 0]}}, {'type': 'Feature', 'properties': {'id': '3f3f9863-1d54-4c7d-a881-875680c7af50', 'info': None, 'row': 143, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [78.98923991234426, -16.739157387937095, 0]}}, {'type': 'Feature', 'properties': {'id': 'e6fc702c-a3e8-4e42-bd8a-5d60da35e50f', 'info': None, 'row': 143, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.79743623091744, -16.696199202986662, 0]}}, {'type': 'Feature', 'properties': {'id': '78f704ab-ddd5-4abe-8eea-afab0829fd10', 'info': None, 'row': 143, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.60571789863546, -16.653064933615266, 0]}}, {'type': 'Feature', 'properties': {'id': '9450bf94-aedf-4eeb-b40e-aac56cbbdfc8', 'info': None, 'row': 143, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.41408500480054, -16.609755140136922, 0]}}, {'type': 'Feature', 'properties': {'id': 'de0cb2af-6d20-4fb5-9d12-ed5a67527fba', 'info': None, 'row': 143, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.22253764356478, -16.566270321207174, 0]}}, {'type': 'Feature', 'properties': {'id': '8eea67f5-fef3-47be-ba31-fae2defc37a5', 'info': None, 'row': 143, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.03107588989934, -16.522611046419154, 0]}}, {'type': 'Feature', 'properties': {'id': '757dd631-d82d-40ce-8cfa-879fc0e8858a', 'info': None, 'row': 143, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.83969980451444, -16.4787779423684, 0]}}, {'type': 'Feature', 'properties': {'id': 'fcf2edea-44fc-4bf1-a81d-6349e1028656', 'info': None, 'row': 143, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.64840946202519, -16.434771566879867, 0]}}, {'type': 'Feature', 'properties': {'id': '890ef95f-2792-4a47-bf7f-2c567b916680', 'info': None, 'row': 143, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.45720495336539, -16.390592385934983, 0]}}, {'type': 'Feature', 'properties': {'id': '3749883c-ab2f-449a-a558-7b19fcea6a14', 'info': None, 'row': 143, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.2660863099178, -16.346241074245356, 0]}}, {'type': 'Feature', 'properties': {'id': '03003fdc-7f4a-4ab5-8cb6-4d9e4cc4ca91', 'info': None, 'row': 143, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.07505359317362, -16.30171814716307, 0]}}, {'type': 'Feature', 'properties': {'id': 'b1ea4d5b-8874-410a-9cb2-1360257925c7', 'info': None, 'row': 143, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.88410683655063, -16.257024211490595, 0]}}, {'type': 'Feature', 'properties': {'id': '3eb430a7-9be3-4c10-be68-ed6274864d0d', 'info': None, 'row': 143, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.69324610052348, -16.212159761086294, 0]}}, {'type': 'Feature', 'properties': {'id': '14b33ec1-60b1-40a2-a2f7-c6d4f5a13aa7', 'info': None, 'row': 143, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.5024714190461, -16.167125407717243, 0]}}, {'type': 'Feature', 'properties': {'id': '7225e55b-1e6b-4306-926c-22b9638ff4bd', 'info': None, 'row': 143, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.3117828234645, -16.121921772475968, 0]}}, {'type': 'Feature', 'properties': {'id': 'c3d397b5-d537-4401-a516-9816e00fef8f', 'info': None, 'row': 143, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.12118032999852, -16.07654940731194, 0]}}, {'type': 'Feature', 'properties': {'id': '4fe7ccb4-1d73-4425-9b22-525f1ac92d5b', 'info': None, 'row': 156, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.73072852440473, -17.025711418690964, 0]}}, {'type': 'Feature', 'properties': {'id': '44af0b59-4b37-494d-92c1-dbe9aa06a7f6', 'info': None, 'row': 156, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.53847169779783, -16.98344015389006, 0]}}, {'type': 'Feature', 'properties': {'id': 'e65f42d9-2971-402b-ad4c-13a99442c71c', 'info': None, 'row': 156, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.34630050148664, -16.94098925617628, 0]}}, {'type': 'Feature', 'properties': {'id': 'e3a192bc-d6c2-4552-b0ce-c8d05c331cec', 'info': None, 'row': 156, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.15421501544537, -16.898359212747664, 0]}}, {'type': 'Feature', 'properties': {'id': '7acfa7ba-1a12-4d01-af76-768199130f20', 'info': None, 'row': 156, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [78.96221535825421, -16.855550717670997, 0]}}, {'type': 'Feature', 'properties': {'id': '1f5d2758-d665-435a-9f3a-4724261ea6fd', 'info': None, 'row': 156, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.77030169679594, -16.81256422408176, 0]}}, {'type': 'Feature', 'properties': {'id': '4a221edd-acc8-427b-873e-14a50c388047', 'info': None, 'row': 156, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.57847413325871, -16.769400343181488, 0]}}, {'type': 'Feature', 'properties': {'id': '5f13e280-4762-4711-9ac1-cb69faddd3c1', 'info': None, 'row': 156, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.38673275605093, -16.726059654208665, 0]}}, {'type': 'Feature', 'properties': {'id': '462bbc9e-b118-4c39-815d-e9f539693fe3', 'info': None, 'row': 156, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.19507766385203, -16.68254265240741, 0]}}, {'type': 'Feature', 'properties': {'id': '5076402d-2b28-49d0-ae8d-006fbcaedf41', 'info': None, 'row': 156, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [78.00350893380346, -16.63884991417188, 0]}}, {'type': 'Feature', 'properties': {'id': '8de15a2d-ed87-4887-a35b-fd656869d69e', 'info': None, 'row': 156, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.81202663262363, -16.594982057213706, 0]}}, {'type': 'Feature', 'properties': {'id': '481b3523-e306-44cf-999e-664c8dbc54d2', 'info': None, 'row': 156, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.62063083701896, -16.550939647071893, 0]}}, {'type': 'Feature', 'properties': {'id': '8622d189-47f9-49f7-aff0-ef65d213ab7c', 'info': None, 'row': 156, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.42932164156643, -16.50672315125584, 0]}}, {'type': 'Feature', 'properties': {'id': '4268f202-15a6-498b-889e-6ce7d4cb03ac', 'info': None, 'row': 156, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.23809908216565, -16.462333242181693, 0]}}, {'type': 'Feature', 'properties': {'id': '82c93049-aba0-49dd-bbdc-6811e1fbe04d', 'info': None, 'row': 156, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.04696322198797, -16.41777044465632, 0]}}, {'type': 'Feature', 'properties': {'id': '7c3be8d0-270c-4119-9d54-e004d1c53c53', 'info': None, 'row': 156, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.85591410341709, -16.37303534516679, 0]}}, {'type': 'Feature', 'properties': {'id': 'b9f94f56-25c0-4997-acc2-05a535502cdf', 'info': None, 'row': 156, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.66495177154194, -16.328128515430862, 0]}}, {'type': 'Feature', 'properties': {'id': '8910ec27-295e-4489-a39f-79acc1ca6738', 'info': None, 'row': 156, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.47407628682882, -16.283050476230507, 0]}}, {'type': 'Feature', 'properties': {'id': '8d15a86a-98f1-4dfe-a6f2-532de6e6f2e3', 'info': None, 'row': 156, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.28328768157166, -16.237801860812567, 0]}}, {'type': 'Feature', 'properties': {'id': '752bcc86-ceae-4edc-898d-82fcfb3fb9ae', 'info': None, 'row': 156, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.0925859519726, -16.192383315837326, 0]}}, {'type': 'Feature', 'properties': {'id': '334ee4a9-72c3-4585-9f2a-cd2b5876160f', 'info': None, 'row': 169, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.70412594042295, -17.142201387995193, 0]}}, {'type': 'Feature', 'properties': {'id': 'e5ccc03e-bfee-45c4-a5b7-a6fb3ad51f9e', 'info': None, 'row': 169, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.51175535703925, -17.09990691727083, 0]}}, {'type': 'Feature', 'properties': {'id': 'a539b8c2-8de4-4505-8ee7-f6336499e1ba', 'info': None, 'row': 169, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.31947114386408, -17.05743151330161, 0]}}, {'type': 'Feature', 'properties': {'id': '5b680614-ca1f-42f4-b1ce-1c2b2727349a', 'info': None, 'row': 169, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.12727338152382, -17.01477566749075, 0]}}, {'type': 'Feature', 'properties': {'id': 'aca0cec9-eed4-4a42-a9ab-3f00ea3f58d8', 'info': None, 'row': 169, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [78.93516219145658, -16.97194007263723, 0]}}, {'type': 'Feature', 'properties': {'id': 'a17e1928-8544-4e50-a46c-3b51017c72b9', 'info': None, 'row': 169, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.7431377432454, -16.928925183797816, 0]}}, {'type': 'Feature', 'properties': {'id': '16e76987-74a2-4da9-a640-81ca2aca95a0', 'info': None, 'row': 169, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.55120014140304, -16.88573161670088, 0]}}, {'type': 'Feature', 'properties': {'id': '19a26701-e4e7-4cdb-8b46-60218c92415b', 'info': None, 'row': 169, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.35934947852387, -16.84235994814656, 0]}}, {'type': 'Feature', 'properties': {'id': 'a467cab1-c678-479b-817b-0ec8cf4bfcd7', 'info': None, 'row': 169, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.16758586079379, -16.798810657860184, 0]}}, {'type': 'Feature', 'properties': {'id': '72a35ae7-68a1-4142-a54e-df28728ab37b', 'info': None, 'row': 169, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [77.9759093534042, -16.75508438787979, 0]}}, {'type': 'Feature', 'properties': {'id': '85bda2ea-60e4-4249-ad36-c5941a80e460', 'info': None, 'row': 169, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.784320042739, -16.71118169046106, 0]}}, {'type': 'Feature', 'properties': {'id': 'f4847bd5-f860-4f5a-9910-c1295a9d655a', 'info': None, 'row': 169, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.59281800714363, -16.667103141082357, 0]}}, {'type': 'Feature', 'properties': {'id': '236e4ef0-69bb-4b38-8705-a448582b743d', 'info': None, 'row': 169, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.40140333175817, -16.622849262423095, 0]}}, {'type': 'Feature', 'properties': {'id': '8f371803-5e16-4c79-a7fe-b61af3565e03', 'info': None, 'row': 169, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.2100760695446, -16.578420673343263, 0]}}, {'type': 'Feature', 'properties': {'id': 'a8f141b3-fb5c-42a7-ae3a-2e1579b13d7d', 'info': None, 'row': 169, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [77.01883628327523, -16.53381791677079, 0]}}, {'type': 'Feature', 'properties': {'id': 'b1ea518c-1d3f-4045-9b29-50ed04232f89', 'info': None, 'row': 169, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.8276840214985, -16.489041570415843, 0]}}, {'type': 'Feature', 'properties': {'id': 'c5b7d43e-01fb-4e6c-9e7e-ab5d0776e1fd', 'info': None, 'row': 169, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.63661932632994, -16.444092234015773, 0]}}, {'type': 'Feature', 'properties': {'id': '2827b76d-b0e4-46ed-ab36-04318c075217', 'info': None, 'row': 169, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.44564225970845, -16.398970438055244, 0]}}, {'type': 'Feature', 'properties': {'id': 'dfaf0bf9-2569-4084-b80a-a0f1b6c2858c', 'info': None, 'row': 169, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.25475286920592, -16.35367677055937, 0]}}, {'type': 'Feature', 'properties': {'id': '7a1e4c1c-b3eb-4eeb-94a0-5284ee46c134', 'info': None, 'row': 169, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.06395114206596, -16.30821192907974, 0]}}, {'type': 'Feature', 'properties': {'id': '739d479e-b2d6-4b95-9450-602e26bd8ab8', 'info': None, 'row': 182, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.67750402815008, -17.258688562371145, 0]}}, {'type': 'Feature', 'properties': {'id': '1f022a60-48d7-4599-9051-d035b56b59e8', 'info': None, 'row': 182, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.4850190637966, -17.216370828055144, 0]}}, {'type': 'Feature', 'properties': {'id': '361473a7-e3ed-4ac7-8aef-e619cee4c9e2', 'info': None, 'row': 182, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.29262121260166, -17.173870854878345, 0]}}, {'type': 'Feature', 'properties': {'id': 'cec650c0-044f-4b12-aedc-4a09e73cc00e', 'info': None, 'row': 182, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.10031055276937, -17.13118915195324, 0]}}, {'type': 'Feature', 'properties': {'id': '825f6b44-c88c-461e-886e-16eddc00c34a', 'info': None, 'row': 182, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [78.90808721185026, -17.08832639683902, 0]}}, {'type': 'Feature', 'properties': {'id': 'e8bebca1-8628-4f60-806f-64cc184bf571', 'info': None, 'row': 182, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.7159513619764, -17.045283047276897, 0]}}, {'type': 'Feature', 'properties': {'id': 'e92fbd44-b987-4e79-9947-3640dad1089c', 'info': None, 'row': 182, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.52390310691553, -17.00205973657521, 0]}}, {'type': 'Feature', 'properties': {'id': '88177150-239d-4398-ab0f-346eca030d33', 'info': None, 'row': 182, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.33194254714793, -16.958657023551655, 0]}}, {'type': 'Feature', 'properties': {'id': '3ca5afca-a161-4e1a-8bcf-e88d8246263c', 'info': None, 'row': 182, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.14006979769225, -16.91507536711043, 0]}}, {'type': 'Feature', 'properties': {'id': 'e63f507a-fc0b-4c42-861e-6c331bd3f26a', 'info': None, 'row': 182, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [77.94828490294847, -16.871315511782548, 0]}}, {'type': 'Feature', 'properties': {'id': '1500420a-ace3-4b09-93d1-ad934517a3b8', 'info': None, 'row': 182, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.75658797792235, -16.82737790729384, 0]}}, {'type': 'Feature', 'properties': {'id': 'd7ae0733-1be2-4d25-ba16-5a2ad40ef2ee', 'info': None, 'row': 182, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.56497910235238, -16.783263140330636, 0]}}, {'type': 'Feature', 'properties': {'id': 'c3b14bfe-b01f-4087-b62e-1cb7e9b2e13d', 'info': None, 'row': 182, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.37345834299299, -16.738971825446225, 0]}}, {'type': 'Feature', 'properties': {'id': '457e4a85-997f-41ca-8034-e7a350682af4', 'info': None, 'row': 182, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.18202577846604, -16.694504492832046, 0]}}, {'type': 'Feature', 'properties': {'id': 'a6c11854-b5ec-4428-9dd4-81cee2755585', 'info': None, 'row': 182, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [76.99068146968192, -16.649861709636813, 0]}}, {'type': 'Feature', 'properties': {'id': 'b974a79f-2f94-47d4-afeb-61d024db7e73', 'info': None, 'row': 182, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.79942546933441, -16.60504405311332, 0]}}, {'type': 'Feature', 'properties': {'id': 'f4dda0f1-3063-4770-8950-6a9e4d6a4241', 'info': None, 'row': 182, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.60825782552027, -16.560052115067084, 0]}}, {'type': 'Feature', 'properties': {'id': '9d6dcc7b-8cb3-4afb-af0f-85a3d498c82d', 'info': None, 'row': 182, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.41717858400963, -16.514886506633843, 0]}}, {'type': 'Feature', 'properties': {'id': '4280ae3a-f7cf-4c91-a7de-cf0da54e1452', 'info': None, 'row': 182, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.22618781756826, -16.469547730927626, 0]}}, {'type': 'Feature', 'properties': {'id': '2d44555a-7c13-46f0-80d2-eadbe6b3d777', 'info': None, 'row': 182, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.03528551255268, -16.42403650441109, 0]}}, {'type': 'Feature', 'properties': {'id': '7484471b-f0e8-46f3-a4c5-670338030854', 'info': None, 'row': 195, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.65083911433858, -17.375169498708495, 0]}}, {'type': 'Feature', 'properties': {'id': '7048759e-c03f-49f2-b8c5-8d7aa7277fb8', 'info': None, 'row': 195, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.45823838944236, -17.332828373417918, 0]}}, {'type': 'Feature', 'properties': {'id': 'c6f5f0e1-9d38-4c55-ab0b-188b8e4368bd', 'info': None, 'row': 195, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.26572552278711, -17.29030371240244, 0]}}, {'type': 'Feature', 'properties': {'id': 'e1610328-78d0-4f05-9db5-4043b98ae480', 'info': None, 'row': 195, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.0733005978714, -17.24759600909214, 0]}}, {'type': 'Feature', 'properties': {'id': 'b23eaa69-fc95-4ac5-a6c8-0992505b6500', 'info': None, 'row': 195, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [78.88096374034066, -17.204705960454646, 0]}}, {'type': 'Feature', 'properties': {'id': '171e3f5c-9e84-4596-b2f7-cbdfa3357d1f', 'info': None, 'row': 195, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.68871512523614, -17.16163402539082, 0]}}, {'type': 'Feature', 'properties': {'id': '9bfb72b7-5fc0-43f6-be56-b83266d45e6e', 'info': None, 'row': 195, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.49655486261078, -17.11838082530769, 0]}}, {'type': 'Feature', 'properties': {'id': 'f5837038-68ab-411d-b7c3-49a160606d42', 'info': None, 'row': 195, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.3044830626003, -17.07494689423141, 0]}}, {'type': 'Feature', 'properties': {'id': 'e3dca132-f741-4623-bdd2-260741b4e494', 'info': None, 'row': 195, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.11249981666188, -17.03133280535702, 0]}}, {'type': 'Feature', 'properties': {'id': 'df44f55c-da27-4e1c-abf6-33d7b41502b8', 'info': None, 'row': 195, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [77.92060520068924, -16.98753918805516, 0]}}, {'type': 'Feature', 'properties': {'id': '608fe2bf-31ce-4573-a261-f2aeb4b456ca', 'info': None, 'row': 195, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.72879932378517, -16.943566533521015, 0]}}, {'type': 'Feature', 'properties': {'id': '647ea1f7-72d7-4502-b2d0-2de990ed3729', 'info': None, 'row': 195, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.53708227023264, -16.89941542646508, 0]}}, {'type': 'Feature', 'properties': {'id': '1e39f0c1-284b-478e-9671-08b00c904bd9', 'info': None, 'row': 195, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.34545410675939, -16.85508649819904, 0]}}, {'type': 'Feature', 'properties': {'id': 'ed3f3988-31b0-44ef-8a78-e49a1009e67f', 'info': None, 'row': 195, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.15391492061464, -16.81058026052296, 0]}}, {'type': 'Feature', 'properties': {'id': '2930a861-2a60-48f0-b290-3015543cf680', 'info': None, 'row': 195, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [76.96246477216253, -16.765897299405925, 0]}}, {'type': 'Feature', 'properties': {'id': '72268bee-cd5c-41d8-b923-7dccac476559', 'info': None, 'row': 195, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.77110371809654, -16.721038192304206, 0]}}, {'type': 'Feature', 'properties': {'id': 'b02345a6-66b7-44c6-83ae-02473d5dfdc7', 'info': None, 'row': 195, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.57983181340236, -16.676003519609978, 0]}}, {'type': 'Feature', 'properties': {'id': '44778beb-3240-4fd2-a396-18c534a3bbdb', 'info': None, 'row': 195, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.38864910260928, -16.63079391350585, 0]}}, {'type': 'Feature', 'properties': {'id': '3d04a302-37d6-4104-bb1e-4cc0fed01375', 'info': None, 'row': 195, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.19755566800077, -16.58540985548001, 0]}}, {'type': 'Feature', 'properties': {'id': '0ea4745d-4bcf-4bfd-9e01-34adb4d3bee4', 'info': None, 'row': 195, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [76.00655149953836, -16.539852063124417, 0]}}, {'type': 'Feature', 'properties': {'id': '36fc0d15-8274-4e1b-a014-dafc332e64cd', 'info': None, 'row': 208, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.62415334453513, -17.4916474239123, 0]}}, {'type': 'Feature', 'properties': {'id': 'cae675ec-78b1-4edc-b6d9-f10acc43656d', 'info': None, 'row': 208, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.43143619495945, -17.44928284569173, 0]}}, {'type': 'Feature', 'properties': {'id': '0e839765-bf05-47b7-a3a6-79867476f8a4', 'info': None, 'row': 208, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.23880765214426, -17.406733428712233, 0]}}, {'type': 'Feature', 'properties': {'id': '0c73645b-c000-433d-8d97-a5965cffb4fc', 'info': None, 'row': 208, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.04626780116836, -17.36399966674433, 0]}}, {'type': 'Feature', 'properties': {'id': '237b18e8-5cfb-438f-b031-51b9d0bf47de', 'info': None, 'row': 208, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [78.85381676964681, -17.3210822595709, 0]}}, {'type': 'Feature', 'properties': {'id': 'bdff401c-9f76-47a5-8473-4e1428375593', 'info': None, 'row': 208, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.6614547354293, -17.27798166785876, 0]}}, {'type': 'Feature', 'properties': {'id': 'abd2338d-970a-4da5-b04d-4e4d9edab118', 'info': None, 'row': 208, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.46918181209129, -17.234698512868892, 0]}}, {'type': 'Feature', 'properties': {'id': '75c06729-d1a7-42d2-96cc-fd03e19d8804', 'info': None, 'row': 208, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.27699811708193, -17.19123331363512, 0]}}, {'type': 'Feature', 'properties': {'id': 'ca5bb0a7-9fb8-46a3-91b5-fa37ada7385c', 'info': None, 'row': 208, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.08490373089343, -17.14758670513284, 0]}}, {'type': 'Feature', 'properties': {'id': '69093585-ffc5-44b9-90f9-0c9b7a918dff', 'info': None, 'row': 208, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [77.89289874781556, -17.10375925639681, 0]}}, {'type': 'Feature', 'properties': {'id': 'cf5f0610-e88d-4feb-aaca-166d5a64ee67', 'info': None, 'row': 208, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.70098327542607, -17.059751481909874, 0]}}, {'type': 'Feature', 'properties': {'id': 'cc14e2c3-1ca2-4973-bcc1-3d035ea475e1', 'info': None, 'row': 208, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.50915740164133, -17.015563968239555, 0]}}, {'type': 'Feature', 'properties': {'id': '240c1dad-b033-45c9-b39b-e2087de17b05', 'info': None, 'row': 208, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.317421196989, -16.9711973479637, 0]}}, {'type': 'Feature', 'properties': {'id': 'd0e8e8df-f5d8-4f7a-87ce-98b82b722583', 'info': None, 'row': 208, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.12577475573549, -16.926652121285535, 0]}}, {'type': 'Feature', 'properties': {'id': 'd81fdfa1-bc9c-40f2-a88d-b8613cc064b9', 'info': None, 'row': 208, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [76.9342181295836, -16.88192892573476, 0]}}, {'type': 'Feature', 'properties': {'id': '164931da-876a-4fad-a2c5-7d9402231455', 'info': None, 'row': 208, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.74275139168148, -16.83702828890316, 0]}}, {'type': 'Feature', 'properties': {'id': '4b892c30-2764-4495-98d9-c24150bc11be', 'info': None, 'row': 208, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.5513745967969, -16.79195080849913, 0]}}, {'type': 'Feature', 'properties': {'id': '835091e9-868e-497e-b72d-69f373bbcd3a', 'info': None, 'row': 208, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.36008779493636, -16.74669711108038, 0]}}, {'type': 'Feature', 'properties': {'id': '1e955116-7a7e-42e3-9b7e-353506e3e1fa', 'info': None, 'row': 208, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.1688910630922, -16.701267715343427, 0]}}, {'type': 'Feature', 'properties': {'id': '7c9b2538-30ba-42b9-a2c8-be4c3e88bd52', 'info': None, 'row': 208, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [75.97778440762976, -16.655663289363414, 0]}}, {'type': 'Feature', 'properties': {'id': 'eeedb480-eb63-4c2b-9cce-17fbc7caab87', 'info': None, 'row': 221, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.59744383731977, -17.608121921970692, 0]}}, {'type': 'Feature', 'properties': {'id': 'a85c82a5-f00f-468e-8a15-58fcbe31ff0a', 'info': None, 'row': 221, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.4046095071722, -17.565733820305088, 0]}}, {'type': 'Feature', 'properties': {'id': '88741aab-3dbc-4798-9056-0957d067cdbd', 'info': None, 'row': 221, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.21186453621662, -17.523159569824173, 0]}}, {'type': 'Feature', 'properties': {'id': '952c1bae-7272-449a-ba8b-eb7174058d47', 'info': None, 'row': 221, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [79.01920900688451, -17.480399682875472, 0]}}, {'type': 'Feature', 'properties': {'id': 'ab7860c6-fdd1-4901-a05f-1119743f1978', 'info': None, 'row': 221, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [78.82664305317084, -17.437454843171412, 0]}}, {'type': 'Feature', 'properties': {'id': '656a7965-2fc9-46e3-8798-0683e7038cca', 'info': None, 'row': 221, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.63416685561903, -17.394325513834893, 0]}}, {'type': 'Feature', 'properties': {'id': 'a78367c8-db3b-445f-bb5b-f3df1d38f59e', 'info': None, 'row': 221, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.44178052818268, -17.35101232936015, 0]}}, {'type': 'Feature', 'properties': {'id': 'c27b3984-3d11-49d3-972c-462087397d70', 'info': None, 'row': 221, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.24948419295863, -17.307515804941765, 0]}}, {'type': 'Feature', 'properties': {'id': 'dca68634-0c69-4f15-addc-bab1e58ff9ff', 'info': None, 'row': 221, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.05727793381122, -17.26383657757021, 0]}}, {'type': 'Feature', 'properties': {'id': '8cde8063-98c3-4dd1-b89f-d8c223cf4538', 'info': None, 'row': 221, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [77.86516184851887, -17.21997521834336, 0]}}, {'type': 'Feature', 'properties': {'id': 'a7634ab2-5fc8-4d9c-bfba-9182fbdf9bf9', 'info': None, 'row': 221, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.67313604811697, -17.175932244338018, 0]}}, {'type': 'Feature', 'properties': {'id': '41bd082e-0fa5-4e1e-8265-a4688987c30f', 'info': None, 'row': 221, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.48120062311851, -17.131708248341155, 0]}}, {'type': 'Feature', 'properties': {'id': '1879c318-3e80-40bf-a616-c0d41771c537', 'info': None, 'row': 221, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.28935565220635, -17.087303846564204, 0]}}, {'type': 'Feature', 'properties': {'id': '0b045a34-6647-4360-b399-b23ca0ee29db', 'info': None, 'row': 221, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.09760123483134, -17.042719535346766, 0]}}, {'type': 'Feature', 'properties': {'id': '364c2b65-0243-4fae-9992-eadcf5ea6a1a', 'info': None, 'row': 221, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [76.90593740479878, -16.997956041042748, 0]}}, {'type': 'Feature', 'properties': {'id': '104cb5e3-bdf6-4a9f-949b-0308ad5a855f', 'info': None, 'row': 221, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.71436426588708, -16.95301378439419, 0]}}, {'type': 'Feature', 'properties': {'id': '39b5a0a6-2f24-4051-aea8-9d391e3bbdeb', 'info': None, 'row': 221, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.52288186455755, -16.90789341312449, 0]}}, {'type': 'Feature', 'properties': {'id': '11931d1b-ff2f-454a-bb33-9e85ab06a041', 'info': None, 'row': 221, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.33149026394001, -16.862595517809737, 0]}}, {'type': 'Feature', 'properties': {'id': '0ff74c64-2ed5-4288-bbde-3f64f72a52ea', 'info': None, 'row': 221, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.14018951888096, -16.81712072131743, 0]}}, {'type': 'Feature', 'properties': {'id': 'cde91448-e451-474c-8501-4abc746363b9', 'info': None, 'row': 221, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [75.94897966671645, -16.771469584562542, 0]}}, {'type': 'Feature', 'properties': {'id': '8f69e3ee-d6c3-452f-93f1-b7faf46c05a9', 'info': None, 'row': 234, 'col': 0}, 'geometry': {'type': 'Point', 'coordinates': [79.57069646682201, -17.724590939336043, 0]}}, {'type': 'Feature', 'properties': {'id': '725acde0-8431-4426-a05e-7de372a66c33', 'info': None, 'row': 234, 'col': 21}, 'geometry': {'type': 'Point', 'coordinates': [79.37775680466375, -17.682182078864532, 0]}}, {'type': 'Feature', 'properties': {'id': 'fb755ac7-b3ba-4b2c-81ac-168cee1a65ed', 'info': None, 'row': 234, 'col': 42}, 'geometry': {'type': 'Point', 'coordinates': [79.18490514073575, -17.639585330061074, 0]}}, {'type': 'Feature', 'properties': {'id': '37561833-da5f-4c4c-8285-609c18a9876e', 'info': None, 'row': 234, 'col': 63}, 'geometry': {'type': 'Point', 'coordinates': [78.99214202380648, -17.596801326533438, 0]}}, {'type': 'Feature', 'properties': {'id': 'aef9d21a-cb81-4518-979c-3a3e65316a38', 'info': None, 'row': 234, 'col': 84}, 'geometry': {'type': 'Point', 'coordinates': [78.79946795607074, -17.553830716334613, 0]}}, {'type': 'Feature', 'properties': {'id': 'e281ffe1-19d4-4bd2-bbb3-c13179a904a6', 'info': None, 'row': 234, 'col': 105}, 'geometry': {'type': 'Point', 'coordinates': [78.60688334343558, -17.510674144766934, 0]}}, {'type': 'Feature', 'properties': {'id': '147a1b67-cc2b-47f4-b0aa-8cdd50a20013', 'info': None, 'row': 234, 'col': 126}, 'geometry': {'type': 'Point', 'coordinates': [78.41438850652243, -17.467332235897587, 0]}}, {'type': 'Feature', 'properties': {'id': '70c03b76-fd26-4766-b258-aa6a49b6a89b', 'info': None, 'row': 234, 'col': 147}, 'geometry': {'type': 'Point', 'coordinates': [78.22198370925068, -17.423805575197186, 0]}}, {'type': 'Feature', 'properties': {'id': 'd3820ba4-858f-417a-9534-3e15c7a44759', 'info': None, 'row': 234, 'col': 168}, 'geometry': {'type': 'Point', 'coordinates': [78.0296691721304, -17.380094752408663, 0]}}, {'type': 'Feature', 'properties': {'id': '498de3cd-633f-4f43-a1a4-7a72d307d16a', 'info': None, 'row': 234, 'col': 189}, 'geometry': {'type': 'Point', 'coordinates': [77.83744508708523, -17.336200365621906, 0]}}, {'type': 'Feature', 'properties': {'id': '23222d04-e03d-417a-a6f5-413ac1af0d74', 'info': None, 'row': 234, 'col': 210}, 'geometry': {'type': 'Point', 'coordinates': [77.64531162600505, -17.29212301820484, 0]}}, {'type': 'Feature', 'properties': {'id': 'e65aecbe-8403-4add-aa69-76288678a155', 'info': None, 'row': 234, 'col': 231}, 'geometry': {'type': 'Point', 'coordinates': [77.45326895291173, -17.247863277677503, 0]}}, {'type': 'Feature', 'properties': {'id': 'd2463ad7-fa62-4317-b628-72405ccee393', 'info': None, 'row': 234, 'col': 252}, 'geometry': {'type': 'Point', 'coordinates': [77.26131719518965, -17.203421790697163, 0]}}, {'type': 'Feature', 'properties': {'id': '0caa6f17-55dc-4065-a9c6-73e063ed55e3', 'info': None, 'row': 234, 'col': 273}, 'geometry': {'type': 'Point', 'coordinates': [77.06945649554143, -17.158799070228017, 0]}}, {'type': 'Feature', 'properties': {'id': '61e77ee4-7671-41da-961c-98fec2162db5', 'info': None, 'row': 234, 'col': 294}, 'geometry': {'type': 'Point', 'coordinates': [76.87768694660828, -17.113995767958645, 0]}}, {'type': 'Feature', 'properties': {'id': '385d37d6-5bc5-407f-b3df-aac244c887de', 'info': None, 'row': 234, 'col': 315}, 'geometry': {'type': 'Point', 'coordinates': [76.68600865848583, -17.069012418721776, 0]}}, {'type': 'Feature', 'properties': {'id': '17fc2188-77a7-4154-aa8e-5559f6a69582', 'info': None, 'row': 234, 'col': 336}, 'geometry': {'type': 'Point', 'coordinates': [76.49442171729717, -17.02384963126705, 0]}}, {'type': 'Feature', 'properties': {'id': 'b1efec25-d20c-4e90-8527-47ce84685b45', 'info': None, 'row': 234, 'col': 357}, 'geometry': {'type': 'Point', 'coordinates': [76.30292620137163, -16.97850803991793, 0]}}, {'type': 'Feature', 'properties': {'id': 'fd571ae9-c4cf-4405-845a-3304a7f20f08', 'info': None, 'row': 234, 'col': 378}, 'geometry': {'type': 'Point', 'coordinates': [76.11152221216145, -16.932988174768614, 0]}}, {'type': 'Feature', 'properties': {'id': 'a119da52-9df8-4a4c-80c3-439f4f556523', 'info': None, 'row': 234, 'col': 399}, 'geometry': {'type': 'Point', 'coordinates': [75.9202097789837, -16.887290709469696, 0]}}]}
array(0)
- windspeed_dual(line, sample)float64nan nan 1.5 8.7 ... 9.0 9.0 10.0
- comment :
- wind speed and direction inverted from model gmf_cmod5n (VV) and gmf_s1_v2 (VH)
- model :
- gmf_cmod5n gmf_s1_v2
array([[ nan, nan, 1.5, ..., 25.8, 8. , nan], [ nan, nan, 2.1, ..., 29.5, 9.2, nan], [ nan, nan, 2.3, ..., 29.9, 8.5, nan], ..., [ nan, nan, nan, ..., 15.9, 15.6, 9.7], [ nan, nan, nan, ..., 14.1, 13.8, 9.6], [ nan, nan, nan, ..., 9. , 9. , 10. ]])
- ancillary_wind_speed(line, sample)float6411.03 11.07 11.1 ... 13.71 13.73
array([[11.03221159, 11.06676038, 11.09654795, ..., 22.53373882, 22.30080115, 22.07195098], [11.04098691, 11.07523893, 11.10598657, ..., 22.71164426, 22.47633076, 22.2451646 ], [11.05045798, 11.08444979, 11.11552215, ..., 22.88343744, 22.64632154, 22.41308767], ..., [12.31185864, 12.33497141, 12.35776179, ..., 13.71181072, 13.73309649, 13.75383436], [12.29111344, 12.31658923, 12.34176566, ..., 13.70055281, 13.72165985, 13.74274344], [12.26564562, 12.29346108, 12.32085124, ..., 13.68389786, 13.7051064 , 13.72656094]])
- incidence(line, sample)float6419.47 19.55 19.63 ... 46.52 46.57
- history :
- incidenceAngle: annotation/s1a.xml: - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/line - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/pixel - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/incidenceAngle
array([[19.46749001, 19.54873141, 19.62997281, ..., 46.5925121 , 46.64257342, 46.69263473], [19.46677834, 19.54802009, 19.62926184, ..., 46.59201904, 46.6420809 , 46.69214276], [19.46606667, 19.54730877, 19.62855088, ..., 46.59152598, 46.64158838, 46.69165078], ..., [19.29354462, 19.37486493, 19.45618524, ..., 46.47129612, 46.52149003, 46.57168394], [19.29278772, 19.37410794, 19.45542815, ..., 46.47072697, 46.52092148, 46.57111599], [19.29203082, 19.37335095, 19.45467107, ..., 46.47015782, 46.52035293, 46.57054803]])
- windspeed_cross(line, sample)float64nan nan 3.0 3.0 ... 8.0 6.8 7.0 3.0
array([[ nan, nan, 3. , ..., 25.8, 7.4, nan], [ nan, nan, 3. , ..., 29.5, 9. , nan], [ nan, nan, 3. , ..., 30. , 8.1, nan], ..., [ nan, nan, nan, ..., 15.9, 15.7, 9.4], [ nan, nan, nan, ..., 14. , 13.8, 9.2], [ nan, nan, nan, ..., 6.8, 7. , 3. ]])
- sigma0_ocean_VH(line, sample)float64nan nan 1e-15 ... 0.0002512 1e-15
array([[ nan, nan, 1.00000000e-15, ..., 3.53468274e-03, 2.84606500e-04, nan], [ nan, nan, 1.00000000e-15, ..., 4.43905658e-03, 4.51932354e-04, nan], [ nan, nan, 1.00000000e-15, ..., 4.56320979e-03, 3.48507005e-04, nan], ..., [ nan, nan, nan, ..., 1.49645884e-03, 1.45414267e-03, 4.88714701e-04], [ nan, nan, nan, ..., 1.17451475e-03, 1.13126339e-03, 4.70211117e-04], [ nan, nan, nan, ..., 2.39005695e-04, 2.51202903e-04, 1.00000000e-15]])
- winddir_dual(line, sample)float64nan nan 46.87 ... 136.8 136.8 140.8
array([[ nan, nan, 46.86831665, ..., 255.91915894, 241.91928101, nan], [ nan, nan, 53.86778259, ..., 259.91864014, 240.91876221, nan], [ nan, nan, 42.86723328, ..., 257.9181366 , 239.91825867, nan], ..., [ nan, nan, nan, ..., 128.78886414, 128.78900146, 133.78913879], [ nan, nan, nan, ..., 129.7882843 , 129.78842163, 133.78855896], [ nan, nan, nan, ..., 136.78768921, 136.78782654, 140.78796387]])
- offboresight(line, sample)float6416.94 17.01 17.08 ... 40.3 40.35
array([[16.94033837, 17.01255947, 17.08478057, ..., 40.33062588, 40.3716654 , 40.41270493], [16.93985528, 17.01207672, 17.08429816, ..., 40.33035533, 40.37139539, 40.41243544], [16.93937219, 17.01159397, 17.08381575, ..., 40.33008479, 40.37112537, 40.41216595], ..., [16.82217867, 16.89447616, 16.96677365, ..., 40.26386365, 40.30503212, 40.34620059], [16.82166409, 16.89396153, 16.96625897, ..., 40.26353871, 40.30470777, 40.34587683], [16.82114952, 16.89344691, 16.96574429, ..., 40.26321376, 40.30438341, 40.34555306]])
- noise_lut(pol, line, sample)float321.898e+04 1.724e+04 ... 1.879e+03
- history :
- noise_lut: noise_lut_azi_raw_grd: annotation/calibration/noise.xml: - /noise/noiseAzimuthVectorList/noiseAzimuthVector/line - /noise/noiseAzimuthVectorList/noiseAzimuthVector/firstAzimuthLine - /noise/noiseAzimuthVectorList/noiseAzimuthVector/lastAzimuthLine - /noise/noiseAzimuthVectorList/noiseAzimuthVector/firstRangeSample - /noise/noiseAzimuthVectorList/noiseAzimuthVector/lastRangeSample - /noise/noiseAzimuthVectorList/noiseAzimuthVector/noiseAzimuthLut - /noise/noiseAzimuthVectorList/noiseAzimuthVector/swath noise_lut_range_raw: annotation/calibration/noise.xml: - /noise/noiseRangeVectorList/noiseRangeVector/line | /noise/noiseVectorList/noiseVector/line - /noise/noiseRangeVectorList/noiseRangeVector/pixel | /noise/noiseVectorList/noiseVector/pixel - /noise/noiseRangeVectorList/noiseRangeVector/noiseRangeLut | /noise/noiseVectorList/noiseVector/noiseLut - /noise/noiseRangeVectorList/noiseRangeVector/azimuthTime
array([[[18983.6 , 17237.143 , 15825.601 , ..., 1390.7626 , 994.4581 , 106.54908], [18461.334 , 16762.924 , 15390.216 , ..., 1378.9948 , 986.0436 , 105.64752], [18018.008 , 16360.383 , 15020.639 , ..., 1370.586 , 980.031 , 105.00332], ..., [ nan, nan, nan, ..., 1298.8496 , 1351.7589 , 1407.8301 ], [ nan, nan, nan, ..., 1321.6825 , 1375.4575 , 1432.449 ], [ nan, nan, nan, ..., 1351.4481 , 1406.4342 , 1464.7092 ]], [[26333.396 , 23830.414 , 21823.756 , ..., 1818.1952 , 1301.3331 , 139.42856], [25608.928 , 23174.805 , 21223.354 , ..., 1802.8107 , 1290.322 , 138.2488 ], [24993.96 , 22618.291 , 20713.701 , ..., 1791.8177 , 1282.4541 , 137.40579], ..., [ nan, nan, nan, ..., 1661.314 , 1732.0918 , 1807.1826 ], [ nan, nan, nan, ..., 1689.1057 , 1760.9763 , 1837.2379 ], [ nan, nan, nan, ..., 1727.1461 , 1800.6353 , 1878.6144 ]]], dtype=float32)
- noise_lut_azi(pol, line, sample)float321.128 1.128 1.128 ... 1.123 1.123
- history :
- noise_lut_azi_raw_grd: annotation/calibration/noise.xml: - /noise/noiseAzimuthVectorList/noiseAzimuthVector/line - /noise/noiseAzimuthVectorList/noiseAzimuthVector/firstAzimuthLine - /noise/noiseAzimuthVectorList/noiseAzimuthVector/lastAzimuthLine - /noise/noiseAzimuthVectorList/noiseAzimuthVector/firstRangeSample - /noise/noiseAzimuthVectorList/noiseAzimuthVector/lastRangeSample - /noise/noiseAzimuthVectorList/noiseAzimuthVector/noiseAzimuthLut - /noise/noiseAzimuthVectorList/noiseAzimuthVector/swath noise_lut_azi_raw_grd: annotation/calibration/noise.xml: - /noise/noiseAzimuthVectorList/noiseAzimuthVector/line - /noise/noiseAzimuthVectorList/noiseAzimuthVector/firstAzimuthLine - /noise/noiseAzimuthVectorList/noiseAzimuthVector/lastAzimuthLine - /noise/noiseAzimuthVectorList/noiseAzimuthVector/firstRangeSample - /noise/noiseAzimuthVectorList/noiseAzimuthVector/lastRangeSample - /noise/noiseAzimuthVectorList/noiseAzimuthVector/noiseAzimuthLut - /noise/noiseAzimuthVectorList/noiseAzimuthVector/swath
array([[[1.1282482, 1.1282482, 1.1282482, ..., 1.0196892, 1.0196892, 1.0196892], [1.0972085, 1.0972085, 1.0972085, ..., 1.0110612, 1.0110612, 1.0110612], [1.0708604, 1.0708604, 1.0708604, ..., 1.004896 , 1.004896 , 1.004896 ], ..., [ nan, nan, nan, ..., 1.076504 , 1.076504 , 1.076504 ], [ nan, nan, nan, ..., 1.0980896, 1.0980896, 1.0980896], [ nan, nan, nan, ..., 1.1228197, 1.1228197, 1.1228197]], [[1.1282482, 1.1282482, 1.1282482, ..., 1.0196892, 1.0196892, 1.0196892], [1.0972085, 1.0972085, 1.0972085, ..., 1.0110612, 1.0110612, 1.0110612], [1.0708604, 1.0708604, 1.0708604, ..., 1.004896 , 1.004896 , 1.004896 ], ..., [ nan, nan, nan, ..., 1.076504 , 1.076504 , 1.076504 ], [ nan, nan, nan, ..., 1.0980896, 1.0980896, 1.0980896], [ nan, nan, nan, ..., 1.1228197, 1.1228197, 1.1228197]]], dtype=float32)
- lineSpacing()float641e+03
- units :
- m
array(1000.)
- longitude(line, sample)float6480.05 80.04 80.03 ... 75.76 75.75
- history :
- longitude: annotation/s1a.xml: - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/line - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/pixel - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/longitude
array([[80.04782947, 80.03873596, 80.02964244, ..., 76.29742492, 76.28840107, 76.27937723], [80.0458075 , 80.0367136 , 80.02761971, ..., 76.29525662, 76.28623245, 76.27720828], [80.04378552, 80.03469125, 80.02559697, ..., 76.29308833, 76.28406383, 76.27503934], ..., [79.56452314, 79.55533427, 79.54614541, ..., 75.77695917, 75.76785169, 75.75874421], [79.56246537, 79.55327612, 79.54408687, ..., 75.77474076, 75.76563291, 75.75652506], [79.56040759, 79.55121796, 79.54202833, ..., 75.77252236, 75.76341413, 75.7543059 ]])
- land_mask(line, sample)int80 0 0 0 0 0 0 0 ... 0 0 0 0 0 0 0 0
- history :
- land_mask: cartopy.feature.NaturalEarthFeature land
- meaning :
- 0: ocean , 1: land
array([[0, 0, 0, ..., 0, 0, 0], [0, 0, 0, ..., 0, 0, 0], [0, 0, 0, ..., 0, 0, 0], ..., [0, 0, 0, ..., 0, 0, 0], [0, 0, 0, ..., 0, 0, 0], [0, 0, 0, ..., 0, 0, 0]], dtype=int8)
- nesz_VH_final(line, sample)float640.003807 0.003782 ... 0.0004186
- comment :
- nesz has been flattened using windspeed.nesz_flattening
array([[0.00380749, 0.00378172, 0.00375613, ..., 0.0003941 , 0.00039245, 0.00039081], [0.0037503 , 0.00372522, 0.00370031, ..., 0.00039881, 0.00039716, 0.00039552], [0.00369819, 0.00367377, 0.00364951, ..., 0.00040431, 0.00040266, 0.00040102], ..., [0.00352878, 0.00350622, 0.0034838 , ..., 0.00041417, 0.00041254, 0.00041091], [0.00351429, 0.00349196, 0.00346976, ..., 0.00041758, 0.00041594, 0.00041431], [0.00349632, 0.00347425, 0.00345232, ..., 0.00042186, 0.00042021, 0.00041858]])
- sigma0_lut(pol, line, sample)float641.874e+03 1.87e+03 ... 1.274e+03
- history :
- sigma0_lut: annotation/calibration/calibration.xml: //calibration/calibrationVectorList/calibrationVector/sigmaNought
array([[[1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676], [1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676], [1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676], ..., [1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676], [1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676], [1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676]], [[1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676], [1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676], [1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676], ..., [1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676], [1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676], [1873.755 , 1870.02375 , 1866.3299 , ..., 1275.52535 , 1275.004725 , 1274.47875676]]])
- noise_lut_range(pol, line, sample)float321.683e+04 1.528e+04 ... 1.673e+03
- history :
- noise_lut_range_raw: annotation/calibration/noise.xml: - /noise/noiseRangeVectorList/noiseRangeVector/line | /noise/noiseVectorList/noiseVector/line - /noise/noiseRangeVectorList/noiseRangeVector/pixel | /noise/noiseVectorList/noiseVector/pixel - /noise/noiseRangeVectorList/noiseRangeVector/noiseRangeLut | /noise/noiseVectorList/noiseVector/noiseLut - /noise/noiseRangeVectorList/noiseRangeVector/azimuthTime noise_lut_range_raw: annotation/calibration/noise.xml: - /noise/noiseRangeVectorList/noiseRangeVector/line | /noise/noiseVectorList/noiseVector/line - /noise/noiseRangeVectorList/noiseRangeVector/pixel | /noise/noiseVectorList/noiseVector/pixel - /noise/noiseRangeVectorList/noiseRangeVector/noiseRangeLut | /noise/noiseVectorList/noiseVector/noiseLut - /noise/noiseRangeVectorList/noiseRangeVector/azimuthTime noise_lut_range_raw: annotation/calibration/noise.xml: - /noise/noiseRangeVectorList/noiseRangeVector/line | /noise/noiseVectorList/noiseVector/line - /noise/noiseRangeVectorList/noiseRangeVector/pixel | /noise/noiseVectorList/noiseVector/pixel - /noise/noiseRangeVectorList/noiseRangeVector/noiseRangeLut | /noise/noiseVectorList/noiseVector/noiseLut - /noise/noiseRangeVectorList/noiseRangeVector/azimuthTime noise_lut_range_raw: annotation/calibration/noise.xml: - /noise/noiseRangeVectorList/noiseRangeVector/line | /noise/noiseVectorList/noiseVector/line - /noise/noiseRangeVectorList/noiseRangeVector/pixel | /noise/noiseVectorList/noiseVector/pixel - /noise/noiseRangeVectorList/noiseRangeVector/noiseRangeLut | /noise/noiseVectorList/noiseVector/noiseLut - /noise/noiseRangeVectorList/noiseRangeVector/azimuthTime
array([[[16825.73 , 15277.793 , 14026.701 , ..., 1363.9083 , 975.2561 , 104.49172], [16825.73 , 15277.793 , 14026.701 , ..., 1363.9083 , 975.2561 , 104.49172], [16825.73 , 15277.793 , 14026.701 , ..., 1363.9083 , 975.2561 , 104.49172], ..., [ 0. , 0. , 0. , ..., 1206.5442 , 1255.6934 , 1307.7797 ], [ 0. , 0. , 0. , ..., 1203.6199 , 1252.5913 , 1304.492 ], [ 0. , 0. , 0. , ..., 1203.6199 , 1252.5913 , 1304.492 ]], [[23340.074 , 21121.605 , 19343.045 , ..., 1783.0876 , 1276.2057 , 136.73633], [23340.074 , 21121.605 , 19343.045 , ..., 1783.0876 , 1276.2057 , 136.73633], [23340.074 , 21121.605 , 19343.045 , ..., 1783.0876 , 1276.2057 , 136.73633], ..., [ 0. , 0. , 0. , ..., 1543.2493 , 1608.9971 , 1678.7515 ], [ 0. , 0. , 0. , ..., 1538.2222 , 1603.6727 , 1673.1221 ], [ 0. , 0. , 0. , ..., 1538.2222 , 1603.6727 , 1673.1221 ]]], dtype=float32)
- sigma0_ocean_VV(line, sample)float64nan nan 0.1527 ... 0.02757 0.01905
array([[ nan, nan, 0.15266891, ..., 0.09798013, 0.01887847, nan], [ nan, nan, 0.2039838 , ..., 0.11599328, 0.02207009, nan], [ nan, nan, 0.2124894 , ..., 0.10965535, 0.02090809, nan], ..., [ nan, nan, nan, ..., 0.05232882, 0.05342966, 0.03342258], [ nan, nan, nan, ..., 0.05016846, 0.04567412, 0.03168606], [ nan, nan, nan, ..., 0.02705501, 0.02756985, 0.01904535]])
- windspeed_co(line, sample)float64nan nan 1.5 8.7 ... 11.4 11.5 10.0
- comment :
- wind speed and direction inverted from model gmf_cmod5n (VV)
- model :
- gmf_cmod5n
array([[ nan, nan, 1.5, ..., 21. , 9.5, nan], [ nan, nan, 2.1, ..., 24.3, 10.3, nan], [ nan, nan, 2.3, ..., 23.1, 10.2, nan], ..., [ nan, nan, nan, ..., 15.1, 15.3, 12.4], [ nan, nan, nan, ..., 14.9, 14.1, 12.1], [ nan, nan, nan, ..., 11.4, 11.5, 10. ]])
- latitude(line, sample)float64-15.63 -15.63 ... -16.9 -16.89
- history :
- latitude: annotation/s1a.xml: - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/line - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/pixel - /product/geolocationGrid/geolocationGridPointList/geolocationGridPoint/latitude
array([[-15.62752284, -15.6255231 , -15.62352336, ..., -14.76984842, -14.76770538, -14.76556233], [-15.63648714, -15.63448732, -15.6324875 , ..., -14.77876088, -14.77661767, -14.77447446], [-15.64545144, -15.64345155, -15.64145165, ..., -14.78767335, -14.78552997, -14.78338659], ..., [-17.75146828, -17.74944884, -17.7474294 , ..., -16.88126118, -16.87907733, -16.87689347], [-17.76042739, -17.75840788, -17.75638837, ..., -16.890168 , -16.88798398, -16.88579995], [-17.76938651, -17.76736692, -17.76534733, ..., -16.89907483, -16.89689063, -16.89470642]])
- winddir_co(line, sample)float64nan nan 46.87 ... 136.8 136.8 140.8
array([[ nan, nan, 46.86831665, ..., 255.91915894, 241.91928101, nan], [ nan, nan, 53.86778259, ..., 259.91864014, 240.91876221, nan], [ nan, nan, 42.86723328, ..., 257.9181366 , 239.91825867, nan], ..., [ nan, nan, nan, ..., 128.78886414, 128.78900146, 133.78913879], [ nan, nan, nan, ..., 129.7882843 , 129.78842163, 133.78855896], [ nan, nan, nan, ..., 136.78768921, 136.78782654, 140.78796387]])
- gamma0_lut(pol, line, sample)float641.818e+03 1.814e+03 ... 1.056e+03
- history :
- gamma0_lut: annotation/calibration/calibration.xml: //calibration/calibrationVectorList/calibrationVector/gamma
array([[[1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649], [1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649], [1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649], ..., [1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649], [1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649], [1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649]], [[1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649], [1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649], [1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649], ..., [1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649], [1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649], [1818.2852 , 1814.2002 , 1810.15315 , ..., 1057.56385 , 1056.649475 , 1055.72448649]]])
- sampleSpacing()float641e+03
- units :
- m
- referential :
- ground
array(1000.)
- ground_heading(line, sample)float32-167.1 -167.1 ... -167.2 -167.2
- comment :
- at ground level, computed from lon/lat in azimuth direction
- long_name :
- Platform heading (azimuth from North)
- units :
- Degrees
array([[-167.13193, -167.1318 , -167.13168, ..., -167.08084, -167.08072, -167.0806 ], [-167.13248, -167.13235, -167.13222, ..., -167.08136, -167.08124, -167.08112], [-167.13303, -167.13289, -167.13277, ..., -167.08186, -167.08174, -167.08163], ..., [-167.26921, -167.26906, -167.26892, ..., -167.21114, -167.211 , -167.21086], [-167.26982, -167.26968, -167.26953, ..., -167.21172, -167.21158, -167.21144], [-167.27043, -167.2703 , -167.27014, ..., -167.21231, -167.21217, -167.21204]], dtype=float32)
- ancillary_wind_direction(line, sample)float6455.97 56.17 56.36 ... 131.1 131.2
array([[ 55.96836609, 56.16915338, 56.35930245, ..., 258.45925651, 258.12102459, 257.77217093], [ 55.90880531, 56.11472504, 56.313968 , ..., 257.86775852, 257.53356687, 257.19337051], [ 55.84424924, 56.05540713, 56.26161556, ..., 257.26440989, 256.93289566, 256.60069556], ..., [ 72.3940811 , 72.45782978, 72.51830959, ..., 130.95530917, 131.06999307, 131.18984923], [ 72.46701538, 72.53115303, 72.59244777, ..., 130.95215729, 131.06738616, 131.18559797], [ 72.54508604, 72.60835719, 72.67006442, ..., 130.95060956, 131.06307132, 131.17845917]])
- polPandasIndex
PandasIndex(Index(['VV', 'VH'], dtype='object', name='pol'))
- linePandasIndex
PandasIndex(Float64Index([ 12.0, 37.0, 62.0, 87.0, 112.0, 137.0, 162.0, 187.0, 212.0, 237.0, ... 5762.0, 5787.0, 5812.0, 5837.0, 5862.0, 5887.0, 5912.0, 5937.0, 5962.0, 5987.0], dtype='float64', name='line', length=240))
- samplePandasIndex
PandasIndex(Float64Index([ 12.0, 37.0, 62.0, 87.0, 112.0, 137.0, 162.0, 187.0, 212.0, 237.0, ... 10187.0, 10212.0, 10237.0, 10262.0, 10287.0, 10312.0, 10337.0, 10362.0, 10387.0, 10412.0], dtype='float64', name='sample', length=417))
- name :
- SENTINEL1_DS:/tmp/S1A_EW_GRDM_1SDV_20230217T002336_20230217T002412_047268_05AC30_Z005.SAFE:EW
- short_name :
- SENTINEL1_DS:S1A_EW_GRDM_1SDV_20230217T002336_20230217T002412_047268_05AC30_Z005.SAFE:EW
- product :
- GRDM
- safe :
- S1A_EW_GRDM_1SDV_20230217T002336_20230217T002412_047268_05AC30_Z005.SAFE
- swath :
- EW
- multidataset :
- False
- ipf :
- 3.52
- platform :
- SENTINEL-1A
- pols :
- VV VH
- start_date :
- 2023-02-17 00:23:36.783295
- stop_date :
- 2023-02-17 00:24:12.397167
- footprint :
- POLYGON ((80.05316482421334 -15.62417982506369, 76.27139463973474 -14.75914155166145, 75.74395474319797 -16.89750975171368, 79.56366636012008 -17.77537303564744, 80.05316482421334 -15.62417982506369))
- coverage :
- 244km * 417km (line * sample )
- orbit_pass :
- Descending
- platform_heading :
- -167.8308256427968
[28]:
#ds_1000.to_netcdf("my_L2_product.nc")